How to parse URL using parse_url function in PHP
URL is a basic concept in Web development. It refers to the Uniform Resource Locator, which is the address used to locate resources on the Web. In PHP, there is a built-in function parse_url that can be used to parse URLs. This article will introduce how to use this function.
1. Basic concept of parse_url function
The parse_url function in PHP is a function used to parse a URL and return the various components of the URL. The URL types supported by this function include: http, ftp, gopher, file, etc. Almost any URL can be parsed using this function, and returns an array containing the following key-value pairs:
scheme: The protocol part of the URL
host: The hostname part of the URL
port : The port part of the URL
user: The username part of the URL
pass: The password part of the URL
path: The path part of the URL
query: The query string part of the URL
fragment: URL The anchor part
2. How to use the parse_url function
The following is a sample code for using the parse_url function to parse the URL:
$url = "https://www.example.com/index.php?key1=value1&key2=value2#section1"; $arr = parse_url($url); print_r($arr);
The output result is:
Array ( [scheme] => https [host] => www.example.com [path] => /index.php [query] => key1=value1&key2=value2 [fragment] => section1 )
Yes It can be seen that the parse_url function extracts the protocol, host, path, query string and anchor point from the URL, and returns them as an array.
3. Parse the query string part
In actual development, we often need to parse the query string part in the URL, because the query string usually contains the parameters and values passed to the Web server. The parse_str function in PHP can be used to parse the query string into an associative array. The following is a sample code:
$str = "key1=value1&key2=value2"; parse_str($str, $arr); print_r($arr);
The output result is:
Array ( [key1] => value1 [key2] => value2 )
The parse_str function parses the query string into an associative array, where each key corresponds to a parameter name in the query string. , each value corresponds to the value corresponding to the parameter name.
4. Parse multiple URIs
In actual development, we sometimes need to parse multiple URLs at the same time. At this time, we can use the array_map function in PHP. The function of the array_map function is to apply the callback function to each element in the given array and return a new array containing the results of the callback function execution. The following is a sample code:
$urls = [ "https://www.example.com/index.php?key1=value1&key2=value2#section1", "https://www.example.com/about-us.php?key1=value3&key2=value4#section2" ]; $arrs = array_map('parse_url', $urls); print_r($arrs);
The output result is:
Array ( [0] => Array ( [scheme] => https [host] => www.example.com [path] => /index.php [query] => key1=value1&key2=value2 [fragment] => section1 ) [1] => Array ( [scheme] => https [host] => www.example.com [path] => /about-us.php [query] => key1=value3&key2=value4 [fragment] => section2 ) )
It can be seen that the array_map function passes each element in the array as a parameter to the parse_url function and executes the parse_url function The results are added to a new array and returned.
5. Summary
This article introduces how to use the parse_url function in PHP to parse URLs. First, we learned about the return value of the parse_url function, second, we introduced how to use the parse_str function to parse the query string part, and finally we also introduced how to parse multiple URLs at the same time. Knowing these basic usage methods is enough to meet our needs for parsing URLs in actual development.
The above is the detailed content of How to parse URL using parse_url function in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
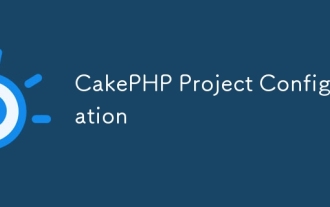
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
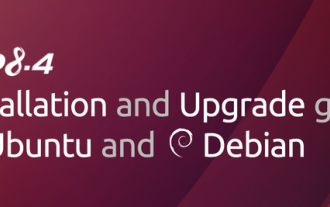
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
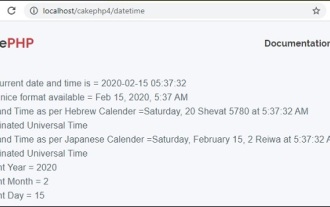
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
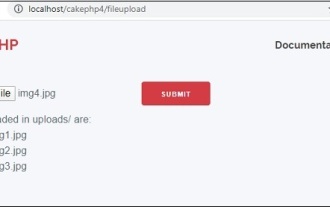
To work on file upload we are going to use the form helper. Here, is an example for file upload.
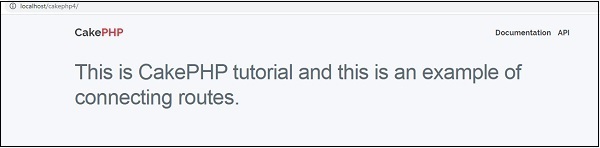
In this chapter, we are going to learn the following topics related to routing ?
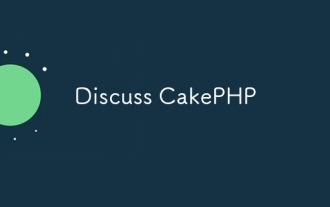
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
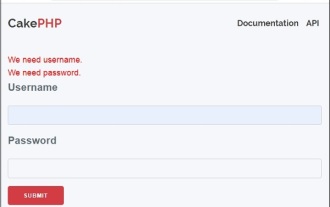
Validator can be created by adding the following two lines in the controller.
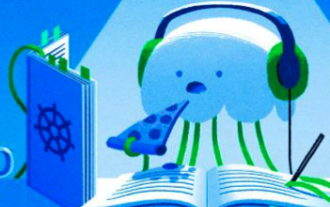
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
