


Methods to solve the problem of concurrency race conditions in Go language development
Methods to solve the problem of concurrency race conditions in Go language development
In Go language development, due to its inherent support for concurrency, it is easy for race conditions to occur. A race condition refers to the competition between multiple threads or goroutines when accessing shared resources, leading to unpredictable results. This is caused by multiple threads or coroutines accessing and modifying shared data simultaneously.
Race conditions are a common problem, which may lead to serious problems such as incorrect calculation results, data corruption, and data overwriting. Therefore, we must take some measures to solve this problem.
First of all, we can use mutex locks (Mutex) to solve the problem of concurrency race conditions. Mutex locks can ensure that only one thread or coroutine can access protected shared resources at the same time. In Go language, we can lock and unlock by calling the Lock() and Unlock() methods in the code block.
The following is an example of using a mutex lock:
package main import ( "fmt" "sync" ) var ( counter int mutex sync.Mutex ) func main() { wg := sync.WaitGroup{} for i := 0; i < 100; i++ { wg.Add(1) go func() { defer wg.Done() increment() }() } wg.Wait() fmt.Println("Counter:", counter) } func increment() { mutex.Lock() defer mutex.Unlock() counter++ }
In the above example, we define a counter variable and a mutex lock mutex. In the increment() function, we first lock the lock by calling the mutex.Lock() method, then increment the counter by 1 in the code block, and finally unlock it by calling the mutex.Unlock() method.
By using a mutex lock, we can ensure that only one thread or coroutine can access and modify the counter variable at the same time, thus solving the problem of concurrency race conditions.
In addition to mutex locks, we can also use read-write locks (RWMutex) to improve performance. Read-write locks are divided into read locks and write locks. Multiple threads or coroutines can acquire read locks at the same time, but only one thread or coroutine can acquire write locks. This can improve concurrency performance in scenarios with more reads and less writes.
The following is an example of using a read-write lock:
package main import ( "fmt" "sync" ) var ( counter int rwMutex sync.RWMutex ) func main() { wg := sync.WaitGroup{} for i := 0; i < 100; i++ { wg.Add(1) go func() { defer wg.Done() increment() }() } wg.Wait() fmt.Println("Counter:", counter) } func increment() { rwMutex.Lock() defer rwMutex.Unlock() counter++ }
In the above example, we replace the mutex lock with a read-write lock. In the increment() function, we first add the lock by calling the rwMutex.Lock() method, then increment the counter by 1 in the code block, and finally unlock it by calling the rwMutex.Unlock() method.
By using read-write locks, we can ensure that only one thread or coroutine can write to the counter variable at the same time, but allow multiple threads or coroutines to read the counter variable at the same time, thus improving concurrency performance.
In addition to using the lock mechanism, we can also use channels to solve the problem of concurrency race conditions. Channels are a mechanism used by the Go language to implement communication between coroutines. Through channels, we can ensure that only one coroutine can access and modify shared resources.
The following is an example of using channels:
package main import ( "fmt" "sync" ) var ( counter int doneChan = make(chan bool) ) func main() { wg := sync.WaitGroup{} for i := 0; i < 100; i++ { wg.Add(1) go func() { defer wg.Done() increment() }() } wg.Wait() <-doneChan fmt.Println("Counter:", counter) } func increment() { counter++ if counter == 100 { doneChan <- true } }
In the above example, we notify the main coroutine that all addition operations have been completed by defining a doneChan channel. In the increment() function, we first add 1 to the counter, and then determine if the counter is equal to 100, and send a true value to the doneChan channel.
Finally, in the main coroutine we use the <-doneChan syntax to wait for and receive the value of the doneChan channel to ensure that all addition operations have been completed.
By using channels, we can avoid direct access to shared resources, but synchronize operations between coroutines through channels, thereby solving the problem of concurrency race conditions.
To sum up, there are many ways to solve the problem of concurrency race conditions in Go language development, including using mutex locks, read-write locks and channels. These methods can effectively solve the problem of concurrency race conditions and improve the concurrency performance of the program. Developers should choose appropriate methods to solve concurrency race conditions based on specific needs to improve program stability and performance.
The above is the detailed content of Methods to solve the problem of concurrency race conditions in Go language development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


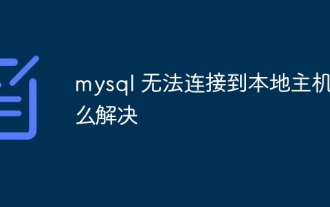
The MySQL connection may be due to the following reasons: MySQL service is not started, the firewall intercepts the connection, the port number is incorrect, the user name or password is incorrect, the listening address in my.cnf is improperly configured, etc. The troubleshooting steps include: 1. Check whether the MySQL service is running; 2. Adjust the firewall settings to allow MySQL to listen to port 3306; 3. Confirm that the port number is consistent with the actual port number; 4. Check whether the user name and password are correct; 5. Make sure the bind-address settings in my.cnf are correct.
