Java deals with XML parsing exceptions
How to solve XML parsing exceptions in Java development
Abstract: XML (Extensible Markup Language) is a commonly used data exchange format. In Java development, we often need to parse XML files. However, you may encounter various exceptions when parsing XML files. This article will introduce how to solve common XML parsing exceptions in Java development.
1. Types of XML parsing exceptions
In Java development, common XML parsing exceptions mainly include the following types:
- SAXParseException: This is a basic XML parsing Exception, this exception is thrown when the parser finds an error while parsing the XML document.
- ParserConfigurationException: This exception is thrown when an illegal parser is created.
- IOException: This exception is thrown when an I/O error occurs while parsing the XML document.
- SAXException: This exception is thrown when the XML parser encounters other exceptions.
2. Methods to solve XML parsing exceptions
For the above common XML parsing exceptions, we can take the following methods to solve them:
- Use Try- Catch block handles exceptions
This is the most basic method of handling exceptions. Use the Try-Catch block to capture exceptions thrown during XML parsing and handle the exceptions accordingly. For example:
try { // 解析XML } catch (SAXParseException e) { // 处理解析异常 } catch (ParserConfigurationException e) { // 处理解析异常 } catch (IOException e) { // 处理解析异常 } catch (SAXException e) { // 处理解析异常 }
- Use a suitable parser
In Java development, there are many XML parsers to choose from, such as DOM, SAX, StAX, etc. Each parser has its advantages and applicable scenarios. Reasonable selection of the appropriate parser can better solve XML parsing exceptions. For example, if you need to operate on the entire XML document, it is recommended to use a DOM parser; if you need to keep the XML input stream in memory and process the XML elements one by one, you can use a SAX parser. - Verify the validity of the XML document
Before parsing the XML document, you can first verify the validity of the XML document. By verifying whether the XML document conforms to the corresponding DTD (Document Type Definition) or XSD (XML Schema Definition) specification, problems in the XML document can be discovered early and subsequent parsing exceptions can be avoided. For example, code example using DTD for verification:
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); factory.setValidating(true); DocumentBuilder builder = factory.newDocumentBuilder(); builder.setErrorHandler(new ErrorHandler() { public void warning(SAXParseException exception) throws SAXException { // 处理验证警告 } public void error(SAXParseException exception) throws SAXException { // 处理验证错误 } public void fatalError(SAXParseException exception) throws SAXException { // 处理致命验证错误 } }); Document document = builder.parse(new File("example.xml"));
- Error handling strategy
According to specific business requirements and system architecture, an error handling strategy can be defined to handle XML parsing exceptions. For example, you can choose to record error logs, re-parse XML documents, give user prompts, etc. Depending on the specific situation, choosing an appropriate processing strategy can make the system more robust and reliable.
Conclusion:
In Java development, parsing XML files is one of the common tasks. However, when parsing XML, you may encounter various exceptions. This article introduces how to solve common XML parsing exceptions in Java development, including using Try-Catch blocks to handle exceptions, selecting an appropriate parser, verifying the validity of XML documents, and defining error handling strategies. By rationally using these methods, XML parsing exceptions can be better solved and the robustness and reliability of the program can be improved.
References:
- Oracle official documentation - XML parsing exception handling
- JavaTechNotes - Common XML Parsing Errors and Solutions
Key Words: Java development, XML parsing, exception handling, DOM, SAX, StAX
The above is the detailed content of Java deals with XML parsing exceptions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
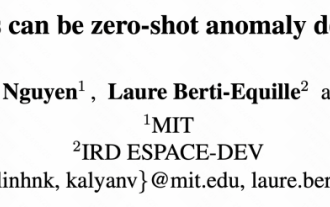
Today I would like to introduce to you an article published by MIT last week, using GPT-3.5-turbo to solve the problem of time series anomaly detection, and initially verifying the effectiveness of LLM in time series anomaly detection. There is no finetune in the whole process, and GPT-3.5-turbo is used directly for anomaly detection. The core of this article is how to convert time series into input that can be recognized by GPT-3.5-turbo, and how to design prompts or pipelines to let LLM solve the anomaly detection task. Let me introduce this work to you in detail. Image paper title: Largelanguagemodelscanbezero-shotanomalydete
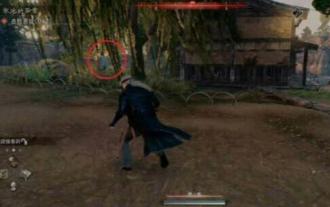
The abnormality in the pool is a side task in the game. Many players want to know how to complete the abnormality in the pool task. It is actually very simple. First, we must master the technique of shooting in the water before we can accept the task and investigate the source of the stench. Later, we discovered It turns out that there are a lot of corpses under the pool. Let’s take a look at this graphic guide for the unusual tasks in the pool in Rise of Ronin. Guide to unusual missions in the Ronin Rise Pool: 1. Talk to Iizuka and learn the technique of shooting in the water. 2. Go to the location in the picture below to receive the abnormal task in the pool. 3. Go to the mission location and talk to the NPC, and learn that there is a foul smell in the nearby pool. 4. Go to the pool to investigate. 5. Swim to the location in the picture below, dive underwater, and you will find a lot of corpses. 6. Use a camera to take pictures of the corpse. 7
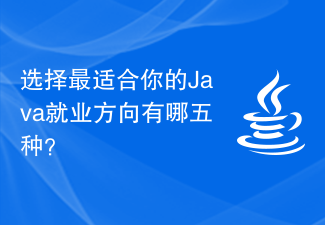
There are five employment directions in the Java industry, which one is suitable for you? Java, as a programming language widely used in the field of software development, has always been popular. Due to its strong cross-platform nature and rich development framework, Java developers have a wide range of employment opportunities in various industries. In the Java industry, there are five main employment directions, including JavaWeb development, mobile application development, big data development, embedded development and cloud computing development. Each direction has its characteristics and advantages. The five directions will be discussed below.
