PHP optimizes MySQL connections
How to optimize MySQL connection in PHP program?
MySQL is one of the most commonly used open source databases, and it is very common to use MySQL in PHP applications. However, as the amount of data increases and the number of concurrent users increases, the performance issues of MySQL connections may become a serious bottleneck. Therefore, optimizing MySQL connections is crucial to improve the performance of your application. This article will introduce some methods to optimize MySQL connections in PHP programs.
- Reduce the number of connections: Each time you establish a connection to the database, additional overhead will be incurred, including network overhead and database server resource overhead. Therefore, minimizing the number of unnecessary connections is the key to improving performance. A common approach is to use persistent connections, either using
mysql_pconnect
or using thePDO::ATTR_PERSISTENT
option in the PDO extension. This allows you to use a connection pool to share connections and reduce the cost of connection establishment. - Merge query: When a page requires multiple database queries, these queries can be merged into one to reduce unnecessary database requests. This reduces communication overhead with the database and reduces server resource usage. It is a common practice to combine multiple queries into a single query using the
UNION
operator orJOIN
. - Use indexes: Indexes only exist in database tables, but they can greatly improve query performance. When using a MySQL database, it is a common optimization method to create indexes for the primary keys and commonly used query fields of database tables. In a PHP program, you can use the
CREATE INDEX
statement or use a database management tool to create an index. - Limit the size of the result set: In some cases, it is not necessary to return all query results, only some results need to be returned. You can use the
LIMIT
keyword to limit the number of rows returned. This can reduce the size of the returned results and reduce network transmission overhead. - Cache query results: For some data that is frequently queried and does not change frequently, the query results can be cached. This can reduce the load on the database and speed up queries. PHP caching extensions such as Memcached or Redis can be used to implement caching.
- Use batch operations: When you need to insert a large amount of data or update a large amount of data, you can use batch operations to improve performance. Compared with executing SQL statements one by one, batch operations can reduce communication overhead and database operation overhead. In PHP programs, you can use the
INSERT INTO ... VALUES (...)
syntax to insert multiple rows of data at one time. - Disable automatic submission: In some cases, if automatic submission is performed every time a SQL statement is executed, unnecessary IO overhead and locking operations will occur. Autocommit can be disabled by setting the
autocommit
option, and then committed manually when appropriate. - Optimize the query statement: The performance of the query statement depends on its cost, including the complexity of the query, the indexes used and the size of the data. In terms of optimizing query statements, you can use the
EXPLAIN
syntax to analyze the query execution plan, understand the bottleneck of query performance, and then optimize accordingly.
To summarize, optimizing MySQL connections can significantly improve the performance of PHP applications. We can achieve this goal by reducing the number of joins, merging queries, using indexes, limiting result set size, caching query results, using batch operations, disabling automatic submission and optimizing query statements. At the same time, developers should also perform performance tuning according to specific application scenarios and constantly look for better optimization methods.
The above is the detailed content of PHP optimizes MySQL connections. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




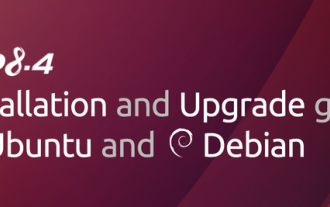
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
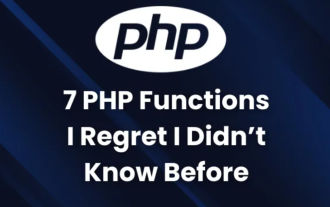
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
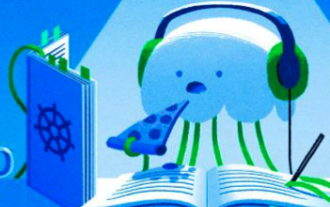
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
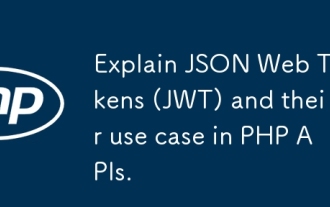
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
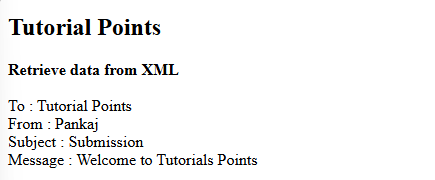
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
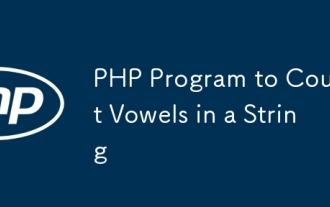
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
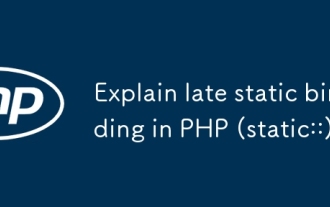
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
