


Develop an efficient RESTful API service using the PHP framework Fat-Free
RESTful API is a standard Web service architecture based on the HTTP protocol. It is the most popular technology in the development of various Web applications on the Internet today. RESTful APIs can be used to quickly provide many different data and functions to external applications or other services. In this article, we will introduce Fat-Free, an efficient PHP framework, and how to use it to develop a RESTful API service.
1. What is the Fat-Free framework?
Fat-Free is a lightweight, flexible open source PHP framework. Its name also implies its characteristics: fast, Simple and compact. The framework provides many built-in basic functional modules, such as routing, template engine, database, etc., making it very efficient, simple and flexible when creating web applications.
2. Why use the Fat-Free framework?
- Very lightweight: The Fat-Free framework has a very small capacity and takes up very little space, so it can be loaded quickly and can Easily deployed on various servers.
- Powerful routing system: The routing system of the Fat-Free framework clearly embodies the idea of the framework – providing developers with fast and accurate URL path mapping, and providing many functions that help developers work. , such as: route protection, route constraints, route aliases, etc.
- Flexible ORM: The Fat-Free framework provides a very flexible ORM model, supports multiple databases, supports various relationships and query methods, and is a complete database operation framework.
- Powerful plug-in mechanism: The Fat-Free framework provides many powerful plug-ins to support various functions, including email, image processing, security, printing debugging, etc.
3. How to use the Fat-Free framework to develop RESTful API services?
- Install the Fat-Free framework
You can start from Fat- Download the installation package from Free's official website, or you can use composer to install it.
- Create a RESTful API folder
Create a new API folder in your Web directory and move the Fat-Free framework to the API folder, as shown in the figure Shown:
├─API/ │ ├─f3/ │ │ ├─lib/ │ │ ├─... │ ├─index.php
- Create the API entry file index.php
Create a file index.php, which is the entry file for our API service. We need to include the Fat-Free framework.
<?php $f3 = require('f3/lib/base.php'); // RESTful API 路由 $f3->route('GET /api/@apiname','api@get'); $f3->route('POST /api/@apiname','api@post'); $f3->route('PUT /api/@apiname','api@put'); $f3->route('DELETE /api/@apiname','api@delete'); // 连接数据库 $f3->set('DB', new DBSQL('mysql:host=localhost;port=3306;dbname=test', 'root', 'root')); // 执行 $f3->run();
In this file, we define four routes, corresponding to the four request methods of the HTTP protocol, namely GET, POST, PUT, and DELETE. The Fat-Free framework supports processing requests through routing, which defines the mapping relationship between URL paths and functions. Therefore, we define a controller called api and map four different request methods to it.
- Create API Controller
We need an API controller to handle requests initiated by the client and return corresponding response data.
<?php class api { protected $APIVer = 'v1'; private function respond($response) { header('Content-type: application/json; charset=utf-8'); header('Cache-control: max-age=3600'); echo json_encode($response, JSON_PRETTY_PRINT|JSON_UNESCAPED_UNICODE); } public function get($f3) { $request = new WebREST($f3->get('VERB'), @$f3->get('PARAMS.apiname'), @$f3->get('PARAMS.id')); $result = $request->process(); if ($result) { $this->respond($result); $f3->status(200); } else $f3->status(404); } public function post($f3) { $request = new WebREST($f3->get('VERB'), @$f3->get('PARAMS.apiname'), @$f3->get('PARAMS.id')); $result = $request->process(); if ($result) { $this->respond($result); $f3->status(201); } else $f3->status(404); } public function put($f3) { $request = new WebREST($f3->get('VERB'), @$f3->get('PARAMS.apiname'), @$f3->get('PARAMS.id')); $result = $request->process(); if ($result) { $this->respond($result); $f3->status(202); } else $f3->status(404); } public function delete($f3) { $request = new WebREST($f3->get('VERB'), @$f3->get('PARAMS.apiname'), @$f3->get('PARAMS.id')); $result = $request->process(); if ($result) { $this->respond($result); $f3->status(202); } else $f3->status(404); } }
In this controller, four methods are defined: get, post, put, and delete. In these methods, we need to instantiate a Web REST object and call its process method to get the response data. From the perspective of HTTP response, the response data should be in JSON format, so in the respond method, we use PHP's json_encode method to convert the response data into a JSON string and output it to the client.
- Create the Web/REST.php class file
This class file is used to handle requests from the RESTful API server.