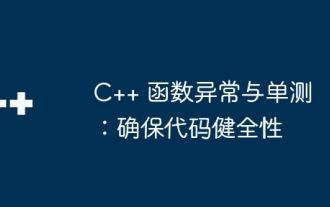
Exception handling and unit testing are important practices to ensure the soundness of C++ code. Exceptions are handled through try-catch blocks, and when the code throws an exception, it jumps to the catch block. Unit testing isolates code testing to verify that exception handling works as expected under different circumstances. Practical case: The sumArray function calculates the sum of array elements and throws an exception to handle an empty input array. Unit testing verifies the expected behavior of a function under abnormal circumstances, such as throwing an std::invalid_argument exception when an array is empty. Conclusion: By leveraging exception handling and unit testing, we can handle exceptions, prevent code from crashing, and ensure that the code behaves as expected under abnormal conditions.
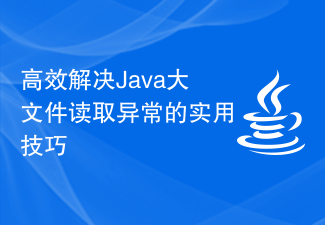
Practical tips for efficiently resolving large file read exceptions in Java require specific code examples. Overview: When processing large files, Java may face problems such as memory overflow and performance degradation. This article will introduce several practical techniques to effectively solve Java large file reading exceptions, and provide specific code examples. Background: When processing large files, we may need to read the file contents into memory for processing, such as searching, analyzing, extracting and other operations. However, when the file is large, the following problems are often encountered: Memory overflow: trying to copy the entire file at once
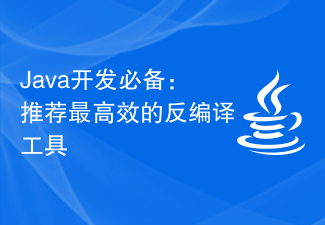
Essential for Java developers: Recommend the best decompilation tool, specific code examples are required Introduction: During the Java development process, we often encounter situations where we need to decompile existing Java classes. Decompilation can help us understand and learn other people's code, or make repairs and optimizations. This article will recommend several of the best Java decompilation tools and provide some specific code examples to help readers better learn and use these tools. 1. JD-GUIJD-GUI is a very popular open source
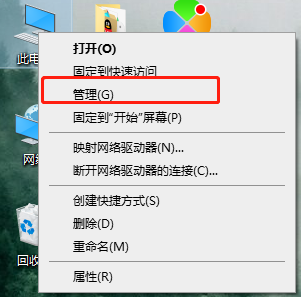
Some users suddenly find that their sound card driver is abnormal when using the computer. If this happens, you can update the driver from the device manager or roll back the driver to see if the problem is solved successfully. How to solve sound card driver abnormality 1. Right-click "This PC" and select "Manage" 2. Click "Device Manager", click "Sound" 3. Right-click the driver and select "Properties" 4. Click "Driver" at the top, and then click below You can choose "Update or rollback"
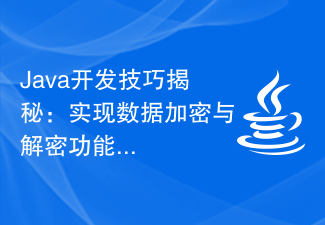
Java development skills revealed: Implementing data encryption and decryption functions In the current information age, data security has become a very important issue. In order to protect the security of sensitive data, many applications use encryption algorithms to encrypt the data. As a very popular programming language, Java also provides a rich library of encryption technologies and tools. This article will reveal some techniques for implementing data encryption and decryption functions in Java development to help developers better protect data security. 1. Selection of data encryption algorithm Java supports many
