How to create perfect form validation with PHP
In web development, form validation is a very important part. It can ensure that the user's input data conforms to the specified format and rules, effectively preventing some unnecessary errors and malicious behaviors. As a powerful and popular programming language, PHP can implement form validation functions by writing code. But, how to create perfect form validation with PHP? This article will introduce you in detail from the following aspects.
1. Call form submission and verification
To use PHP to implement form verification, you must first call the submit button in the HTML form. In an HTML form, you can use the <form>
tag to include form elements, such as <input>
, <textarea>
, etc. In the PHP code, you need to specify the request method corresponding to the form submission, that is, $_REQUEST
or $_POST
.
For example, in an HTML form, suppose we define a text box for entering a user name. Then, its submission and verification can be called like this in PHP code:
<?php if(isset($_REQUEST['submit'])){ $username = $_POST['username']; //进行验证 } ?>
Here, the isset()
function can check whether the request exists and return true
or false
. The $_POST
refers to the form data submitted through the POST request.
2. Verify form input data
When validating a form, you usually need to check whether the user's input data conforms to the specified format and rules. For example, for the entered username, we can check whether its length exceeds the limit, whether it contains illegal characters, etc. Common form verification methods are as follows:
1. Check the input format: Verify whether the format of the input data complies with the protocol. For example, when validating an email address, you can use regular expressions to check whether it is formatted correctly.
if(preg_match('/^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,4}$/',$_POST['email'])){ //合法 }
2. Check the string length: Verify whether the length of the input string meets the requirements. For example, when validating a username, you can check if its length is between 6-20 characters.
if( strlen($username) >= 6 && strlen($username) <= 20 ){ //合法 }
3. Check whether it is empty: Verify whether the required fields are entered, and detect whether the data is empty. For example, when validating a password, you can check to see if it is empty.
if(empty($_REQUEST['password'])){ //密码为空,需要填写 }
3. Processing form submission data
After verification, the input data of the form already conforms to the specified rules and formats, but we still need to process the data, such as saving it to the database or send an email, etc. In PHP code, you can use the $_REQUEST
and $_POST
global variables to get the form submission information and then process it. For example:
<?php if( isset($_REQUEST['submit']) ){ $username = trim($_POST['username']); $email = trim($_POST['email']); $password = trim($_POST['password']); //将数据保存到数据库中 mysqli_query($db,"INSERT INTO user (username,email,password) VALUES ('$username','$email','$password')"); //发送电子邮件 $to = $email; $subject = '注册成功'; $message = '恭喜您!您已经成功注册本站账户。'; $headers = 'From: webmaster@example.com'; mail($to,$subject,$message,$headers); } ?>
4. Handling error information
When performing form verification, some errors often occur, such as the input data format is incorrect, required fields are empty, etc. In response to these error situations, we need to provide timely feedback to users and prompt correct operation steps. In PHP code, you can use the echo
statement and the die()
function to output error information. The example is as follows:
if(preg_match('/^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,4}$/',$_POST['email'])){ //合法 }else{ echo '输入的邮箱地址不正确,请重新输入!'; die(); }
5. Conclusion
PHP form validation is a very important part of web development. During the implementation process, user experience, security, and code readability need to be fully taken into consideration. By introducing the above aspects in detail, I believe you can already master how to use PHP to create perfect form validation.
The above is the detailed content of How to create perfect form validation with PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


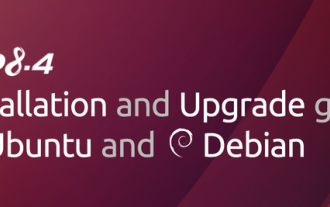
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
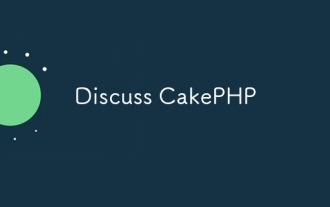
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
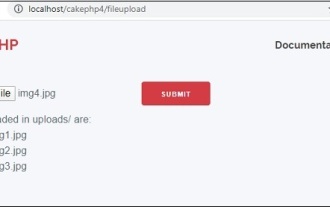
To work on file upload we are going to use the form helper. Here, is an example for file upload.
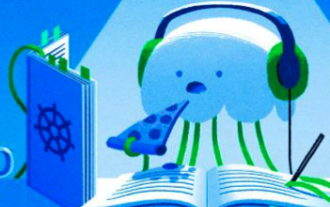
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
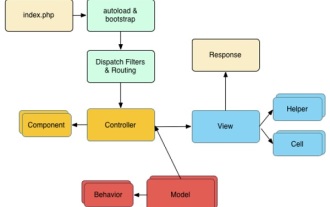
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
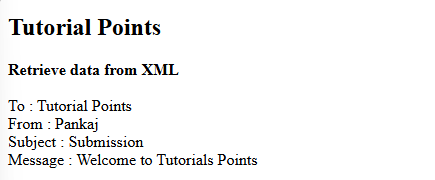
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
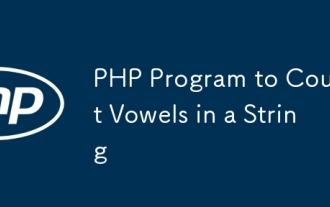
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
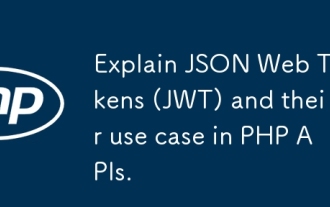
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