<?php namespace Web; class REST { private $verb; // HTTP 请求方法 private $apiname; // API名称 private $id; // API 记录id private $user; // 用户认证信息 protected $db; // 数据库连接 protected $base; // 数据库基本名称 protected $table; // 表名 protected $data; // 用于 POST 和 PUT 请求中的数据 protected $fields = array(); // 表字段名称 protected $response_code = array( 100 => 'Continue', 101 => 'Switching Protocols', 200 => 'OK', 201 => 'Created', 202 => 'Accepted', 203 => 'Non-Authoritative Information', 204 => 'No Content', 205 => 'Reset Content', 206 => 'Partial Content', 300 => 'Multiple Choices', 301 => 'Moved Permanently', 302 => 'Found', 303 => 'See Other', 304 => 'Not Modified', 305 => 'Use Proxy', 307 => 'Temporary Redirect', 400 => 'Bad Request', 401 => 'Unauthorized', 402 => 'Payment Required', 403 => 'Forbidden', 404 => 'Not Found', 405 => 'Method Not Allowed', 406 => 'Not Acceptable', 407 => 'Proxy Authentication Required', 408 => 'Request Timeout', 409 => 'Conflict', 410 => 'Gone', 411 => 'Length Required', 412 => 'Precondition Failed', 413 => 'Request Entity Too Large', 414 => 'Request-URI Too Long', 415 => 'Unsupported Media Type', 416 => 'Requested Range Not Satisfiable', 417 => 'Expectation Failed', 500 => 'Internal Server Error', 501 => 'Not Implemented', 502 => 'Bad Gateway', 503 => 'Service Unavailable', 504 => 'Gateway Timeout', 505 => 'HTTP Version Not Supported' ); public function __construct($verb, $apiname, $id = null, $data = null) { $this->verb = $verb; $this->apiname = $apiname; $this->id = $id; $this->data = $data; $this->db = Base::instance()->get('DB'); } public function process() { //$sql = "SELECT..."; ... } } }
In this class file, we implement a REST class that handles requests from the RESTful API server. The class contains the HTTP request method type, API name, API record ID, data to be processed, etc. This class operates the database, obtains relevant data, creates requests and returns response data.
4. Conclusion
As we have seen before, it is very easy to develop RESTful API services using the PHP framework Fat-Free, because it is a lightweight framework itself, and Its powerful routing mechanism means that we can define API routes very flexibly. In addition, it provides many very useful modules to help us complete web application development quickly. This is the main reason why we choose Fat-Free as the PHP framework. It is its lightweight, efficient, reliable and flexible characteristics that enable us to quickly create exquisite RESTful APIs.
The above is the detailed content of Develop an efficient RESTful API service using the PHP framework Fat-Free. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


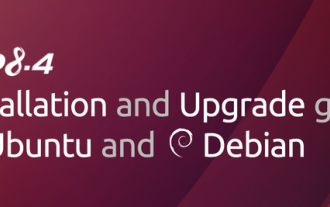
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
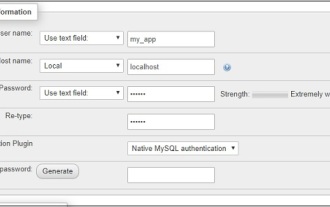
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
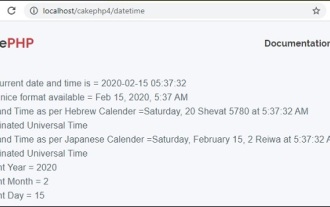
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
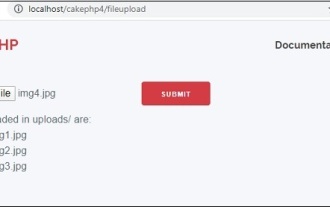
To work on file upload we are going to use the form helper. Here, is an example for file upload.
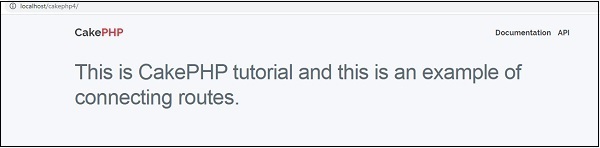
In this chapter, we are going to learn the following topics related to routing ?
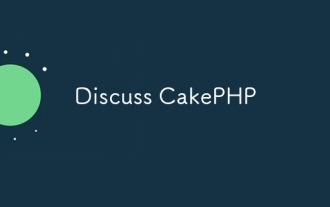
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
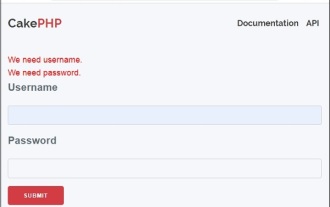
Validator can be created by adding the following two lines in the controller.
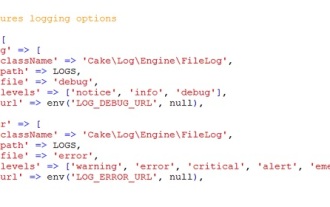
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
