Go language concurrent task scheduling solution
Methods to solve concurrent task scheduling problems in Go language development
With the continuous upgrading of computer hardware and the improvement of performance, the demand for concurrent processing in software development has also increased. As a modern concurrent programming language, Go language has certain advantages in solving concurrent task scheduling problems. This article will introduce some methods to solve concurrent task scheduling problems in Go language development.
1. Using goroutine and channel
In the Go language, goroutine is a lightweight thread that can execute multiple tasks in parallel during development. By using goroutines, tasks can be split into multiple subtasks and executed concurrently. At the same time, using channels for communication and data synchronization between tasks can effectively solve concurrent task scheduling problems.
For example, suppose we have a function that needs to execute multiple tasks at the same time. We can use goroutine to execute these tasks in parallel, and then use a channel to wait for the task to complete and collect the results of the task.
func parallelExecuteTasks(tasks []func() int) []int { results := make([]int, len(tasks)) done := make(chan bool) for i, task := range tasks { go func(i int, task func() int) { results[i] = task() done <- true }(i, task) } for range tasks { <-done } return results }
In the above example, we used a done channel to wait for the completion of the task. Each goroutine will store the results of the task in the results slice and notify the main thread that the task has been completed through the done channel. The main thread waits for the completion of all tasks by receiving messages from the done channel.
2. Use the sync package
The standard library of the Go language provides sync and atomic packages to solve concurrent task scheduling problems. The WaitGroup type in the sync package makes it convenient to wait for the completion of a group of concurrent tasks.
func parallelExecuteTasks(tasks []func() int) []int { var wg sync.WaitGroup results := make([]int, len(tasks)) for i, task := range tasks { wg.Add(1) go func(i int, task func() int) { results[i] = task() wg.Done() }(i, task) } wg.Wait() return results }
In the above example, we used a WaitGroup to wait for all tasks to be completed. Each goroutine notifies WaitGroup through the Done method when executing a task, and the main thread waits for the completion of all tasks by calling the Wait method.
3. Using the context package
The context package of the Go language is a package used to pass request range data, control the life cycle of goroutine, and handle cancellation operations. By using the context package, you can easily control and cancel tasks during the concurrent task scheduling process.
func parallelExecuteTasks(ctx context.Context, tasks []func() int) []int { results := make([]int, len(tasks)) wg := sync.WaitGroup{} for i, task := range tasks { wg.Add(1) go func(i int, task func() int) { defer wg.Done() select { case <-ctx.Done(): return default: results[i] = task() } }(i, task) } wg.Wait() return results }
In the above example, we pass context as a parameter to the parallelExecuteTasks function to control the life cycle of the task. In each goroutine, we use the select statement to monitor whether the context is canceled. If it is canceled, it will return directly, otherwise the task will be executed.
Summary:
By using goroutine and channel, sync package and context package, we can effectively solve the concurrent task scheduling problem in Go language development. These methods can help us make better use of the concurrency features of the Go language and improve program efficiency and performance. When encountering concurrent task scheduling problems during the development process, you can choose an appropriate method to solve it according to the specific situation.
The above is the detailed content of Go language concurrent task scheduling solution. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
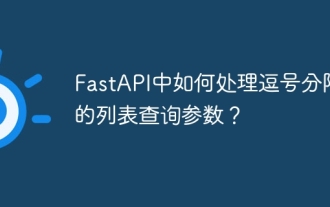
Fastapi ...
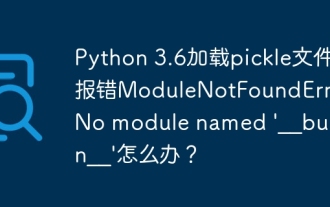
Loading pickle file in Python 3.6 environment error: ModuleNotFoundError:Nomodulenamed...
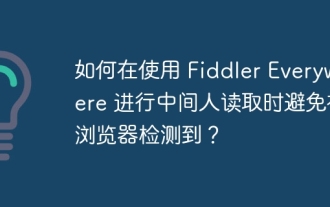
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
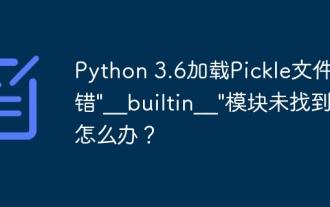
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...
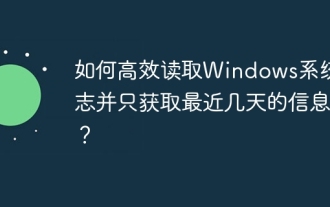
Efficient reading of Windows system logs: Reversely traverse Evtx files When using Python to process Windows system log files (.evtx), direct reading will be from the earliest...
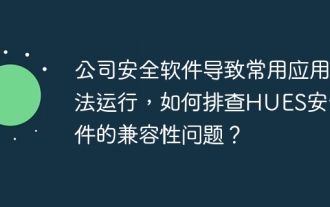
Compatibility issues with company security software and application and troubleshooting. Many companies will install security software in order to ensure internal network security. However, sometimes security software...
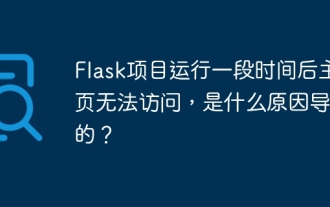
After running for a period of time, the Flask project cannot access the homepage. Troubleshooting recently encountered a difficult problem: in CentOS...
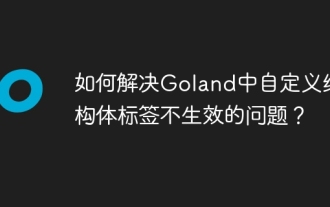
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
