How to solve URL decoding exceptions in Java development
How to solve URL decoding exceptions in Java development
In Java development, we often encounter situations where we need to decode URLs. However, due to different encoding methods or non-standard URL strings, URL decoding abnormalities sometimes occur. This article will introduce some common URL decoding exceptions and corresponding solutions.
1. Causes of URL decoding exception
- Encoding method mismatch: Special characters in the URL need to be URL encoded, that is, converted into hexadecimal starting with % value. When decoding, you need to use a method that matches the encoding. If the encoding method does not match, a decoding exception will occur.
- URL string format error: The URL may contain special characters, such as spaces, question marks, equal signs, etc. If the URL string does not conform to the URL specification, an exception will occur during decoding.
2. Methods to solve URL decoding exceptions
- Use appropriate URL decoding methods
In Java, a variety of URLs are provided Decoding methods, such as the decode() method of the java.net.URLDecoder class, the getPath() method of the java.net.URI class, etc. Different methods are suitable for different decoding scenarios, and we need to choose the appropriate decoding method according to the specific situation.
- Specify the correct encoding method
There are many URL encoding methods, such as UTF-8, ISO-8859-1, etc. When decoding, you need to explicitly specify the encoding to match the previous encoding. Otherwise, a decoding exception will occur. When using the decode() method of the URLDecoder class, you can specify the encoding method by providing the second parameter, for example: URLDecoder.decode(url, "UTF-8").
- Preprocess the URL string
Before decoding the URL string, we can preprocess it to remove special characters or Some fixes. For example, you can use String's replaceAll() method to replace spaces with and question marks with ?. This ensures that the URL string conforms to the URL specification, thereby avoiding decoding exceptions.
- Use third-party libraries
In addition to Java’s own URL decoding method, there are also some third-party libraries that can help us decode URLs. For example, Apache's HttpClient library provides the URLEncodedUtils class, which can be used for URL decoding and encoding. Using these libraries can simplify our development work and provide more ways to handle decoding exceptions.
3. Summary
In Java development, URL decoding exceptions are one of the more common problems. In order to avoid decoding exceptions, we need to correctly choose the appropriate decoding method, specify the correct encoding method, and preprocess the URL string. In addition, using third-party libraries is also a good way to solve URL decoding exceptions. Through reasonable processing and selection, we can better solve the problem of URL decoding exceptions in Java development and improve the reliability and robustness of the code.
The above is the detailed content of How to solve URL decoding exceptions in Java development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


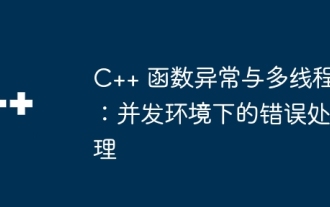
Function exception handling in C++ is particularly important for multi-threaded environments to ensure thread safety and data integrity. The try-catch statement allows you to catch and handle specific types of exceptions when they occur to prevent program crashes or data corruption.
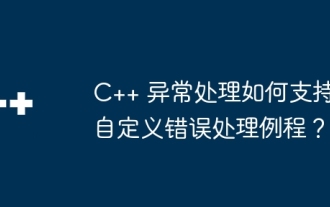
C++ exception handling allows the creation of custom error handling routines to handle runtime errors by throwing exceptions and catching them using try-catch blocks. 1. Create a custom exception class derived from the exception class and override the what() method; 2. Use the throw keyword to throw an exception; 3. Use the try-catch block to catch exceptions and specify the exception types that can be handled.
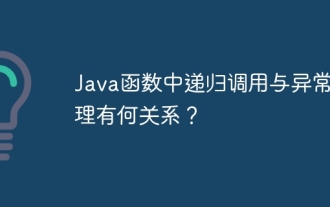
Exception handling in recursive calls: Limiting recursion depth: Preventing stack overflow. Use exception handling: Use try-catch statements to handle exceptions. Tail recursion optimization: avoid stack overflow.
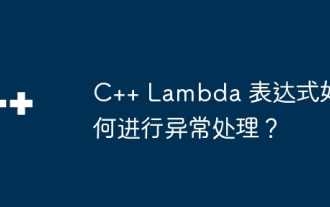
Exception handling in C++ Lambda expressions does not have its own scope, and exceptions are not caught by default. To catch exceptions, you can use Lambda expression catching syntax, which allows a Lambda expression to capture a variable within its definition scope, allowing exception handling in a try-catch block.

In multithreaded C++, exception handling follows the following principles: timeliness, thread safety, and clarity. In practice, you can ensure thread safety of exception handling code by using mutex or atomic variables. Additionally, consider reentrancy, performance, and testing of your exception handling code to ensure it runs safely and efficiently in a multi-threaded environment.
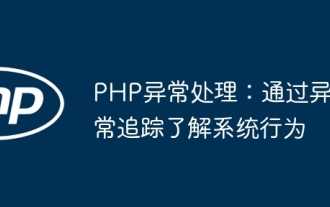
PHP exception handling: Understanding system behavior through exception tracking Exceptions are the mechanism used by PHP to handle errors, and exceptions are handled by exception handlers. The exception class Exception represents general exceptions, while the Throwable class represents all exceptions. Use the throw keyword to throw exceptions and use try...catch statements to define exception handlers. In practical cases, exception handling is used to capture and handle DivisionByZeroError that may be thrown by the calculate() function to ensure that the application can fail gracefully when an error occurs.
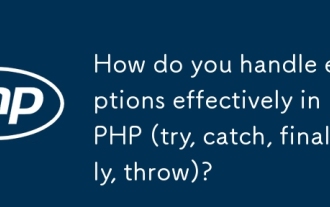
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
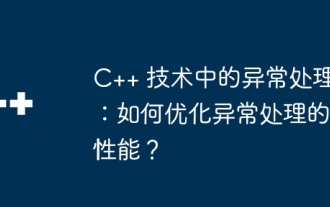
In order to optimize exception handling performance in C++, the following four techniques can be implemented: Avoid unnecessary exception throwing. Use lightweight exception classes. Prioritize efficiency and design exception classes that contain only necessary information. Take advantage of compiler options to achieve the best balance of performance and stability.