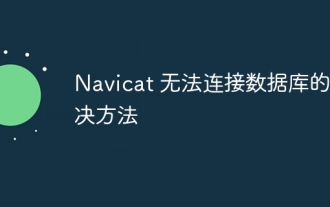
The following steps can be used to resolve the problem that Navicat cannot connect to the database: Check the server connection, make sure the server is running, address and port correctly, and the firewall allows connections. Verify the login information and confirm that the user name, password and permissions are correct. Check network connections and troubleshoot network problems such as router or firewall failures. Disable SSL connections, which may not be supported by some servers. Check the database version to make sure the Navicat version is compatible with the target database. Adjust the connection timeout, and for remote or slower connections, increase the connection timeout timeout. Other workarounds, if the above steps are not working, you can try restarting the software, using a different connection driver, or consulting the database administrator or official Navicat support.
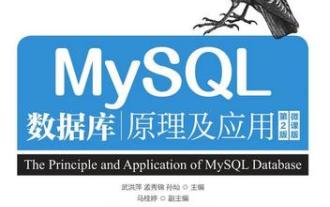
The solution to MySQL installation error is: 1. Carefully check the system environment to ensure that the MySQL dependency library requirements are met. Different operating systems and version requirements are different; 2. Carefully read the error message and take corresponding measures according to prompts (such as missing library files or insufficient permissions), such as installing dependencies or using sudo commands; 3. If necessary, try to install the source code and carefully check the compilation log, but this requires a certain amount of Linux knowledge and experience. The key to ultimately solving the problem is to carefully check the system environment and error information, and refer to the official documents.
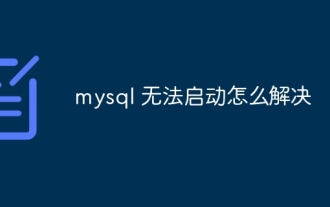
There are many reasons why MySQL startup fails, and it can be diagnosed by checking the error log. Common causes include port conflicts (check port occupancy and modify configuration), permission issues (check service running user permissions), configuration file errors (check parameter settings), data directory corruption (restore data or rebuild table space), InnoDB table space issues (check ibdata1 files), plug-in loading failure (check error log). When solving problems, you should analyze them based on the error log, find the root cause of the problem, and develop the habit of backing up data regularly to prevent and solve problems.
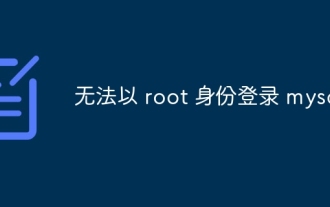
The main reasons why you cannot log in to MySQL as root are permission problems, configuration file errors, password inconsistent, socket file problems, or firewall interception. The solution includes: check whether the bind-address parameter in the configuration file is configured correctly. Check whether the root user permissions have been modified or deleted and reset. Verify that the password is accurate, including case and special characters. Check socket file permission settings and paths. Check that the firewall blocks connections to the MySQL server.
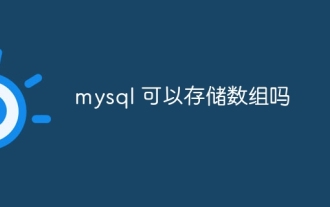
MySQL does not support array types in essence, but can save the country through the following methods: JSON array (constrained performance efficiency); multiple fields (poor scalability); and association tables (most flexible and conform to the design idea of relational databases).
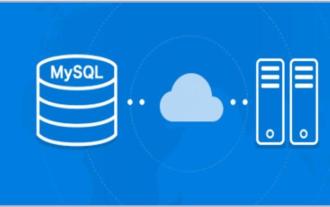
The main reasons for MySQL installation failure are: 1. Permission issues, you need to run as an administrator or use the sudo command; 2. Dependencies are missing, and you need to install relevant development packages; 3. Port conflicts, you need to close the program that occupies port 3306 or modify the configuration file; 4. The installation package is corrupt, you need to download and verify the integrity; 5. The environment variable is incorrectly configured, and the environment variables must be correctly configured according to the operating system. Solve these problems and carefully check each step to successfully install MySQL.
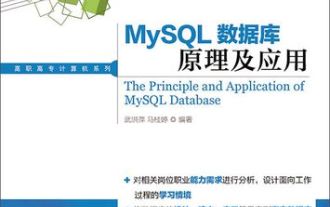
MySQL configuration file corruption can be repaired through the following solutions: 1. Simple fix: If there are only a small number of errors (such as missing semicolons), use a text editor to correct it, and be sure to back up before modifying; 2. Complete reconstruction: If the corruption is serious or the configuration file cannot be found, refer to the official document or copy the default configuration file of the same version, and then modify it according to the needs; 3. Use the installation program to provide repair function: Try to automatically repair the configuration file using the repair function provided by the installer. After selecting the appropriate solution to repair it, you need to restart the MySQL service and verify whether it is successful and develop good backup habits to prevent such problems.
