How to use PHP to implement OSS cloud storage
In recent years, cloud storage has become more and more widely used, and Alibaba Cloud's OSS cloud storage service has also occupied a place in the domestic market. On this basis, this article will share how to use PHP to implement OSS cloud storage.
First, we need to register an account on the Alibaba Cloud official website and create an OSS storage space. The creation steps are described in detail in the official documentation and will not be repeated here.
Next, we need to install the OSS SDK for PHP provided by Alibaba Cloud. Use the following command in the terminal to install:
composer require aliyuncs/oss-sdk-php
After the installation is complete, we can connect and set up the OSS client through the following code:
use OSSOssClient; use OSSCoreOssException; $accessKeyId = '<Your AccessKeyId>'; $accessKeySecret = '<Your AccessKeySecret>'; $endpoint = '<Your endpoint>'; $bucket = '<Your bucket name>'; try { //创建OSS客户端连接 $ossClient = new OssClient($accessKeyId, $accessKeySecret, $endpoint); //设置存储空间默认ACL设置为私有 $ossClient->putBucketAcl($bucket, OssClient::OSS_ACL_TYPE_PRIVATE); } catch (OssException $e) { //连接失败处理 }
Among them, $accessKeyId
and $accessKeySecret
are the AccessKey ID and AccessKey Secret obtained through the Alibaba Cloud official website, $endpoint
is the access domain name of the OSS service, and $bucket
is the created storage space name.
Next, we can upload and delete files through the following code:
$file = '<Your local file path>'; $object = '<Your object name>'; try { //上传文件到指定的存储空间中 $ossClient->uploadFile($bucket, $object, $file); //删除存储空间中的指定文件 $ossClient->deleteObject($bucket, $object); } catch (OssException $e) { //处理上传或删除文件失败的情况 }
Among them, $file
is the local file path that needs to be uploaded, $object
is the name of the object stored in OSS. We can also download files through the following code:
$localFile = '<Your local file path>'; try { //从存储空间中下载指定名称的文件到指定本地路径 $ossClient->getObject($bucket, $object, ['fileDownload' => $localFile]); } catch (OssException $e) { //处理下载文件失败的情况 }
When uploading and downloading files, we can set some optional parameters, such as the file's ACL, Content-Type, etc. Specific parameters can be found in the official documentation.
Finally, when we do not need to use the OSS client, we can close and clean up the client through the following code:
try { //断开OSS客户端连接并清理客户端实例 $ossClient->close(); } catch (OssException $e) { //处理断开OSS客户端连接失败的情况 }
The above is the basic process of using PHP to implement OSS cloud storage. Through these codes, we can easily implement functions such as file upload, download, and deletion in OSS storage space. In practical applications, we can also perform parameter settings and function expansion according to our own needs.
The above is the detailed content of How to use PHP to implement OSS cloud storage. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


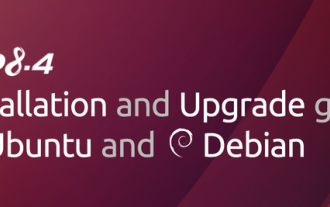
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
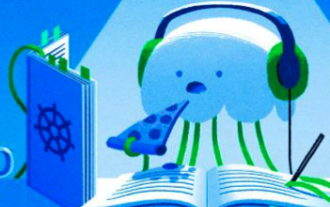
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
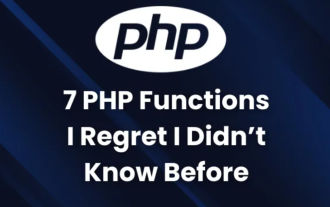
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
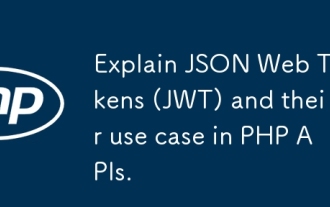
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
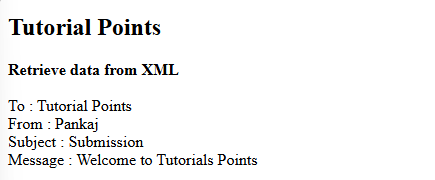
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
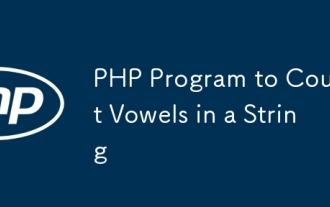
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
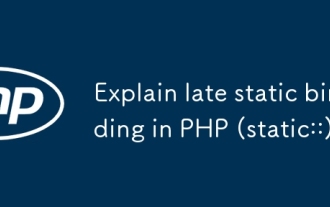
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
