


How to solve the problem of expanding and collapsing multi-level menus in Vue development
How to solve the problem of expanding and collapsing multi-level menus in Vue development
In web application development, it is often necessary to use multi-level menus to display complex data structures and functional modules. However, when designing multi-level menus, a common problem is how to implement the expand and collapse functions of the menu. This article will introduce how to solve the problem of multi-level menu expansion and collapse in Vue development.
1. Requirements Analysis
Before solving the problem, you first need to clarify the requirements. Multi-level menus usually consist of a first-level menu and multi-level submenus. Users can click on the first-level menu to expand or collapse the corresponding submenu. When the user clicks on a first-level menu, its submenus should expand; when the user clicks on the first-level menu again, its submenus should collapse. When only one first-level menu is expanded at the same time, other expanded first-level menus should be collapsed.
2. Solution
Based on the above requirements, we can solve the problem of multi-level menu expansion and collapse through the following steps:
- Data structure design
First, we need to design a suitable data structure to store the status of the menu. Normally, we can use an array to represent the multi-level structure of the menu. Each menu item should contain a unique identifier (such as id), a display name (such as name), and a Boolean value (such as isOpen) to indicate whether the menu item is expanded.
- Component design
Next, we need to design a component to display the menu. This component should include an area for displaying the first-level menu and an area for displaying the submenu. When displaying a first-level menu, you can dynamically generate first-level menu items by traversing the menu data array, and determine whether to display a submenu based on the isOpen property of the menu item.
- Event processing
When the user clicks on the first-level menu, we need to handle the corresponding event to expand or collapse the corresponding submenu. When handling events, we can change the expanded state of the menu item by modifying the isOpen property of the corresponding menu item in the menu data array.
- Style design
Finally, we need to design the corresponding style to display the menu. In styles, you can usually use dynamic class name binding to change the expanded state of a menu item based on its isOpen property.
3. Code Example
The following is a simple Vue code example, showing how to implement the multi-level menu expansion and collapse function:
<template> <div> <div v-for="menu in menus" :key="menu.id" @click="toggleMenu(menu)"> {{ menu.name }} </div> <div v-if="currentMenu && currentMenu.isOpen"> <!-- 展示子菜单 --> </div> </div> </template> <script> export default { data () { return { menus: [ { id: 1, name: '一级菜单1', isOpen: false }, { id: 2, name: '一级菜单2', isOpen: false }, { id: 3, name: '一级菜单3', isOpen: false } ], currentMenu: null } }, methods: { toggleMenu (menu) { if (menu.isOpen) { // 如果菜单已展开,则收起菜单 menu.isOpen = false } else { // 如果菜单未展开,则展开菜单,并关闭其他已展开的菜单 this.menus.forEach(item => { if (item !== menu) { item.isOpen = false } }) menu.isOpen = true } this.currentMenu = menu.isOpen ? menu : null } } } </script> <style> /* 样式省略 */ </style>
Through the above code, we pass Steps such as data structure, component design, event processing and style design solve the problem of expanding and collapsing multi-level menus. By clicking on the first-level menu, we can dynamically expand or collapse the corresponding submenu, and only expand one first-level menu while collapsing other expanded first-level menus.
Conclusion
Through the introduction of this article, we have learned how to solve the problem of multi-level menu expansion and collapse in Vue development. Through appropriate data structure design, component design, event handling and style design, we can implement a fully functional multi-level menu and improve user experience. I hope this article is helpful to you, thank you for reading!
The above is the detailed content of How to solve the problem of expanding and collapsing multi-level menus in Vue development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


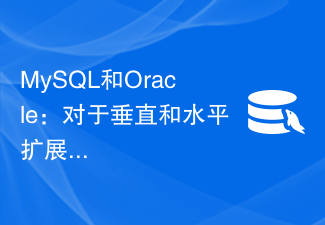
MySQL and Oracle: Vertical and horizontal expansion flexibility comparison In today's big data era, database scalability has become a crucial consideration. Scalability can be divided into two aspects: vertical expansion and horizontal expansion. In this article, we will focus on comparing the flexibility of two common relational databases, MySQL and Oracle, in terms of vertical and horizontal expansion. Vertical expansion Vertical expansion improves database performance by increasing the processing power of the server. This can be achieved by adding more CPU cores and expanding memory capacity
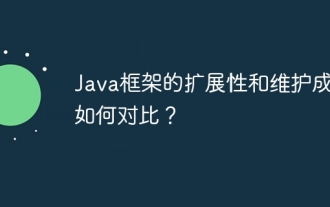
When choosing a Java framework, Spring Framework is known for its high scalability, but as complexity increases, maintenance costs also increase. In contrast, Dropwizard is generally less expensive to maintain but less scalable. Developers should evaluate frameworks based on specific needs.
