


How to use PHP and Kafka to implement real-time game data analysis
With the booming development of the game market, game data analysis has gradually become an indispensable link for game developers and operators. The importance of real-time game data analysis is that it can help developers and operators understand game performance and player behavior as soon as possible, discover problems in a timely manner and take effective solutions.
In order to achieve real-time game data analysis, we can use the two tools PHP and Kafka. As a popular back-end programming language, PHP has high flexibility and scalability, and is also very simple to implement. Kafka is a high-performance, distributed message queue system that can ensure efficient and stable message delivery in large-scale data stream transmission.
Below we will introduce how to use PHP and Kafka to implement real-time game data analysis.
Step One: Install and Configure Kafka
First, we need to install Kafka and configure it accordingly. You can download the stable version from the Kafka official website. Once the download is complete, place the unzipped directory anywhere on the server.
Next, we need to add the following content to Kafka’s configuration file server.properties:
advertised.listeners=PLAINTEXT://[server_ip]:9092
Among them, [server_ip] is your server IP address.
Step 2: Create a Kafka topic
Next, we need to create a Kafka topic. Kafka topic is a category in the message queue system and can be understood as a container for messages. We can create topics using the command line tools that come with Kafka. Enter the following command in the terminal:
bin/kafka-topics.sh --bootstrap-server [server_ip]:9092 --create --replication-factor 1 --partitions 1 --topic [topic_name]
Where [server_ip] is your server IP address, [topic_name] is the topic name you defined. After creating the theme, we can use the following command to check whether the creation is successful:
bin/kafka-topics.sh --bootstrap-server [server_ip]:9092 --list
If the name of the theme you created is displayed in the list, it means the theme was created successfully.
Step 3: Write PHP code
Next, we need to write PHP code to send messages to Kafka. We can do this using the PHP client library officially provided by Kafka. Execute the following command in the terminal to install this library:
composer require rdkafka/rdkafka
After the installation is complete, we can use this library in PHP code. The specific code is as follows:
<?php require_once __DIR__ . '/vendor/autoload.php'; $conf = new RdKafkaConf(); $conf->set('metadata.broker.list', '[server_ip]:9092'); $producer = new RdKafkaProducer($conf); $topic = $producer->newTopic('[topic_name]'); $message = "hello world"; $topic->produce(RD_KAFKA_PARTITION_UA, 0, $message); $producer->flush(1000);
Among them, [server_ip] is your server IP address, and [topic_name] is the topic name you defined.
In this code, we define a producer and then a topic. Next, we send a message to the topic.
Step 4: Write consumer code
We also need to write a consumer to get messages from Kafka and perform data analysis. The specific code is as follows:
<?php require_once __DIR__ . '/vendor/autoload.php'; $conf = new RdKafkaConf(); $conf->set('metadata.broker.list', '[server_ip]:9092'); $consumer = new RdKafkaConsumer($conf); $consumer->subscribe(['[topic_name]']); while (true) { $message = $consumer->consume(120 * 1000); if ($message) { // 进行数据分析 echo $message->payload . " "; } }
In this code, we define a consumer and subscribe it to the previously created topic. Next, we use a loop to continuously obtain messages from Kafka and perform data analysis operations.
So far, we have successfully used PHP and Kafka to implement real-time game data analysis. In this way, developers and operators can understand the performance of game data and user behavior in the first time, discover problems in a timely manner and take corresponding measures.
The above is the detailed content of How to use PHP and Kafka to implement real-time game data analysis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


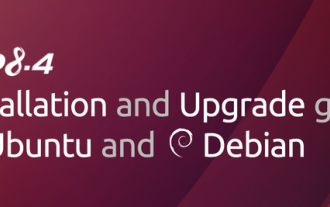
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
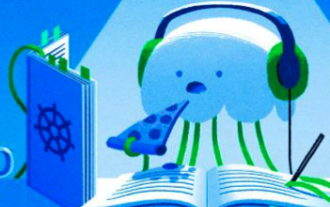
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
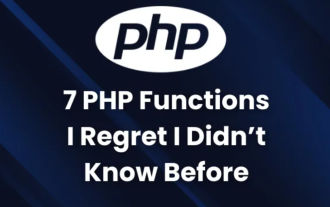
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
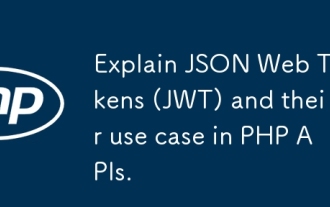
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
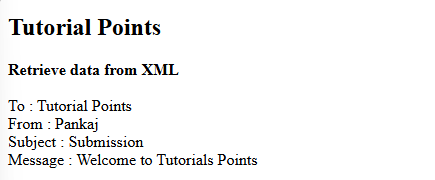
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
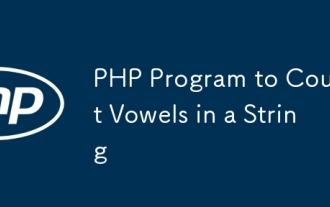
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
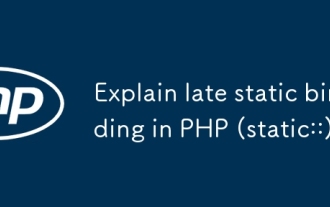
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
