How to optimize Java collection sorting performance
Java is a powerful programming language that is widely used in various types of software development. In Java development, scenarios that often involve sorting collections are involved. However, if performance optimization is not performed for collection sorting, the execution efficiency of the program may decrease. This article will explore how to optimize the performance of Java collection sorting.
1. Choose the appropriate collection class
In Java, there are many collection classes that can be used for sorting, such as ArrayList, LinkedList, TreeSet, etc. Different collection classes have different performance during the sorting process. In order to select the collection class with the best performance, you can evaluate and select based on specific needs and scenarios. For example, if you perform frequent insertion and deletion operations on the collection, you can choose LinkedList; if you perform frequent query operations on the collection, you can choose ArrayList. For scenarios that require frequent sorting of sets, you can choose TreeSet because TreeSet uses a red-black tree data structure internally and has faster sorting performance.
2. Use a custom comparator
In Java, the default comparison rules can be used for sorting collections, or a custom comparator can be used. If you use the default comparison rules, Java sorts the collection elements according to their natural order. However, sometimes the natural order does not meet the needs and needs to be sorted according to custom rules. In order to optimize sorting performance, you can implement a custom comparator and define sorting rules by overriding the compare method. Custom comparators can be optimized according to specific needs to avoid unnecessary comparison operations, thereby improving performance.
3. Use parallel sorting
Java 8 introduces the concept of parallel streams, through which the sorting process of collections can be parallelized. Parallel sorting can make full use of the advantages of multi-core processors to speed up sorting. When there are many elements in the collection and it takes a long time to sort, you can consider using parallel sorting. The code example for sorting using parallel streams is as follows:
List
list.parallelStream()
.sorted() .forEach(System.out::println);
4. Avoid frequently creating collection objects
When using collections for sorting, you need to pay attention to avoid frequently creating collection objects. If a new collection object is created every time it is sorted, it will increase the memory overhead and the burden of garbage collection, and reduce the performance of the program. To avoid this situation, you can clear the collection before sorting, and then use the existing collection object for sorting. Examples are as follows:
List
Collections.sort(list);
list.forEach(System.out: :println);
5. Reasonable use of cache
In scenarios where the same collection needs to be sorted multiple times, you can consider using cache to improve performance. The cache can store the sorting results and use the results in the cache directly the next time you sort to avoid repeated sorting operations. In order to implement the caching function, you can use some caching frameworks, such as Guava Cache or Ehcache.
6. Try to avoid using recursive sorting
Recursive sorting is a commonly used sorting algorithm, but recursive calls will bring additional overhead and occupy more memory and processor resources. In order to optimize performance, it is best to avoid using recursive sorting and consider using iterative sorting algorithms, such as quick sort or merge sort.
Summary:
In Java development, sorting collections is a common and important task. In order to optimize the performance of collection sorting, you can choose an appropriate collection class, use custom comparators, use parallel sorting, avoid frequently creating collection objects, use cache rationally, and try to avoid using recursive sorting. Through these optimization measures, the efficiency of Java collection sorting can be improved and the execution speed of the program can be improved. Ultimately achieving efficient and stable software development.
The above is the detailed content of How to optimize Java collection sorting performance. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
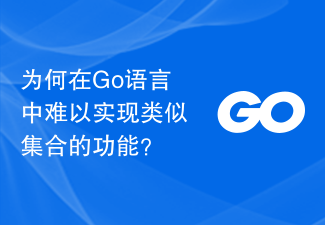
It is difficult to implement collection-like functions in the Go language, which is a problem that troubles many developers. Compared with other programming languages such as Python or Java, the Go language does not have built-in collection types, such as set, map, etc., which brings some challenges to developers when implementing collection functions. First, let's take a look at why it is difficult to implement collection-like functionality directly in the Go language. In the Go language, the most commonly used data structures are slice and map. They can complete collection-like functions, but
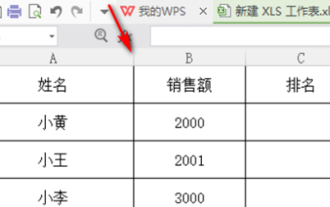
In our work, we often use wps software. There are many ways to process data in wps software, and the functions are also very powerful. We often use functions to find averages, summaries, etc. It can be said that as long as The methods that can be used for statistical data have been prepared for everyone in the WPS software library. Below we will introduce the steps of how to sort the scores in WPS. After reading this, you can learn from the experience. 1. First open the table that needs to be ranked. As shown below. 2. Then enter the formula =rank(B2, B2: B5, 0), and be sure to enter 0. As shown below. 3. After entering the formula, press the F4 key on the computer keyboard. This step is to change the relative reference into an absolute reference.
