Basic operations and usage of arrays in PHP
Basic operations and usage of arrays in PHP
1. Overview
Array is a very important data type in PHP. It can be used to store multiple values, and can Access these values via index or key. Arrays have rich operations and usage methods in PHP. This article will introduce in detail the basic operations and usage methods of arrays in PHP.
2. Create arrays
In PHP, you can create arrays in two ways: countable arrays and associative arrays.
- Create a countable array
A countable array is an array that is arranged in order and indexed numerically. The following is how to create a countable array:
// Method 1: Use the array() function
$numbers = array(1, 2, 3, 4, 5);
// Method 2: Use [] (available from PHP 5.4)
$numbers = [1, 2, 3, 4, 5];
- Create an associative array
Associative array is an array stored with string keys and corresponding values. The following is how to create an associative array:
// Method 1: Use the array() function
$student = array("name" => "John", "age" => ; 18, "grade" => "A");
// Method 2: Use [] (available starting from PHP 5.4)
$student = ["name" => "John ", "age" => 18, "grade" => "A"];
3. Accessing array elements
You can access elements in the array through indexes or keys.
- Accessing countable arrays
For countable arrays, you can use indexing to access array elements. The index starts from 0 and increases sequentially.
$numbers = array(1, 2, 3, 4, 5);
echo $numbers[0]; // Output 1
echo $numbers[2 ]; // Output 3
- Accessing associative arrays
For associative arrays, you can use keys to access array elements.
$student = array("name" => "John", "age" => 18, "grade" => "A");
echo $student["name"]; // Output John
echo $student["age"]; // Output 18
4. Common array operations and functions
- Get the length of the array
Use the count() function to get the number of elements in the array.
$numbers = array(1, 2, 3, 4, 5);
echo count($numbers); // Output 5
- Traverse the array
PHP provides a variety of methods to traverse the array, the most common method is to use the foreach loop.
$numbers = array(1, 2, 3, 4, 5);
foreach ($numbers as $number) {
echo $number . " ";
}
//Output 1 2 3 4 5
- Add elements
You can use the [] or array_push() function to add elements to the array.
$numbers = array(1, 2, 3, 4, 5);
// Use [] to add elements
$numbers[] = 6;
// Use array_push() to add elements
array_push($numbers, 7);
print_r($numbers);
// Output Array (1, 2, 3, 4 , 5, 6, 7)
- Delete elements
You can use the unset() function to delete elements in the array.
$numbers = array(1, 2, 3, 4, 5);
unset($numbers[2]);
print_r($numbers );
// Output Array (1, 2, 4, 5)
5. Summary
This article introduces the basic operations and usage of arrays in PHP, including creating arrays and accessing array elements , get the array length, traverse the array, and add and remove elements. Arrays are a very important data type in PHP and are often used in actual development. Mastering the basic operations and usage of arrays can help developers process data more flexibly and efficiently.
The above is the detailed content of Basic operations and usage of arrays in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
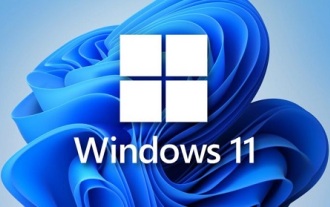
Some users need to change computers, but are afraid that the previous win11 key will no longer be usable after changing computers, so they want to know whether the win11 key can be used when changing computers. In fact, generally speaking, ordinary keys can only be given to one computer. Computer activated. Can I use the win11 key to change my computer? Answer: It is generally not possible to use the win11 key to change my computer. 1. Because ordinary win11 keys are one code for one machine. 2. Unless the key you purchased has been authorized to activate multiple computers, it can be used on different computers. 3. However, if you buy a computer now, it will support the pre-installed system and no manual activation is required. 4. If you assemble the computer yourself, you can also directly use a USB flash drive to install the system, and no activation is required. In most cases, win11

Question: Use C program to explain the concepts of post-increment and pre-increment of arrays. Solution Increment Operator (++) - There are two types of increment operators used to increase the value of a variable by 1 - pre-increment and post-increment. In prepended increment, the increment operator is placed before the operand, and the value is incremented first and then the operation is performed. eg:z=++a;a=a+1z=a The increment operator is placed after the operand in the post-increment operation, and the value will increase after the operation is completed. eg:z=a++;z=aa=a+1 Let us consider an example of accessing a specific element in a memory location by using pre-increment and post-increment. Declare an array of size 5 and perform compile-time initialization. Afterwards try assigning the pre-increment value to variable 'a'. a=++arr[1]
