How to use PHP to implement weather forecast function
As a popular back-end programming language, PHP is widely popular in the field of web development. The weather forecast function is a common web application scenario. Implementing the weather forecast function based on PHP is relatively simple and easy to understand. This article will introduce how to use PHP to implement the weather forecast function.
1. Obtain weather data API
To implement the weather forecast function, you first need to obtain weather data. We can use third-party weather APIs to obtain real-time, accurate weather data. At present, the mainstream weather API providers in China include the free "Xinzhi Weather" and the paid "Aggregation Data Weather".
Taking Xinzhi Weather as an example, we first apply for a developer account on its official website and obtain a free API key. Developer account registration is free, and each account has 1,000 free calls per day by default.
Xinzhi Weather provides many types of APIs, including real-time weather, hourly weather, daily weather, etc. Just select the corresponding API according to actual needs. In this article, we choose to use the real-time weather API to obtain current weather data.
2. Parse JSON format data
The weather data returned by Xinzhi Weather is returned in JSON format. Therefore, we need to learn how to parse JSON format data.
In PHP, you can use the built-in function json_decode() to convert JSON format data into an object or array. For example:
$json_string = '{"name":"Peter","age":21,"city":"New York"}'; $data = json_decode($json_string); echo $data->name;
In the above code, we convert a JSON format string into the object $data, and then output the value "Peter" of its name attribute.
If you do not want to convert JSON data to an object, but want to convert it to an array, you can set the second parameter of the json_decode() function to true. For example:
$json_string = '{"name":"Peter","age":21,"city":"New York"}'; $data = json_decode($json_string, true); echo $data['name'];
3. Use CURL to get data
To get data from the API server, we can use the CURL library in PHP. CURL is a file transfer protocol based on the HTTP protocol, often used to interact with web servers, receive and send data.
In PHP, you can use the curl_init() function to initialize a CURL session, and then use the curl_setopt() function to set some parameters, such as request URL, request method, request header information, request body data, etc. Finally, use the curl_exec() function to execute the CURL session and obtain the data returned by the server.
For example, the following code is an example of using CURL to obtain real-time weather data from the Xinzhi Weather API:
$url = 'https://api.seniverse.com/v3/weather/now.json'; $params = [ 'key' => 'your-api-key', // 替换成你自己的API key 'location' => '北京', 'language' => 'zh-Hans', 'unit' => 'c' ]; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url . '?' . http_build_query($params)); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); $data = json_decode($response, true); echo '当前城市:' . $data['results'][0]['location']['name'] . '<br>'; echo '当前温度:' . $data['results'][0]['now']['temperature'] . '℃<br>'; echo '天气状况:' . $data['results'][0]['now']['text'];
In the above code, we use the http_build_query() function to convert the parameter array is the query string in the URL to send the request parameters to the API server. Then use the json_decode() function to parse the JSON format data returned by the server into an array, and finally output the city, temperature and weather conditions information.
4. Use template syntax to output data
After we obtain the weather data, we need to render it to the front-end page. In order to separate the data from the front-end page, we can use PHP's template engine to achieve this.
Common PHP template engines include Smarty, Blade, Twig, etc. Here we take Blade as an example to introduce how to render weather data to the front-end page.
First, we define a placeholder in the Blade template to display weather data. For example:
<div class="weather"> <p>当前城市:{{ $city }}</p> <p>当前温度:{{ $temperature }}℃</p> <p>天气状况:{{ $text }}</p> </div>
Then, we output the obtained weather data to the placeholder in the PHP code. For example:
$data = json_decode($response, true); $view = new JenssegersBladeBlade(__DIR__.'/views', __DIR__.'/cache'); echo $view->make('weather', [ 'city' => $data['results'][0]['location']['name'], 'temperature' => $data['results'][0]['now']['temperature'], 'text' => $data['results'][0]['now']['text'], ])->render();
In the above code, we first use the constructor of Blade to create a view object $view. Among the constructor parameters, the first parameter is the path to the view folder, and the second parameter is the path to the cache folder.
Then, we use the make() method of the view object to pass in the view file name and the data to be rendered to generate a view instance. Finally, the view content is output using the render() method of the view instance.
5. Complete code example
Finally, we integrate the above code into a complete PHP file to implement the weather forecast function. The complete code is as follows:
<?php require_once 'vendor/autoload.php'; $url = 'https://api.seniverse.com/v3/weather/now.json'; $params = [ 'key' => 'your-api-key', // 替换成你自己的API key 'location' => '北京', 'language' => 'zh-Hans', 'unit' => 'c' ]; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url . '?' . http_build_query($params)); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); $data = json_decode($response, true); $view = new JenssegersBladeBlade(__DIR__.'/views', __DIR__.'/cache'); echo $view->make('weather', [ 'city' => $data['results'][0]['location']['name'], 'temperature' => $data['results'][0]['now']['temperature'], 'text' => $data['results'][0]['now']['text'], ])->render();
In this sample code, we introduce the Blade template engine dependency through the vendor/autoload.php file. We create a blade template in the views folder with placeholders for rendering weather data. Finally, we pass the data into the template through the view object and render the final weather forecast page.
6. Conclusion
Using PHP to implement the weather forecast function is a common web development requirement. With the help of third-party weather API, we can easily obtain weather data. Then, use the CURL library to obtain data, the json_decode() function to parse the data, and the Blade template engine to output the data, and the weather forecast function can be completely realized. Although the above steps are a bit cumbersome, they are relatively easy to get started once you master them.
The above is the detailed content of How to use PHP to implement weather forecast function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


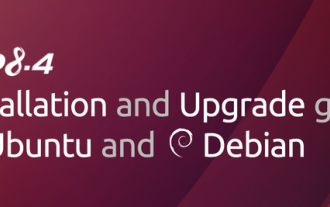
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
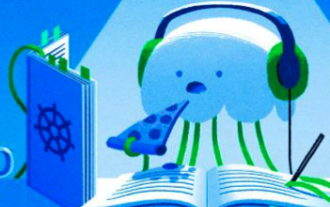
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
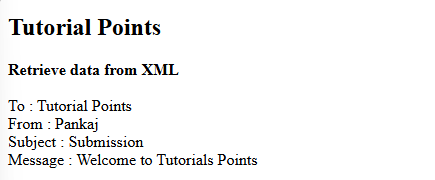
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
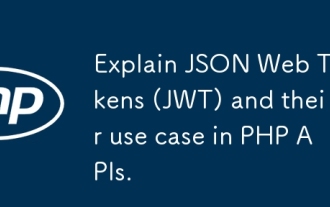
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
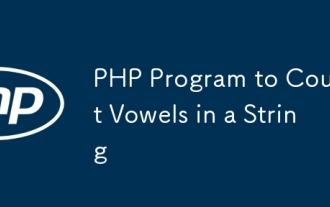
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
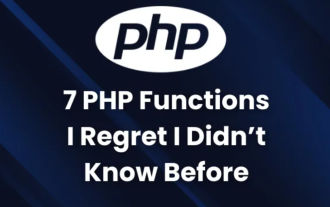
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
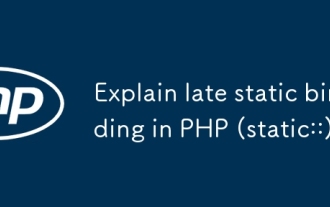
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
