


How does PHP handle uploading and processing of multimedia files?
How does PHP handle multimedia file uploading and processing?
With the development of the Internet, the use of multimedia files is becoming more and more common. Whether it is pictures, audio or video files, they are all things we often need to deal with when developing websites. This article will introduce how PHP handles the uploading and processing of multimedia files.
- Multimedia file upload
PHP provides some functions to handle the upload of multimedia files. Commonly used functions are:
-
move_uploaded_file()
: Move the uploaded file to the specified directory. You can use this function to move files from a temporary directory to somewhere to save them.
The sample code is as follows:
<?php $targetDir = "uploads/"; $targetFile = $targetDir . basename($_FILES["file"]["name"]); $uploadOk = 1; // 检查文件是否已存在 if (file_exists($targetFile)) { echo "文件已存在。"; $uploadOk = 0; } // 检查文件大小 if ($_FILES["file"]["size"] > 500000) { echo "文件太大。"; $uploadOk = 0; } // 允许上传的文件类型 $allowedTypes = array("jpg", "png", "jpeg", "gif"); $fileType = strtolower(pathinfo($targetFile, PATHINFO_EXTENSION)); if (!in_array($fileType, $allowedTypes)) { echo "只允许上传图片文件。"; $uploadOk = 0; } // 检查上传是否成功 if ($uploadOk == 0) { echo "文件上传失败。"; } else { if (move_uploaded_file($_FILES["file"]["tmp_name"], $targetFile)) { echo "文件上传成功。"; } else { echo "文件上传失败。"; } } ?>
- Multimedia file processing
PHP provides a wealth of extensions and libraries to process multimedia files. The following are some commonly used processing techniques and functions:
- Image processing: Use
GD library
orImagick extension
to process image files, such as scaling, cropping, Add watermarks and other operations.
The sample code is as follows:
<?php // 使用GD库进行图片缩放 $sourceFile = "uploads/image.jpg"; $targetFile = "uploads/resized_image.jpg"; list($width, $height) = getimagesize($sourceFile); $aspectRatio = $width / $height; $targetWidth = 200; $targetHeight = 200 / $aspectRatio; $newImage = imagecreatetruecolor($targetWidth, $targetHeight); $sourceImage = imagecreatefromjpeg($sourceFile); imagecopyresampled($newImage, $sourceImage, 0, 0, 0, 0, $targetWidth, $targetHeight, $width, $height); imagejpeg($newImage, $targetFile, 90); imagedestroy($newImage); imagedestroy($sourceImage); ?>
- Audio processing: Use
FFmpeg
to process audio files, such as transcoding, editing, merging and other operations.
The sample code is as follows:
<?php // 使用FFmpeg将音频文件转换为MP3格式 $sourceFile = "uploads/audio.wav"; $targetFile = "uploads/converted_audio.mp3"; exec("ffmpeg -i " . $sourceFile . " -vn -ar 44100 -ac 2 -b:a 192k " . $targetFile); ?>
- Video processing: Use
FFmpeg
to process video files, such as transcoding, editing, screenshots, etc.
The sample code is as follows:
<?php // 使用FFmpeg将视频文件转换为MP4格式 $sourceFile = "uploads/video.mov"; $targetFile = "uploads/converted_video.mp4"; exec("ffmpeg -i " . $sourceFile . " -vcodec h264 -acodec aac " . $targetFile); ?>
Summary:
PHP provides a wealth of functions, extensions and libraries to handle the uploading and processing of multimedia files. By using these tools, we can easily operate multimedia files and achieve richer and more diverse website functions. Developers can choose appropriate technologies and tools to process multimedia files based on specific needs.
The above is the detailed content of How does PHP handle uploading and processing of multimedia files?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


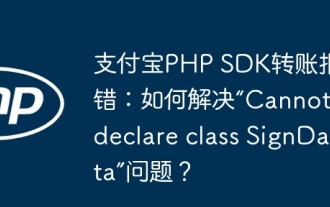
Alipay PHP...
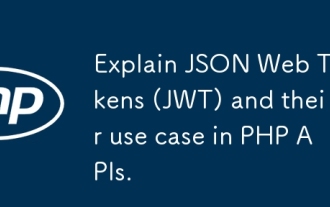
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
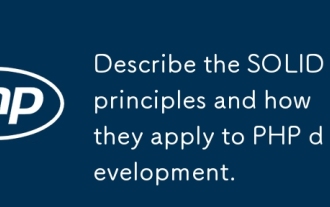
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
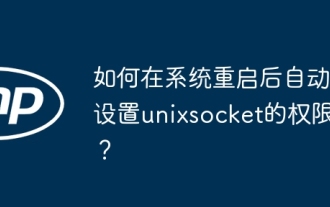
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
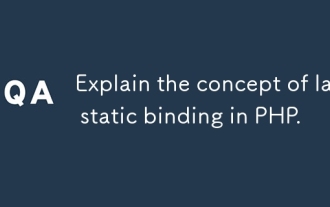
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
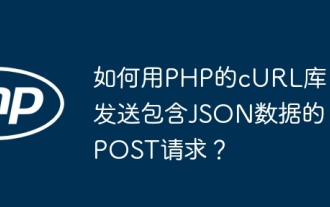
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
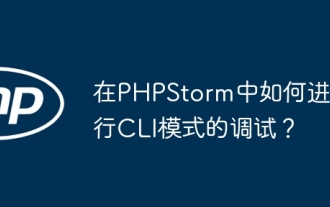
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
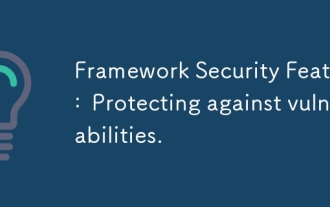
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
