PHP password encryption and secure storage method
With the continuous development and popularization of the Internet, the security of user passwords has attracted increasing attention when performing various operations online. As a commonly used server-side programming language, PHP also has its own set of implementation methods for password encryption and secure storage. This article will introduce how PHP encrypts and secures passwords, helping readers understand and protect their user passwords.
1. Password encryption
Password encryption refers to converting user passwords to generate a seemingly random sequence of characters. Even if a hacker obtains this character sequence, it cannot be restored to the original password. Common password encryption algorithms include MD5, SHA1 and bcrypt.
- Traditional encryption algorithms - MD5 and SHA1
MD5 and SHA1 are one of the most common password encryption algorithms. In PHP, you can use the md5() and sha1() functions for password encryption. However, these algorithms are increasingly at risk of being cracked by hackers because they are one-way encryption and cannot be decrypted back to the original password. Therefore, it is not recommended to use MD5 and SHA1 for password encryption.
Sample code:
$password = '123456';
$encrypted_password = md5($password);
echo $encrypted_password; // Output: e10adc3949ba59abbe56e057f20f883e
- Secure password encryption algorithm-bcrypt
bcrypt is a safe and reliable password encryption algorithm, which is based on the Blowfish encryption algorithm. In PHP, you can use the password_hash() function for password encryption. It is a method supported by PHP 5.5.0 and above.
Sample code:
$password = '123456';
$encrypted_password = password_hash($password, PASSWORD_DEFAULT);
echo $encrypted_password; // Output: $2y$10$IwyKg5WIr0ZG6oWjbja0zuxw8FdW8GmKyMzykluZfoyvG00v4OMu6
This function will automatically generate a random salt value and mix the salt value with the password for encryption. Each execution will produce a different encryption result to increase password security.
2. Secure storage
Password encryption is only part of password protection. In order to better protect user passwords, secure storage is also required. Here are a few common safe storage methods.
- Database Storage
In most cases, user passwords are stored in the database. Before storing passwords in the database, they should be encrypted using the password encryption algorithm described above. Usually, the data type of the password field is varchar, and the appropriate length is set.
- Salt value storage
In order to increase the security of the password, the "salt" method can be used to store the encrypted password in a more complex manner. The salt value is a random string that is mixed with the password and then encrypted and stored, so that even with the same password, the ciphertext stored by different users is different.
Sample code:
$password = '123456';
$salt = 'AbCdEfGh';
$encrypted_password = md5($password.$salt);
echo $encrypted_password ; // Output: a62d15e1e7be18bc451f780ff573a4e1
- Encrypted connection storage
In addition to database storage and salt value storage, other encryption methods can also be used to store user passwords, such as RSA encryption. RSA is an asymmetric encryption algorithm that generates both public and private keys. The user password is first encrypted with the public key and then decrypted by the server's private key.
Sample code:
$password = '123456';
$public_key = 'public_key_path.pem';
$private_key = 'private_key_path.pem';
$ encrypted_password = '';
openssl_public_encrypt($password, $encrypted_password, file_get_contents($public_key));
file_put_contents('encrypted_password.txt', $encrypted_password);
$decrypted_password = '';
openssl_private_decrypt(file_get_contents('encrypted_password.txt'), $decrypted_password, file_get_contents($private_key));
echo $decrypted_password; // Output: 123456
The above is PHP for password encryption and security Some basic methods of storage. In order to better protect user passwords, it is recommended to use safe and reliable encryption algorithms and adopt appropriate storage methods. In addition, attention should also be paid to preventing common security vulnerabilities, such as SQL injection, XSS attacks, etc., to ensure the security of user passwords.
The above is the detailed content of PHP password encryption and secure storage method. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


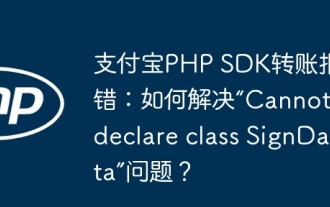
Alipay PHP...
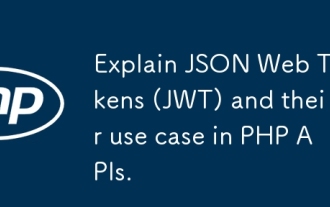
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
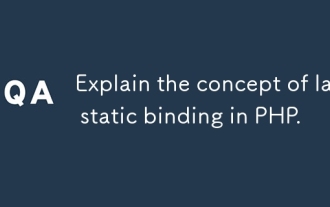
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
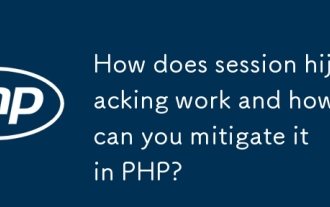
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
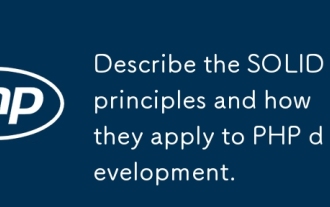
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
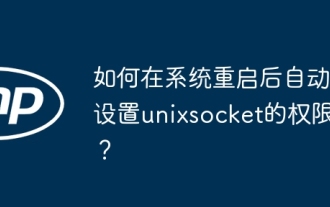
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
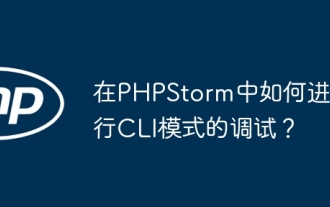
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
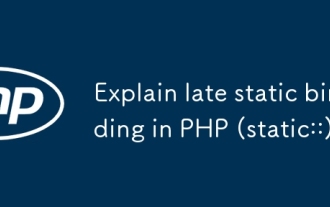
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
