


How to implement real-time IoT security monitoring using PHP and Kafka
The rapid development of the Internet of Things has brought us a lot of convenience, but it has also brought many security risks. In the Internet of Things system, there are a large number of devices, various types of devices, and the system is large and complex. How to ensure network security has become a very important matter. This article will help you better protect the security of the IoT system by introducing how to use PHP and Kafka to implement real-time IoT security monitoring.
1. What is PHP and Kafka
PHP is an open source multi-purpose scripting language, mainly used for web development. It can be embedded in HTML and also supports various database operations. PHP is easy to learn and has low entry barriers, so it is widely used in the field of web development.
Kafka is a distributed message queue system, mainly used in large-scale data processing scenarios. Kafka can realize functions such as message passing, processing, and storage, and has the characteristics of high reliability and high performance. Kafka is mainly written in Scala, but there is also a Kafka client for PHP language.
2. Internet of Things System Security Monitoring Plan
To achieve security monitoring of the Internet of Things system, the following aspects need to be considered:
1. Data collection: collecting objects Data generated by various devices and sensors in the networked system include key data such as device status, device health, and traffic usage.
2. Data processing: parse, analyze and process the collected data, and extract key information, such as device abnormality information, network attack information, etc.
3. Data transmission: When transmitting the processed information to the monitoring center, how to ensure the real-time and accuracy of the information is an important consideration.
4. Data storage: Store monitoring data in the database for better analysis and mining, and improve monitoring effectiveness and performance.
5. Abnormal warning: When the monitoring system detects an abnormal situation, it must issue a timely warning so that the administrator can take timely measures.
3. Use PHP and Kafka to implement real-time IoT security monitoring
The following will introduce how to use PHP and Kafka to implement real-time monitoring for each aspect of the IoT system security monitoring solution:
1. Data collection
Devices and sensors in the Internet of Things system can communicate with the back-end server through protocols such as MQTT and CoAP and upload data. The backend server can use the RESTful API interface to receive data and upload it. In PHP, you can use the cURL library to handle HTTP requests. The following is a simple PHP code example:
$url = "http://example.com/api/data"; //API接口地址 $data = array( 'device_id' => '123', 'timestamp' => '1621557645', 'data' => array( 'temperature' => 25, 'humidity' => 50, 'light' => 100 ) ); $options = array( CURLOPT_RETURNTRANSFER => true, CURLOPT_POST => true, CURLOPT_POSTFIELDS => json_encode($data), CURLOPT_HTTPHEADER => array('Content-Type: application/json'), ); $ch = curl_init($url); curl_setopt_array($ch, $options); $response = curl_exec($ch); curl_close($ch); echo $response;
2. Data processing
PHP itself is a scripting language that can easily process data. Data processing can be processed through PHP's built-in functions and extensions, or through custom functions. The following is a simple PHP code example:
$data = '{"device_id":"123","timestamp":"1621557645","data":{"temperature":25,"humidity":50,"light":100}}'; $json = json_decode($data, true); //将JSON转成PHP数组 $temperature = $json['data']['temperature']; $humidity = $json['data']['humidity']; $light = $json['data']['light']; if ($temperature > 30) { echo "Warning: Temperature too high!"; } if ($humidity > 80) { echo "Warning: Humidity too high!"; } if ($light < 50) { echo "Warning: Light too low!"; }