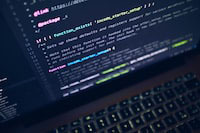
In modern software development, creating scalable, maintainable applications is crucial. PHP design patterns provide a set of proven best practices that help developers achieve code reuse and increase scalability, thereby reducing complexity and development time. What are PHP design patterns? Design patterns are reusable programming solutions to common software design problems. They provide a unified and common way to organize and structure code, thereby promoting code reuse, extensibility, and maintainability. SOLID Principles The PHP design pattern follows the SOLID principles: S (Single Responsibility): Each class or function should be responsible for a single responsibility. O (Open-Closed): The class should be open for extension, but closed for modification. L (Liskov replacement): subclasses should
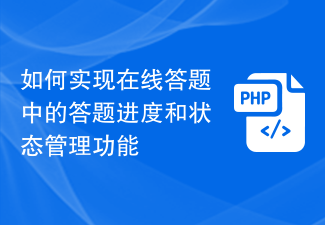
How to implement the answering progress and status management functions in online answering requires specific code examples. When developing an online answering system, answering progress and status management are one of the very important functions. By properly designing and implementing the answering progress and status management functions, it can help users understand their own answering progress and improve user experience and user participation. The following will introduce how to implement the answering progress and status management functions in online answering, and provide specific code examples. 1. Implementation of the question-answering progress management function In online question-answering, question-answering progress management refers to the user’s
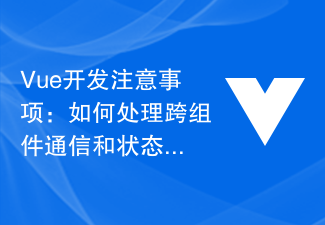
Vue is a popular JavaScript framework that allows us to build interactive and powerful web applications. In Vue development, cross-component communication and state management are two important concepts. This article will introduce some precautions for Vue development to help developers better deal with these two aspects. 1. Cross-component communication In Vue development, cross-component communication is a common requirement. When we need to share data or communicate between different components, we can use the following ways to achieve it: Props and
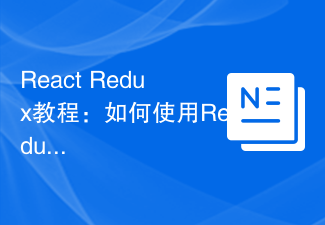
ReactRedux Tutorial: How to Manage Front-End State with Redux React is a very popular JavaScript library for building user interfaces. And Redux is a JavaScript library for managing application state. Together they help us better manage front-end state. This article will introduce how to use Redux to manage state in React applications and provide specific code examples. 1. Install and set up Redux First, we need to install Re
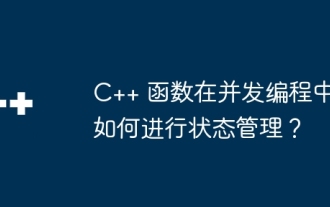
Common techniques for managing function state in C++ concurrent programming include: Thread-local storage (TLS) allows each thread to maintain its own independent copy of variables. Atomic variables allow atomic reading and writing of shared variables in a multi-threaded environment. Mutexes ensure state consistency by preventing multiple threads from executing critical sections at the same time.
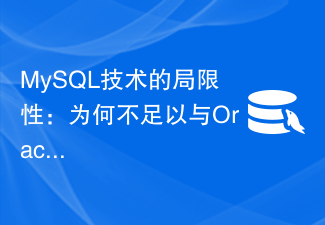
The limitations of MySQL technology: Why is it not enough to compete with Oracle? Introduction: MySQL and Oracle are one of the most popular relational database management systems (RDBMS) in the world today. While MySQL is very popular in web application development and small businesses, Oracle has always dominated the world of large enterprises and complex data processing. This article will explore the limitations of MySQL technology and explain why it is not enough to compete with Oracle. 1. Performance and scalability limitations: MySQL is
