How to use array_merge function to merge arrays in PHP
In PHP, array operations are a very frequent operation. When we need to use values from multiple arrays at the same time, we need to merge these arrays into one array for easy operation. PHP provides the array_merge function to merge two or more arrays into one array. In this article, we will explain how to merge arrays using the array_merge function.
The syntax of the array_merge function is as follows:
array array_merge ( array $array1 [, array $... ] )
Among them, array1
must be an array, ...
represents optional parameters, and you can pass multiple array. This function combines the arrays in all arguments into a new array and returns that array.
For example, the following code merges two arrays and saves the result in a new array:
$array1 = array('apple', 'banana', 'orange'); $array2 = array('watermelon', 'pear', 'pineapple'); $result = array_merge($array1, $array2); print_r($result);
The output result is:
Array ( [0] => apple [1] => banana [2] => orange [3] => watermelon [4] => pear [5] => pineapple )
From the output result, you can It can be seen that the array_merge()
function merges the two arrays into a new array and re-indexes the array in increasing order.
In addition to the default behavior used in the above example, the array_merge()
function has some other parameter settings:
- Keep key name
By default, the array_merge()
function will re-index the numeric key names in the array. If you want to retain the key names of the array, you can set the first parameter of the array_merge()
function (array1
) to an associative array.
For example, the following code will retain the key names and merge two associative arrays:
$array1 = array('a' => 'apple', 'b' => 'banana'); $array2 = array('c' => 'carrot', 'd' => 'date'); $result = array_merge($array1, $array2); print_r($result);
The output result is:
Array ( [a] => apple [b] => banana [c] => carrot [d] => date )
As you can see, array_merge( )
The function merges two associative arrays into one associative array and retains the original key names.
- Merge numeric key names
array_merge()
The function only merges the values of numeric key names by default. If you need to merge the values of associative arrays, you can Use the
operator to merge.
For example, the following code merges associative arrays and numeric arrays:
$array1 = array('a' => 'apple', 'b' => 'banana'); $array2 = array('c' => 'carrot', 'd' => 'date'); $result = $array1 + $array2; print_r($result);
The output result is:
Array ( [a] => apple [b] => banana [c] => carrot [d] => date )
As you can see, $array1 $array2
Implemented the operation of merging two arrays into an associative array and retaining key names.
Summary: array_merge()
The function is a very commonly used function in PHP, which can merge two or more arrays and return a new array. When merging arrays, you can retain the original key names and use the
operator to merge associative arrays. Mastering the usage of the array_merge()
function is very important for PHP array operations.
The above is the detailed content of How to use array_merge function to merge arrays in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
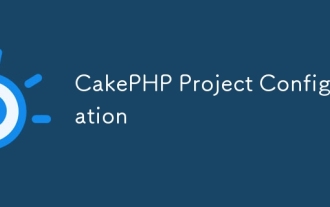
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
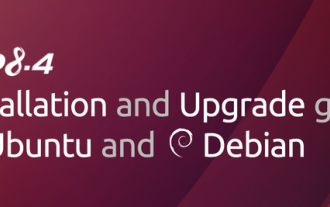
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
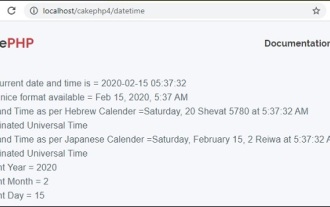
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
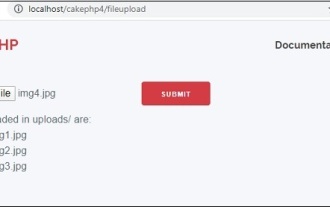
To work on file upload we are going to use the form helper. Here, is an example for file upload.
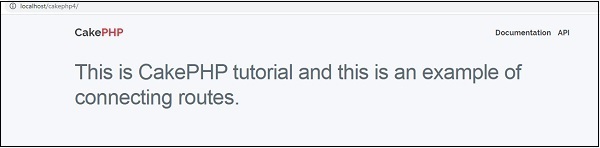
In this chapter, we are going to learn the following topics related to routing ?
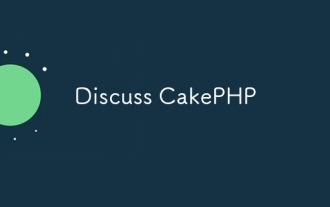
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
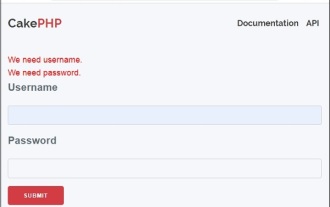
Validator can be created by adding the following two lines in the controller.
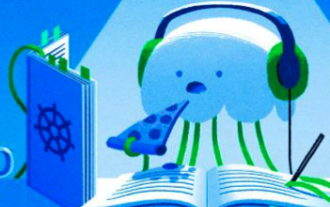
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
