How to optimize string search and replace performance in Java development
In Java development, string search and replacement is a very common operation. In many cases, we need to locate a specific substring in a large text and perform replacement operations. The search and replacement performance of strings often has a greater impact on the overall performance of the program. This article will introduce some optimization strategies to help developers improve the performance of string search and replacement.
- Use the indexOf() function for string search
Java provides the indexOf() function to locate the position of a certain substring in a string. When performing multiple searches, we can usually use this function to search and record the position of each match. This approach can be implemented by looping, thus performing multiple searches. This method is more efficient than using regular expressions to search. - Use StringBuilder to replace strings
The String class in Java is immutable. Every time string splicing and replacement operations are performed, a new String object will be created. This results in frequent object creation and garbage collection, which affects performance. In order to solve this problem, we can use the StringBuilder class to implement string replacement. StringBuilder is mutable, and each operation is performed on the original object, thus avoiding the frequent creation and destruction of objects. - Use regular expressions for string replacement
In some cases, we may need to replace substrings in the string that match specific patterns. In this case, consider using regular expressions to find and replace. Java provides Pattern and Matcher to support regular expression operations. Using regular expressions can simplify your code, but performance may suffer due to the complexity of regular expressions. Therefore, when using regular expressions for string replacement, the performance impact needs to be carefully evaluated. - Using the string pool
In Java, the string pool is a memory area used to store strings. When we create a string, we first check if a string with the same content exists in the string pool. If it exists, the reference is returned directly; if it does not exist, the string is added to the string pool and the reference is returned. Using a string pool avoids creating multiple string objects with the same content, thereby saving memory and improving performance. - Use Boyer-Moore algorithm for string search
Boyer-Moore algorithm is an efficient string search algorithm. It takes advantage of the mismatch information between the target string and the pattern string to minimize the number of comparisons. In string search operations, using the Boyer-Moore algorithm can greatly improve performance. Java provides the indexOf() method of the String class, and the underlying Boyer-Moore algorithm is used to implement string search. - Avoid unnecessary string splicing and copying
When we splice and copy strings, new string objects are often created. In scenarios with high performance requirements, we should try to avoid unnecessary string concatenation and copying operations. You can use StringBuilder or StringBuffer instead of String to perform string splicing operations. Additionally, you can use the substring() method of the String class to obtain a substring of a string instead of concatenating and copying it.
Summary:
In Java development, optimizing string search and replacement performance is an important issue. By using optimization strategies such as indexOf(), StringBuilder, regular expressions, and string pools, the performance of string search and replacement can be effectively improved. In addition, you can choose a suitable search algorithm according to the specific scenario, such as the Boyer-Moore algorithm. In actual development, we should reasonably select and use these optimization strategies based on the needs and performance requirements of the code to achieve the best performance and user experience.
The above is the detailed content of How to optimize string search and replace performance in Java development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


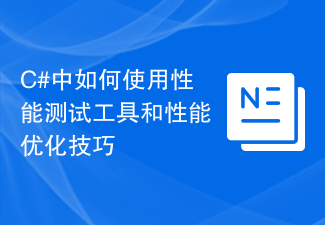
How to use performance testing tools and performance optimization techniques in C# requires specific code examples. Performance optimization plays a very important role in the software development process. It can improve the performance, running speed and responsiveness of the system. C# is a high-performance programming language, and there are many performance optimization techniques and tools that can help us take better advantage of C#. This article will introduce how to use performance testing tools and provide some common performance optimization tips and sample code. Use performance testing tools Performance testing tools can help us evaluate the performance of our code and
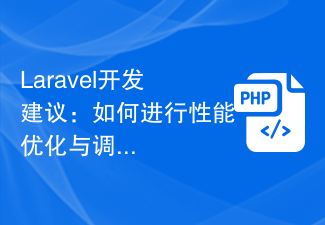
Laravel development suggestions: How to perform performance optimization and debugging Introduction: Laravel is an excellent PHP development framework that is loved by developers for its simplicity, efficiency and ease of use. However, when an application encounters a performance bottleneck, we need to perform performance optimization and debugging to improve user experience. This article will introduce some practical tips and suggestions to help developers optimize and debug the performance of Laravel applications. 1. Performance optimization: Database query optimization: Reducing the number of database queries is the key to performance optimization.