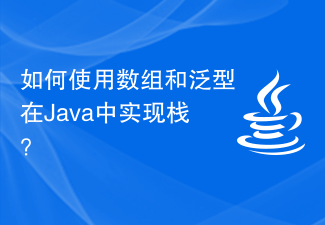
Java implements the stack by utilizing arrays and generics. This creates a versatile and reusable data structure that operates on the last-in-first-out (LIFO) principle. Following this principle, elements are added and removed from the top. By utilizing arrays as the basis, it ensures efficient memory allocation and access. Additionally, by incorporating generics, the stack is able to accommodate elements of different types, thereby enhancing its versatility. The implementation involves the definition of a Stack class containing generic type parameters. It includes basic methods such as push(), pop(), peek() and isEmpty(). Handling of edge cases, such as stack overflows and underflows, is also critical to ensure seamless functionality. This implementation enables developers to create programs that accommodate
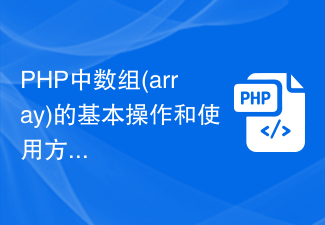
Basic operations and usage of arrays in PHP 1. Overview Array is a very important data type in PHP. It can be used to store multiple values, and these values can be accessed through indexes or keys. Arrays have rich operations and usage methods in PHP. This article will introduce in detail the basic operations and usage methods of arrays in PHP. 2. Create arrays In PHP, you can create arrays in two ways: countable arrays and associative arrays. Creating a Countable Array A countable array is an array that is arranged in order and indexed numerically
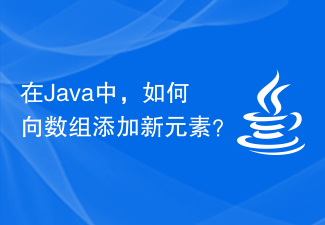
Adding new elements to an array is a common operation in Java and can be accomplished using a variety of methods. This article will introduce several common methods of adding elements to an array and provide corresponding code examples. 1. A common way to use a new array is to create a new array, copy the elements of the original array to the new array, and add new elements at the end of the new array. The specific steps are as follows: Create a new array whose size is 1 larger than the original array. This is because a new element is being added. Copy the elements of the original array to the new array. Add to the end of the new array
![Rearrange an array so that arr becomes arr] and only use O(1) extra space, implemented in C++](https://img.php.cn/upload/article/000/000/164/169319478769496.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
We get an array of positive integer type, say, arr[] of any given size, such that the element value in the array should be greater than 0 but less than the size of the array. The task is to rearrange an array only by changing arr[i] to arr[arr[i]] in the given O(1) space and print the final result. Let’s look at various input and output scenarios for this situation − Input − intarr[] = {032154} Output − Array before arrangement: 032154 Rearrange the array so that arr[i] becomes arr[arr[i]], And has O(1) extra space: 012345 Explanation − We are given an integer array of size 6, and all elements in the array have values less than 6. Now we will rearrange
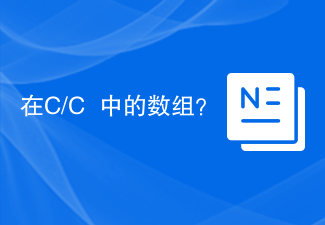
An array is a sequential collection of elements of the same type. Arrays are used to store collections of data, but it is often more useful to think of arrays as collections of variables of the same type. Instead of declaring a single variable such as number0, number1, ... and number99, you can declare an array variable (e.g. number) and represent it using numbers[0], numbers[1] and ..., numbers[99] each variable. Specific elements in the array are accessed through indexing. All arrays consist of contiguous memory locations. The lowest address corresponds to the first element, and the highest address corresponds to the last element. Declaring an ArrayDeclaring an array requires specifying the type of elements and the number of elements required. An array is as follows -ty
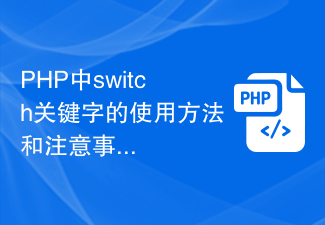
How to use and pay attention to the switch keyword in PHP In PHP programming, switch is a commonly used conditional statement that can execute different code blocks based on different values of variables. This article will introduce how to use the switch keyword and some things to pay attention to. Switch syntax structure: The switch statement consists of multiple cases and a default. Its basic syntax structure is as follows: switch (conditional expression) {case value 1:
