How to solve memory allocation problem in Go language
Methods to solve memory allocation problems in Go language development
In the development of Go language, memory allocation problems are one of the challenges that developers often face. The Go language is known for its efficient garbage collection mechanism and built-in concurrency features. However, incorrect memory allocation and usage can lead to problems such as performance degradation and memory leaks. This article will explore several methods to solve memory allocation problems in Go language development.
Method 1: Avoid excessive use of large objects
In the Go language, the creation and destruction of large objects will take up more memory and time. Therefore, avoiding excessive use of large objects is an effective way to solve memory allocation problems. This can be achieved in the following ways:
- Use object pooling: Object pooling is a common technique that allows you to reuse already created objects instead of frequently creating new objects. Through the object pool, the number of memory allocations can be effectively reduced and performance improved.
- Use buffering: Go language provides buffered data structures, such as buffer channels and cache byte slices. By using buffering, you can avoid the frequent creation and destruction of large objects, thereby reducing the overhead of memory allocation.
Method 2: Reasonable use of pointers
Pointers are one of the most powerful features in the Go language, but they can also easily lead to memory allocation problems. When using pointers, you need to pay special attention to the following points:
- Avoid pointer escape: Pointer escape means that the object pointed to by the pointer is still referenced by other parts after the function returns. When an object is referenced, the garbage collector cannot release the memory it occupies, resulting in a memory leak. Therefore, during the development process, try to avoid pointer escapes.
- Reasonable use of pointer passing: When passing parameters in a function, using pointer passing can avoid object copying and reduce the cost of memory allocation. However, misuse of pointer passing can lead to unnecessary memory allocations. Therefore, when using pointer passing, you need to weigh the pros and cons and choose the appropriate method.
Method 3: Reduce unnecessary memory allocation
Unnecessary memory allocation is one of the common problems in Go language development. The following aspects are ways to reduce unnecessary memory allocation:
- Reuse variables: During loops or iterations, try to avoid frequently creating and destroying variables. You can reduce the memory allocation overhead by defining variables outside the loop or using variable pools to reuse variables.
- Avoid slice expansion: When using a slice, if its capacity is known in advance, you can reduce slice expansion operations by specifying capacity parameters. The process of slicing expansion will cause memory allocation overhead, so try to avoid unnecessary slicing expansion.
Method 4: Proper use of concurrency control
The Go language is known for its excellent concurrency features. However, incorrect concurrency control may cause memory allocation problems. The following aspects are ways to use concurrency control reasonably:
- Use sync.Pool: sync.Pool is an object pool in the Go language, which can be used to reuse objects and reduce the cost of object creation and destruction. overhead. Correct use of sync.Pool can effectively reduce the number of memory allocations.
- Use appropriate locks: In concurrent programming, using appropriate locks can protect access to shared resources and reduce the occurrence of race conditions. Avoiding unnecessary lock contention can reduce memory allocation overhead.
Summary:
To solve the memory allocation problem in Go language development, developers need to pay attention to it in code writing and performance optimization. Methods such as avoiding excessive use of large objects, rational use of pointers, reducing unnecessary memory allocation and rational use of concurrency control can help us solve memory allocation problems and optimize program performance. I hope this article can provide some help and inspiration for readers on memory allocation problems encountered in Go language development.
The above is the detailed content of How to solve memory allocation problem in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


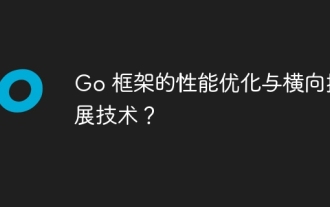
In order to improve the performance of Go applications, we can take the following optimization measures: Caching: Use caching to reduce the number of accesses to the underlying storage and improve performance. Concurrency: Use goroutines and channels to execute lengthy tasks in parallel. Memory Management: Manually manage memory (using the unsafe package) to further optimize performance. To scale out an application we can implement the following techniques: Horizontal Scaling (Horizontal Scaling): Deploying application instances on multiple servers or nodes. Load balancing: Use a load balancer to distribute requests to multiple application instances. Data sharding: Distribute large data sets across multiple databases or storage nodes to improve query performance and scalability.

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
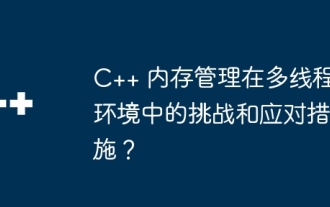
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.
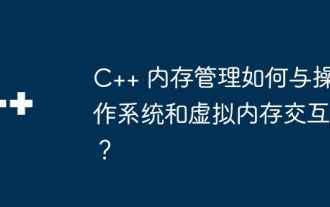
C++ memory management interacts with the operating system, manages physical memory and virtual memory through the operating system, and efficiently allocates and releases memory for programs. The operating system divides physical memory into pages and pulls in the pages requested by the application from virtual memory as needed. C++ uses the new and delete operators to allocate and release memory, requesting memory pages from the operating system and returning them respectively. When the operating system frees physical memory, it swaps less used memory pages into virtual memory.
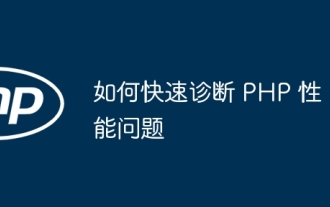
Effective techniques for quickly diagnosing PHP performance issues include using Xdebug to obtain performance data and then analyzing the Cachegrind output. Use Blackfire to view request traces and generate performance reports. Examine database queries to identify inefficient queries. Analyze memory usage, view memory allocations and peak usage.
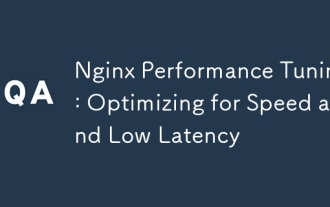
Nginx performance tuning can be achieved by adjusting the number of worker processes, connection pool size, enabling Gzip compression and HTTP/2 protocols, and using cache and load balancing. 1. Adjust the number of worker processes and connection pool size: worker_processesauto; events{worker_connections1024;}. 2. Enable Gzip compression and HTTP/2 protocol: http{gzipon;server{listen443sslhttp2;}}. 3. Use cache optimization: http{proxy_cache_path/path/to/cachelevels=1:2k
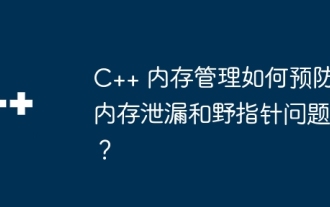
When it comes to memory management in C++, there are two common errors: memory leaks and wild pointers. Methods to solve these problems include: using smart pointers (such as std::unique_ptr and std::shared_ptr) to automatically release memory that is no longer used; following the RAII principle to ensure that resources are released when the object goes out of scope; initializing the pointer and accessing only Valid memory, with array bounds checking; always use the delete keyword to release dynamically allocated memory that is no longer needed.
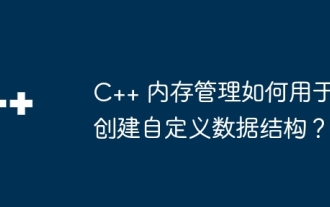
Memory management in C++ allows the creation of custom data structures. Dynamic memory allocation uses the new and delete operators to allocate and free memory at runtime. Custom data structures can be created using dynamic memory allocation, such as a linked list, where the Node structure stores a pointer and data to the next node. In the actual case, the linked list is created using dynamic memory allocation, stores integers and traverses the printing data, and finally releases the memory.
