


How to deal with jump and redirect problems in PHP development
How to deal with jump and redirect issues in PHP development
In PHP development, jump and redirect are frequently used functions. Jumps allow users to jump from the current page to other pages, while redirects point users to a new URL address. Correctly handling jump and redirect issues can not only improve user experience, but also enhance website security. This article will introduce how to deal with jump and redirect problems in PHP development, and provide some practical tips.
1. Use the header() function to implement jumps and redirections
In PHP, you can use the header() function to implement jumps and redirections. This function is used to send HTTP header information, and jumps and redirects can be implemented by modifying the Location header. The following is a sample code that uses the header() function to implement jumps and redirects:
-
Jump to other pages
<?php header("Location: http://www.example.com"); exit(); ?>
Copy after loginThe above code will jump the user to http: //www.example.com page, and terminate the execution of the current page through the exit() function.
Redirect to new URL address
<?php header("HTTP/1.1 301 Moved Permanently"); header("Location: http://www.new-example.com"); exit(); ?>
Copy after loginThe above code uses HTTP status code 301 to indicate a permanent redirect, redirecting the user to http://www.new -example.com page.
2. Precautions and techniques
When dealing with jump and redirection problems, you also need to pay attention to the following matters and techniques:
Show friendly error message
When the jump or redirection fails, a friendly error message should be displayed to the user to help them understand the problem and its solution.<?php header("HTTP/1.1 500 Internal Server Error"); echo "跳转失败,请稍后再试"; exit(); ?>
Copy after loginThe above code uses HTTP status code 500 to indicate an internal error in the server and outputs error information to the user.
Prevent jump and redirect attacks
The jump and redirect functions may be exploited by hackers to carry out attacks, such as Phishing attacks. To prevent this from happening, the redirect and redirect target URLs need to be verified and only redirects to trusted URLs are allowed.<?php $url = $_GET['url']; // 获取用户传递的目标URL $allowedUrls = array('http://www.example.com', 'http://www.new-example.com'); // 允许的URL列表 if (in_array($url, $allowedUrls)) { header("Location: $url"); exit(); } else { header("HTTP/1.1 400 Bad Request"); echo "无效的目标URL"; exit(); } ?>
Copy after loginThe above code first obtains the target URL passed by the user and compares it with the allowed URL list. Only in the allowed URL list will the jump or redirection be performed.
Set jump delay time
In order to give the user some feedback or prompt time, you can set the jump delay time. This can be achieved using the Refresh header of the header() function.<?php header("Refresh: 5; url=http://www.example.com"); echo "将在5秒后跳转到 http://www.example.com"; exit(); ?>
Copy after loginThe above code will jump to the http://www.example.com page after a delay of 5 seconds.
Conclusion
In PHP development, dealing with jump and redirect issues is essential. Properly handling jumps and redirects can improve user experience and enhance website security. By using the header() function to implement jumps and redirections, and adding some common techniques, you can better handle jump and redirection problems. During the development process, we can flexibly use the above methods and other techniques to handle different situations according to specific needs.
The above is the detailed content of How to deal with jump and redirect problems in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
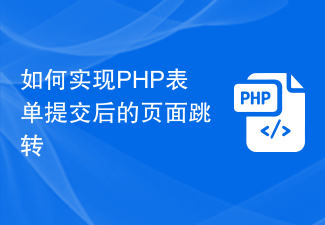
How to implement page jump after PHP form submission [Introduction] In web development, form submission is a common functional requirement. After the user fills out the form and clicks the submit button, the form data usually needs to be sent to the server for processing, and the user is redirected to another page after processing. This article will introduce how to use PHP to implement page jump after form submission. [Step 1: HTML Form] First, we need to write a page containing a form in an HTML page so that users can fill in the data that needs to be submitted.

PHP domain name redirection is an important network technology. It is a method of redirecting different domain names visited by users to the same main domain name. Domain name redirection can solve problems such as website SEO optimization, brand promotion, and user access, and can also prevent the abuse of malicious domain names. In this article, we will introduce the specific methods and principles of PHP domain name redirection.
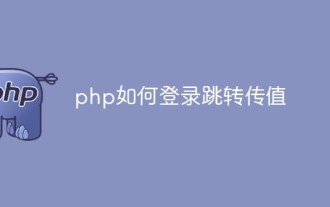
PHP login jump value transfer method: 1. POST value transfer, use the HTML "form" form jump method to post value transfer; 2. GET transfer value, use the <a> tag to jump to xxx.php , obtain the passed value through "$_GET['id']"; 3. SESSION passes the value. Once saved in SESSION, other pages can be obtained through SESSION.
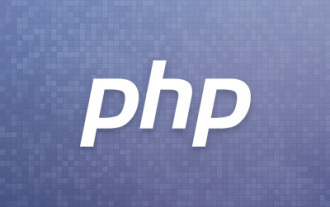
Redirects allow you to redirect client browsers to different URLs. You can use it when switching domains, changing website structure, or switching to HTTPS. In this article, I will show you how to redirect to another page using PHP. I'll explain exactly how PHP redirects work and show you what's happening behind the scenes. Learn PHP with Free Online Courses If you want to learn PHP, check out our PHP Basics free online course! PHP Basics Jeremy McPeak October 29, 2021 How do basic redirects work? Before we get into the details of PHP redirection, let’s take a quick look at how HTTP redirection actually works. Take a look at the image below. Let us understand the above screen
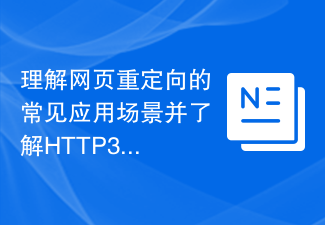
Understand the meaning of HTTP 301 status code: common application scenarios of web page redirection. With the rapid development of the Internet, people's requirements for web page interaction are becoming higher and higher. In the field of web design, web page redirection is a common and important technology, implemented through the HTTP 301 status code. This article will explore the meaning of HTTP 301 status code and common application scenarios in web page redirection. HTTP301 status code refers to permanent redirect (PermanentRedirect). When the server receives the client's
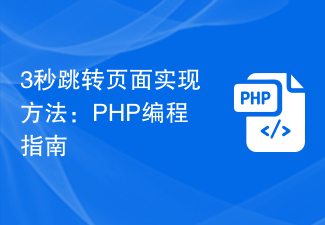
Title: Implementation method of page jump in 3 seconds: PHP Programming Guide In web development, page jump is a common operation. Generally, we use meta tags in HTML or JavaScript methods to jump to pages. However, in some specific cases, we need to perform page jumps on the server side. This article will introduce how to use PHP programming to implement a function that automatically jumps to a specified page within 3 seconds, and will also give specific code examples. The basic principle of page jump using PHP. PHP is a kind of
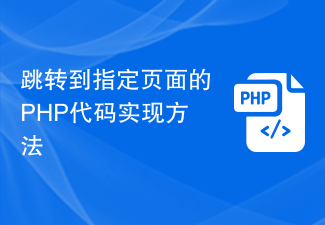
When writing a website or application, you often encounter the need to jump to a specific page. In PHP, we can achieve page jump through several methods. Below I will demonstrate three common jump methods for you, including using the header() function, using JavaScript code, and using meta tags. Using the header() function The header() function is a function used in PHP to send original HTTP header information. This function can be used in combination when implementing page jumps. Below is a
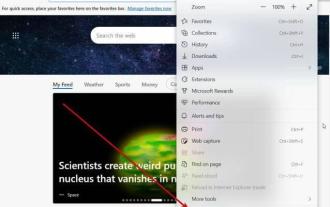
It's no secret that Internet Explorer has fallen out of favor for a long time, but with the arrival of Windows 11, reality sets in. Rather than sometimes replacing IE in the future, Edge is now the default browser in Microsoft's latest operating system. For now, you can still enable Internet Explorer in Windows 11. However, IE11 (the latest version) already has an official retirement date, which is June 15, 2022, and the clock is ticking. With this in mind, you may have noticed that Internet Explorer sometimes opens Edge, and you may not like it. So why is this happening? exist