3. Data transmission
Kafka is a distributed message queue system that can achieve high concurrency and high reliability of message transmission. Kafka provides a variety of client libraries such as Java, Scala, Python, and PHP to facilitate message delivery. The following is a simple PHP code example:
$config = KafkaConsumerConfig::getInstance(); $config->setMetadataRefreshIntervalMs(10000); $config->setMetadataBrokerList('kafka1.example.com:9092,kafka2.example.com:9092,kafka3.example.com:9092'); $config->setGroupId('mygroup'); $consumer = new KafkaConsumer(); $consumer->setLogger(new KafkaLogEchoLogger()); $topics = array('mytopic'); $consumer->subscribe($topics); while (true) { $message = $consumer->consume(); if (empty($message)) { continue; } $data = json_decode($message->payload, true); //将消息转成PHP数组 //处理数据逻辑 }
4. Data storage
In real-time monitoring, it is usually necessary to store the monitored data. MySQL is a commonly used relational database with good ability to store and manage data. This can be done using mysqli or PDO extensions in PHP. The following is a simple PHP code example:
$host = 'localhost'; $username = 'username'; $password = 'password'; $database = 'mydatabase'; $conn = new mysqli($host, $username, $password, $database); if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } $sql = "INSERT INTO data (device_id, timestamp, temperature, humidity, light) VALUES (?, ?, ?, ?, ?)"; $stmt = $conn->prepare($sql); $stmt->bind_param("ssiii", $device_id, $timestamp, $temperature, $humidity, $light); $device_id = '123'; $timestamp = '1621557645'; $temperature = 25; $humidity = 50; $light = 100; $stmt->execute(); $stmt->close(); $conn->close();
5. Abnormal warning
When abnormal data is detected, notifications can be sent through various methods such as email, SMS or APP push. There are a variety of third-party libraries in PHP that can easily send emails or text messages. The following is a simple PHP code example:
use PHPMailerPHPMailerPHPMailer; $mail = new PHPMailer(); $mail->isSMTP(); $mail->Host = 'smtp.example.com'; $mail->SMTPAuth = true; $mail->Username = 'username'; $mail->Password = 'password'; $mail->SMTPSecure = 'tls'; $mail->Port = 587; $mail->setFrom('from@example.com', 'MyAPP'); $mail->addAddress('to@example.com', 'User'); $mail->Subject = 'Warning: Temperature too high!'; $mail->Body = 'Temperature has reached to 35.0 °C!'; $mail->send();
IV. Summary
This article introduces how to use PHP and Kafka to implement real-time IoT security monitoring. When implementing IoT system monitoring, multiple aspects need to be considered, such as data collection, processing, transmission, storage, and abnormal warning. PHP is a scripting language that is easy to learn and can easily handle data processing and send requests. Kafka is a distributed message queue system that can achieve high concurrency and high reliability of message transmission. By combining PHP and Kafka, various problems in security monitoring of IoT systems can be well solved and the effectiveness and performance of monitoring can be improved.
The above is the detailed content of How to implement real-time IoT security monitoring using PHP and Kafka. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


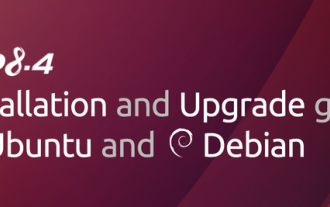
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
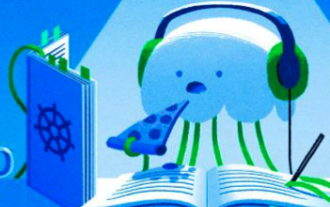
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
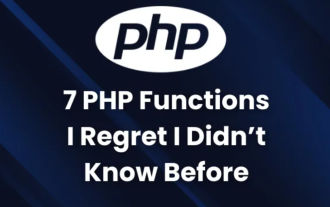
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
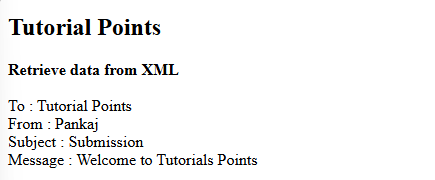
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
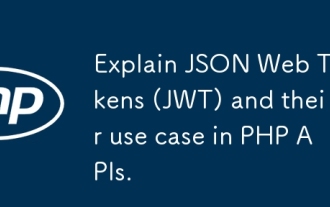
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
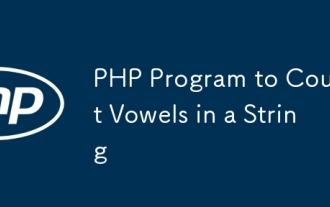
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
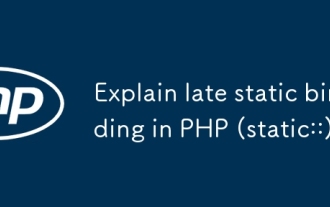
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