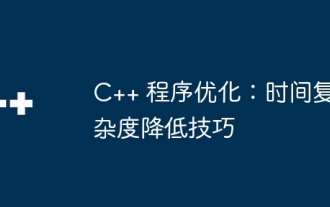
Time complexity measures the execution time of an algorithm relative to the size of the input. Tips for reducing the time complexity of C++ programs include: choosing appropriate containers (such as vector, list) to optimize data storage and management. Utilize efficient algorithms such as quick sort to reduce computation time. Eliminate multiple operations to reduce double counting. Use conditional branches to avoid unnecessary calculations. Optimize linear search by using faster algorithms such as binary search.
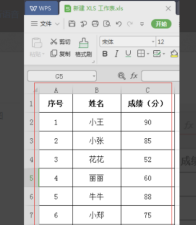
WPS is a very complete office software, including text editing, data tables, PPT presentations, PDF formats, flow charts and other functions. Among them, the ones we use most are text, tables, and demonstrations, and they are also the ones we are most familiar with. In our study work, we sometimes use WPS tables to make some data statistics. For example, the school will count the scores of each student. If we have to manually sort the scores of so many students, it will be really a headache. In fact, we don’t have to worry, because our WPS table has a sorting function to solve this problem for us. Next, let’s learn how to sort WPS together. Method steps: Step 1: First we need to open the WPS table that needs to be sorted
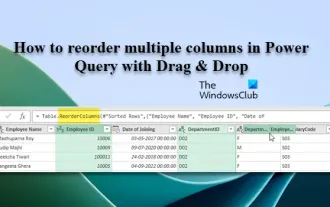
In this article, we will show you how to reorder multiple columns in PowerQuery by dragging and dropping. Often, when importing data from various sources, columns may not be in the desired order. Reordering columns not only allows you to arrange them in a logical order that suits your analysis or reporting needs, it also improves the readability of your data and speeds up tasks such as filtering, sorting, and performing calculations. How to rearrange multiple columns in Excel? There are many ways to rearrange columns in Excel. You can simply select the column header and drag it to the desired location. However, this approach can become cumbersome when dealing with large tables with many columns. To rearrange columns more efficiently, you can use the enhanced query editor. Enhancing the query
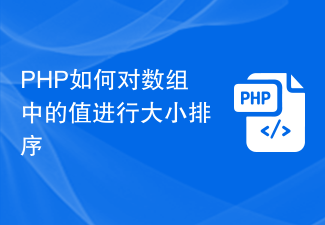
PHP is a commonly used server-side scripting language widely used in website development and data processing fields. In PHP, it is a very common requirement to sort the values in an array by size. By using the built-in sort function, you can easily sort arrays. The following will introduce how to use PHP to sort the values in an array by size, with specific code examples: 1. Sort the values in the array in ascending order:
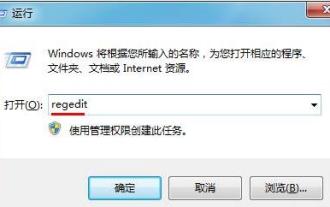
1. Press the key combination (win key + R) on the desktop to open the run window, then enter [regedit] and press Enter to confirm. 2. After opening the Registry Editor, we click to expand [HKEY_CURRENT_USERSoftwareMicrosoftWindowsCurrentVersionExplorer], and then see if there is a Serialize item in the directory. If not, we can right-click Explorer, create a new item, and name it Serialize. 3. Then click Serialize, then right-click the blank space in the right pane, create a new DWORD (32) bit value, and name it Star

Vivox100s parameter configuration revealed: How to optimize processor performance? In today's era of rapid technological development, smartphones have become an indispensable part of our daily lives. As an important part of a smartphone, the performance optimization of the processor is directly related to the user experience of the mobile phone. As a high-profile smartphone, Vivox100s's parameter configuration has attracted much attention, especially the optimization of processor performance has attracted much attention from users. As the "brain" of the mobile phone, the processor directly affects the running speed of the mobile phone.
