


How to write an artificial intelligence-based target detection system using Java
With the increasing development of artificial intelligence technology, target detection systems play an extremely important role in the field of computer vision. This kind of system can automatically identify key objects from images or videos to track and analyze targets. In this article, we will introduce how to write an artificial intelligence-based object detection system using Java.
- Install and configure Java and OpenCV
First, we need to install Java and OpenCV. Java is a programming language for writing target detection systems using Java, and OpenCV is a computer vision library that provides many practical APIs and tools to process and analyze images. We can install and configure Java and OpenCV through the following steps:
1.1 Install Java JDK
Java JDK can be downloaded from the official website. After the installation is complete, you need to set the JAVA_HOME and PATH environment variables. In Windows systems, you can add the following content to the environment variables:
Variable name: JAVA_HOME
Variable value: C:Program FilesJavajdk1.8.0_45
Variable name: PATH
Variable value: %JAVA_HOME% in
1.2 Install OpenCV
OpenCV can be downloaded from the official website. Once the download is complete, unzip the file and copy it to your project's working directory. Then copy the opencv-3.4.3.jar file in the folder to the lib folder of the project. Finally, add the third-party local library folder opencv uildjavad (under Windows) or opencv uildjavalib (under Linux) in the folder to the system's path environment variable.
- Load images and process them
After we have completed the installation and configuration of Java and OpenCV, we can start building the target detection system. First, we need to load the image and perform some basic operations. We can use the following code to achieve these operations:
public static void main(String[] args) { // 载入图像 Mat image = Imgcodecs.imread("test.jpg"); // 缩放图像 Size size = new Size(800,600); Imgproc.resize(image,image,size); // 转换为灰度图像 Mat grayImage = new Mat(); Imgproc.cvtColor(image,grayImage,Imgproc.COLOR_BGR2GRAY); // 模糊处理 Imgproc.GaussianBlur(grayImage,grayImage,new Size(3,3),0); // 边缘检测 Mat edges = new Mat(); Imgproc.Canny(grayImage,edges,50,150); // 显示图像 HighGui.imshow("Test",edges); HighGui.waitKey(0); System.exit(0); }
In this code, we first use the Imgcodecs.imread() function to load the image from the local file system. Then, we use the Imgproc.resize() function to scale the image to 800×600 dimensions. Next, we convert the image to grayscale using the Imgproc.cvtColor() function. Then, we use the Imgproc.GaussianBlur() function to blur the grayscale image to reduce noise and interference. Finally, we use the Imgproc.Canny() function for edge detection for subsequent target detection.
- Target detection
After basic image processing, we can start target detection. In order to achieve this function, we need to first choose a suitable target detection algorithm. In this article, we will use an OpenCV-based Haar feature classifier for object detection. The specific implementation steps are as follows:
3.1 Choose the appropriate Haar classifier
Haar classifier is a classic target detection algorithm. Its basic idea is to identify objects by observing their morphological characteristics. . Specifically, the Haar classifier uses an internal feature value to represent the target, which is obtained by calculating the difference in gray value within the target area. If the morphological characteristics of the target object can be represented by a set of feature values, then we can determine whether the target object exists in an image by comparing these feature values.
In this article, we will use the trained Haar feature classifier provided by OpenCV for target detection. These classifiers already contain a large number of positive and negative samples and can be trained through backpropagation.
3.2 Training Haar classifier
In order to start artificial intelligence target detection, we need to use the built-in training tool of OpenCV to train the Haar classifier. The training process requires a set of positive and negative sample images. Usually, the more sample images, the better the effect.
3.3 Target detection
Through the above steps, we have completed the training of the Haar classifier and can use it for target detection. In Java, we can use the following code to implement the target detection of the Haar classifier:
public static void main(String[] args) { // 载入图像 Mat image = Imgcodecs.imread("test.jpg"); // 装载分类器 CascadeClassifier detector = new CascadeClassifier("classifier.xml"); MatOfRect targets = new MatOfRect(); // 检测目标 detector.detectMultiScale(image,targets); // 在图像上标示目标 for(Rect rect: targets.toArray()){ Imgproc.rectangle(image,rect.tl(),rect.br(),new Scalar(0,0,255),2); } // 显示图像 HighGui.imshow("Test",image); HighGui.waitKey(0); System.exit(0); }
In this code, we first use the Imgcodecs.imread() function to load the image from the local file system. We then build a classifier object using the CascadeClassifier class and use it to detect the image. The detection results are stored in an object of type MatOfRect. Finally, we use the Imgproc.rectangle() function to mark the target on the image, and use the HighGui.imshow() function to display the detection results.
- Summary
In this article, we introduced how to write an artificial intelligence-based target detection system using Java. We first introduced the installation and configuration of Java and OpenCV, then demonstrated some basic image processing functions, and finally implemented object detection using the Haar feature classifier. With this foundation of knowledge and skills, readers can further learn and explore more in-depth and advanced applications and technologies in target detection systems, such as YOLO, RCNN, etc., in order to better adapt to the evolving artificial intelligence era.
The above is the detailed content of How to write an artificial intelligence-based target detection system using Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


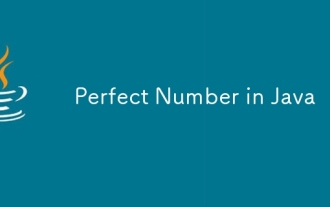
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
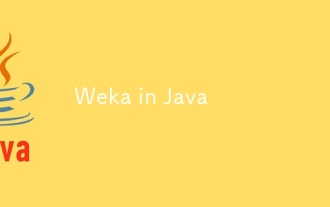
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
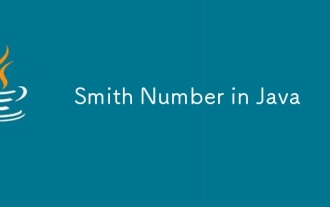
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
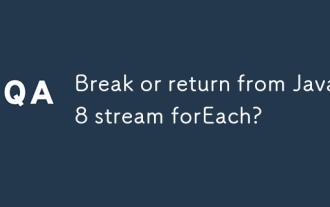
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
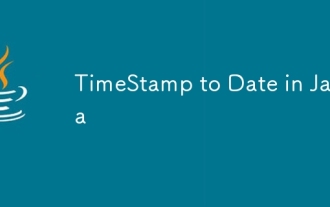
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
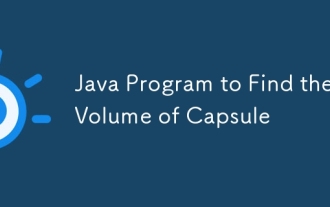
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
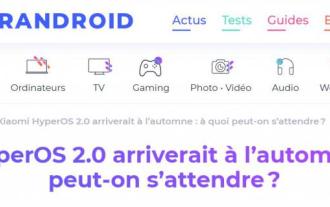
Recently, news broke that Xiaomi will launch the highly anticipated HyperOS 2.0 version in October. 1.HyperOS2.0 is expected to be released simultaneously with the Xiaomi 15 smartphone. HyperOS 2.0 will significantly enhance AI capabilities, especially in photo and video editing. HyperOS2.0 will bring a more modern and refined user interface (UI), providing smoother, clearer and more beautiful visual effects. The HyperOS 2.0 update also includes a number of user interface improvements, such as enhanced multitasking capabilities, improved notification management, and more home screen customization options. The release of HyperOS 2.0 is not only a demonstration of Xiaomi's technical strength, but also its vision for the future of smartphone operating systems.
