Real-time data communication using PHP and SignalR
With the rapid development of mobile Internet, real-time communication has gradually become a very important requirement. In real-time communication, the most basic requirement is real-time data communication, which requires the server to be able to send data to the client in real time and interact in real time.
When implementing real-time data communication, PHP and SignalR are two very powerful tools. PHP is a very popular development language that can be used to write server-side code, while SignalR is a real-time communication framework that can be used to implement real-time data communication.
In this article, we will introduce in detail how to use PHP and SignalR to achieve real-time data communication. We will first introduce the basic concepts and working principles of SignalR, and then introduce how to use PHP and SignalR to build the server and client for real-time data communication. Finally, we will use an example to demonstrate how to use PHP and SignalR to achieve real-time data communication.
1. Basic concepts and working principles of SignalR
SignalR is a real-time communication framework that can be used to achieve real-time data communication. It is based on WebSockets, long polling, Server-Sent Events (SSE) and other technologies, and can perform real-time data communication on different browsers and operating systems.
The working principle of SignalR is very simple. When the client establishes a connection with the server, SignalR will automatically select the most suitable communication method (WebSockets, long polling, SSE, etc.) and establish a persistent connection between the server and the client. When the server has new data that needs to be pushed to the client, SignalR will automatically send the data to the client and trigger corresponding events on the client so that the client can process the data.
2. Use PHP and SignalR to build the server and client for real-time data communication
To use PHP and SignalR to build the server and client for real-time data communication, we need to install SignalR first PHP library. You can install SignalR's PHP library by using Composer. The specific steps are as follows:
- Install Composer
First, we need to install Composer. Composer can be installed from the command line with the following command:
curl -sS https://getcomposer.org/installer | php
- Install SignalR’s PHP library
Use the following command to install the SignalR PHP library on the command line:
composer require signalwire/signalwire
After installing the SignalR PHP library, we can start writing the server and Client code.
The server-side code is as follows:
<?php require __DIR__ . '/vendor/autoload.php'; use SignalWireRestClient; use SignalWireRelayStreamRoom; $client = new Client('YOUR_PROJECT_ID', 'YOUR_AUTH_TOKEN'); $room = new Room($client, 'YOUR_ROOM_ID'); $room->on('data', function ($data) use ($room) { // 处理接收到的数据 }); $room->join();
In the above code, we first create a client instance on the server side using SignalR's PHP library. Then, we registered a callback function in the client instance that handles the "data" event. When the client receives the data sent by the server, the callback function will be automatically called. Finally, we join the client to the SignalR room by calling the join() method.
The client code is as follows:
<!doctype html> <html> <head> <title>SignalR Example</title> <script src="https://code.jquery.com/jquery-3.4.1.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/@signalwire/js@0.14.2/dist/signalwire.min.js"></script> </head> <body> <script> const signalwire = new window.SignalWire.WebSocketEngine({ host: 'relay.signalwire.com', project: 'YOUR_PROJECT_ID', token: 'YOUR_AUTH_TOKEN', room: 'YOUR_ROOM_ID', }); signalwire.on('ready', () => { // 客户端连接成功后的处理 }); signalwire.on('open', () => { // 客户端打开连接后的处理 }); signalwire.on('data', (data) => { // 处理接收到的数据 }); </script> </body> </html>
In the above code, we first create a WebSocketEngine instance on the client side through SignalR's JavaScript library. We then registered some event callback functions on the WebSocketEngine instance to handle various events. Finally, we can send data to the server through the WebSocketEngine instance.
3. Example Demonstration
In order to demonstrate how to use PHP and SignalR to achieve real-time data communication, we can take a chat room as an example. In this chat room, users can enter some text messages on the client, and then the server will push these messages to other clients in real time for real-time interaction.
For specific code implementation, please refer to the following example:
// server.php <?php require __DIR__ . '/vendor/autoload.php'; use SignalWireRestClient; use SignalWireRelayStreamRoom; $client = new Client('YOUR_PROJECT_ID', 'YOUR_AUTH_TOKEN'); $room = new Room($client, 'YOUR_ROOM_ID'); $room->on('data', function ($data) use ($room) { foreach ($room->clients as $client) { $client->send($data); } }); $room->join();
<!-- index.html --> <!doctype html> <html> <head> <title>SignalR Example</title> <script src="https://code.jquery.com/jquery-3.4.1.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/@signalwire/js@0.14.2/dist/signalwire.min.js"></script> </head> <body> <div> <input type="text" id="input" /> <button id="send">Send</button> </div> <div id="messages"></div> <script> const signalwire = new window.SignalWire.WebSocketEngine({ host: 'relay.signalwire.com', project: 'YOUR_PROJECT_ID', token: 'YOUR_AUTH_TOKEN', room: 'YOUR_ROOM_ID', }); signalwire.on('ready', () => { console.log('Connected to the server.'); }); signalwire.on('open', () => { console.log('Connection opened.'); }); signalwire.on('data', (data) => { $('#messages').append('<p>' + data + '</p>'); }); $('#send').on('click', () => { const message = $('#input').val(); signalwire.send(message); $('#input').val(''); }); </script> </body> </html>
In the above code, we first create a SignalR room on the server. When the client connects to the server, it will Join this room. When one of the clients sends a message, the server sends this message to the other clients to achieve real-time communication.
In the client, we created a text input box and a "Send" button. When the user enters text in the text input box and clicks the "Send" button, the text will be sent to the server. , and then the server will send this text to other clients to achieve real-time communication.
Summary
It is very easy to use PHP and SignalR to achieve real-time data communication. You only need to use SignalR's PHP library and JavaScript library. When implementing real-time data communication, we can realize the connection between clients through SignalR's room, and use SignalR's events and callback functions to handle the interaction between the server and the client.
The above is the detailed content of Real-time data communication using PHP and SignalR. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


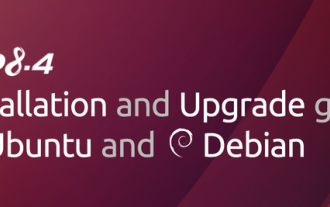
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
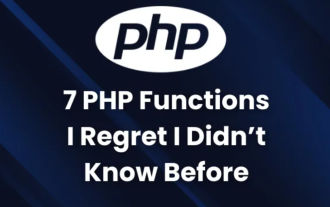
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
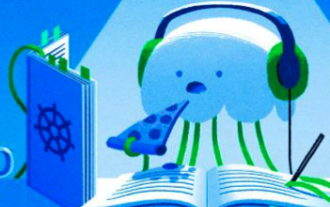
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
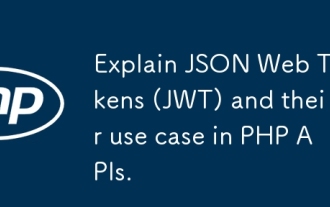
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
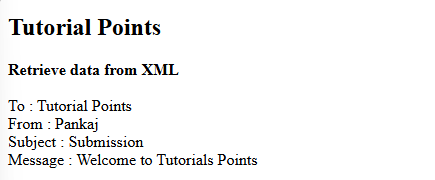
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
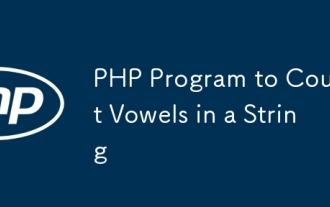
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
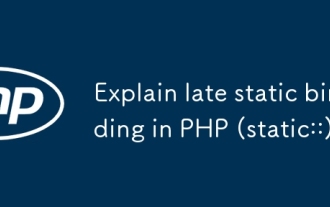
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