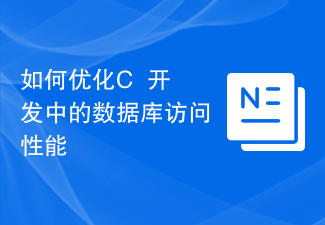
How to optimize database access performance in C++ development Database is an indispensable part of modern software development, and in C++ development, data storage and access through databases are very common requirements. However, for large-scale data operations or complex queries, database access may become a performance bottleneck. In order to improve the running efficiency and response speed of the program, we need to optimize database access performance. This article will introduce some common methods and techniques to help us better optimize database access performance in C++ development. Database Design
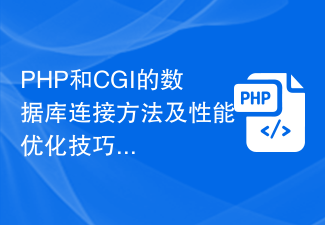
Database connection methods and performance optimization techniques for PHP and CGI In the website development process, the database is a very important component. PHP is a very popular website development language, while CGI is a general web page generation program. This article will introduce database connection methods in PHP and CGI as well as some performance optimization techniques. Database connection methods in PHP PHP provides a variety of methods to connect to the database, the most commonly used of which is to use MySQLi and PDO extensions. a. Extend MySQL using MySQLi
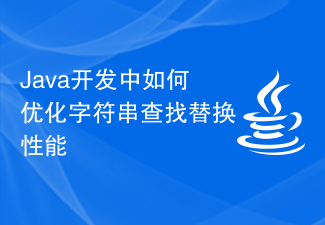
In Java development, string search and replacement is a very common operation. In many cases, we need to locate a specific substring in a large text and perform replacement operations. The search and replacement performance of strings often has a greater impact on the overall performance of the program. This article will introduce some optimization strategies to help developers improve the performance of string search and replacement. Use the indexOf() function to search for strings. Java provides the indexOf() function to locate a substring in a string.
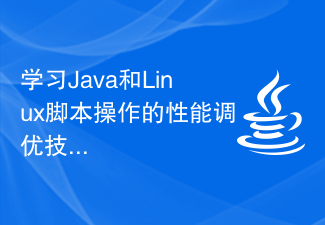
Learn performance tuning skills for Java and Linux script operations Introduction: In today's software development field, performance tuning is an important topic. Whether you are developing Java programs or writing Linux scripts, you need to focus on how to improve the efficiency and performance of your code. This article will introduce some performance tuning techniques for Java and Linux script operations and provide specific code examples. 1. Java performance tuning skills. Use appropriate data structures and algorithms. When writing Java programs, you must choose appropriate data structures and algorithms.
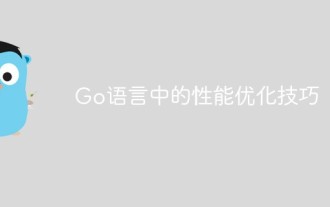
As modern software systems become more complex, performance issues become more critical. Performance optimization is an important but not easy task, involving many aspects and requiring comprehensive consideration. In this article, we will focus on some performance optimization techniques in the Go language to help developers better solve performance problems. Writing Efficient Code When writing code, performance should always be considered. Generally speaking, efficient code has the following characteristics: (1) Simple and clear. Don't write overly complex code, as this will make the code difficult to maintain and debug. (
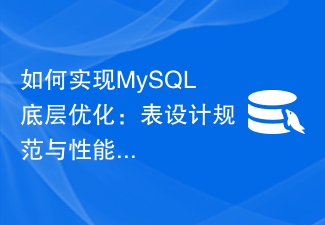
How to realize MySQL underlying optimization: table design specifications and performance optimization techniques. In database management systems, MySQL is a commonly used relational database. During the development process, it is crucial to properly design the database table structure and optimize database performance. This article will introduce how to implement MySQL underlying optimization from two aspects: table design specifications and performance optimization techniques, and provide specific code examples. 1. Table design specifications 1. Choose the appropriate data type When designing the table structure, you should choose the appropriate data type according to actual needs. For example, for
