


Implementing collection sorting using PHP iterator function Iterators
As the amount of data continues to increase, set sorting has become a very important issue in computer science. When writing PHP code, iterator functions are often required to implement collection sorting. This article will introduce how to use the PHP iterator function Iterators to implement collection sorting.
First, we need to understand what an iterator is. Simply put, an iterator is an object that can access the elements of a collection on demand. In PHP, we can use iterator functions to implement objects with iteration capabilities. PHP has many built-in iterator functions, the most commonly used of which is Iterators. So, next let’s learn how to use Iterators to implement collection sorting.
First, we need to create a collection object. In PHP, a collection object is usually an array. We can use the following code to create a collection object named $array and add some data to it:
$array = array(10, 50, 30, 20, 40);
Next, we need to use the iterator function IteratorAggregate to implement an object with iteration capabilities. In PHP, this object is usually a class instance. Here is the class definition we can use:
class MyIterator implements IteratorAggregate { private $array; public function __construct($array) { $this->array = $array; } public function getIterator() { return new ArrayIterator($this->array); } }
In the above code, we have implemented the IteratorAggregate interface and accepted an array object $array in the class constructor. In the getIterator method, we wrap the $array object into an ArrayIterator, thereby creating an object with iteration capabilities. Next, we can use the following code to create a collection object with iteration capabilities:
$collection = new MyIterator($array);
Now, we can use the iterator function to sort the data in the collection object. In PHP, we can use the iterator function IteratorIterator to iterate over the elements in a collection. We can then collect these elements into an array and sort the array using the PHP built-in function sort(). In this way, we can sort the collection objects.
The following is the sorting code implementation:
$iterator = new IteratorIterator($collection); $temp_arr = array(); foreach ($iterator as $value) { $temp_arr[] = $value; } sort($temp_arr);
In the above code, we instantiate an IteratorIterator so that we can access the elements in $collection in order. In the foreach loop, we save the elements from the collection object into the $temp_arr array. We then sort the array using PHP's sort function.
So far, we have successfully implemented sorting of collection objects. However, the sorted results are not returned to the original collection object. In order to return the sorted results into a collection object, we need to use the iterator function ArrayIterator.
The following is the implementation of the sorted results:
$collection = new ArrayIterator($temp_arr); foreach ($collection as $value) { echo $value." "; }
In the above code, we save the sorted results into an ArrayIterator object. We then use a foreach loop to sequentially access the elements in $collection and output them to the screen one after another.
Through the above implementation, we have successfully used the PHP iterator function Iterators to implement collection sorting. If you want to learn more about the usage of iterator functions, you can refer to the PHP official documentation.
The above is the detailed content of Implementing collection sorting using PHP iterator function Iterators. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
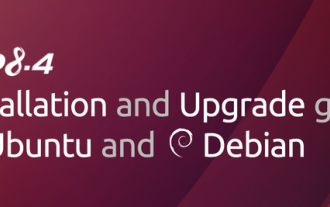
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
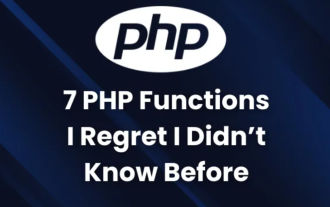
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
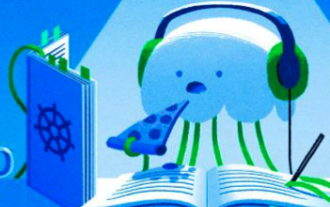
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
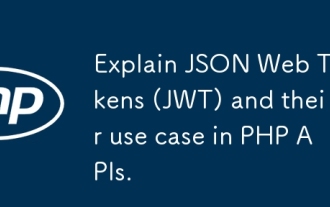
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
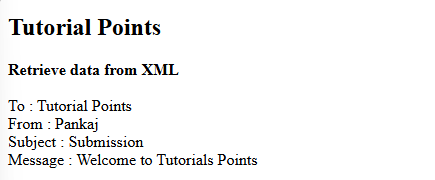
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
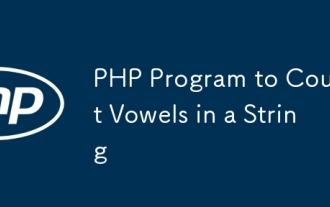
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
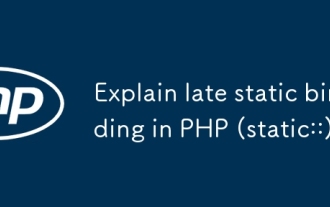
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
