


PHP implements the editing and deletion functions of question answers in the knowledge question and answer website.
PHP implements the editing and deletion functions of question answers in the knowledge question and answer website
In the knowledge question and answer website, in order to ensure the quality of the questions and answers, users often need to edit and delete the published questions and answers operate. PHP is a commonly used back-end programming language with powerful capabilities for processing data and database operations, making it very suitable for implementing these functions. This article will introduce how to use PHP to implement the question answer editing and deletion functions in the knowledge Q&A website.
1. Database design
First, we need to design a database to store question and answer information. Suppose we need to store the title, content, and publication time of the question, and the content and publication time of the answer. We can create a table called "questions" to store the questions' information, and a table called "answers" to store the answers' information. The design of the
questions table is as follows:
CREATE TABLE questions (
id INT AUTO_INCREMENT PRIMARY KEY, title VARCHAR(255) NOT NULL, content TEXT NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
answers The design of the table is as follows:
CREATE TABLE answers (
id INT AUTO_INCREMENT PRIMARY KEY, question_id INT NOT NULL, content TEXT NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP, FOREIGN KEY (question_id) REFERENCES questions(id) ON DELETE CASCADE
);
In the answers table, we use the question_id foreign key to associate answers and questions. And when the question is deleted through the ON DELETE CASCADE setting, the answers associated with it will also be automatically deleted.
2. Implement the question and answer editing function
In PHP, we can obtain the questions and answer content submitted by the user through the data of the HTTP request. Then, edit the questions and answers by connecting to the database and executing SQL statements.
<?php // 获取用户提交的问题内容 $title = $_POST['title']; $content = $_POST['content']; // 连接数据库 $pdo = new PDO('mysql:host=localhost;dbname=your_database_name', 'your_username', 'your_password'); // 编辑问题 $id = $_POST['question_id']; $stmt = $pdo->prepare("UPDATE questions SET title=?, content=? WHERE id=?"); $stmt->execute([$title, $content, $id]); // 跳转回问题详情页 header("Location: question.php?id=$id"); exit; ?>
In the above code, we first obtain the title and content of the question submitted by the user, then use the prepare method to prepare the SQL statement, and use the execute method to execute the SQL statement and update the question content submitted by the user to the database. . Finally, use the header function to redirect the user to the issue details page.
3. Implement the question and answer deletion function
Similar to question editing, we can also obtain the ID of the question or answer that the user wants to delete through the HTTP request data. Then, delete operations are performed by connecting to the database and executing SQL statements.
<?php // 获取用户提交的问题或答案 ID $id = $_GET['id']; // 连接数据库 $pdo = new PDO('mysql:host=localhost;dbname=your_database_name', 'your_username', 'your_password'); if (isset($_GET['question'])) { // 删除问题及其关联的答案 $stmt = $pdo->prepare("DELETE FROM questions WHERE id=?"); $stmt->execute([$id]); } else { // 删除答案 $stmt = $pdo->prepare("DELETE FROM answers WHERE id=?"); $stmt->execute([$id]); } // 跳转回问题详情页 header("Location: question.php"); exit; ?>
In the above code, we first determine whether the user wants to delete a question or an answer, and then prepare and execute the corresponding SQL statement according to different situations. Finally, the header function is also used to redirect the user back to the question details page.
Conclusion
It is very simple to implement the question answer editing and deletion functions in the knowledge Q&A website through PHP. You only need to connect to the database and execute the corresponding SQL statement. I hope this article can help you understand how to use PHP to achieve these functions. If you still have any questions or doubts, you can check the official PHP documentation or refer to other related tutorials. I wish you good results in your development!
The above is the detailed content of PHP implements the editing and deletion functions of question answers in the knowledge question and answer website.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


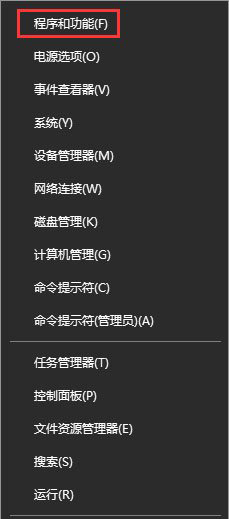
It was found that there is an inetpub folder on the C drive of the computer that takes up a lot of memory. What is this inetpub folder? Can it be deleted directly? In fact, inetpub is a folder on the IIS server. The full name of IIS is Internet Information Services, which is Internet Information Services. It can be used to build and debug websites. If it is not needed, it can be uninstalled. The specific method is as follows: 1. Right-click the Start menu and select "Programs and Features". 2. After opening, click "Turn Windows features on or off". 3. In the Windows feature list, uncheck II
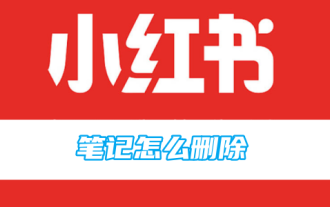
How to delete Xiaohongshu notes? Notes can be edited in the Xiaohongshu APP. Most users don’t know how to delete Xiaohongshu notes. Next, the editor brings users pictures and texts on how to delete Xiaohongshu notes. Tutorial, interested users come and take a look! Xiaohongshu usage tutorial How to delete Xiaohongshu notes 1. First open the Xiaohongshu APP and enter the main page, select [Me] in the lower right corner to enter the special area; 2. Then in the My area, click on the note page shown in the picture below , select the note you want to delete; 3. Enter the note page, click [three dots] in the upper right corner; 4. Finally, the function bar will expand at the bottom, click [Delete] to complete.
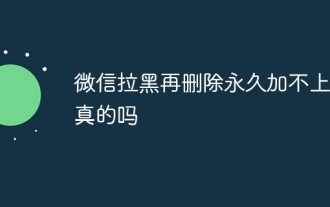
1. First of all, it is false to block and delete someone permanently and not add them permanently. If you want to add the other party after you have blocked them and deleted them, you only need the other party's consent. 2. If a user blocks someone, the other party will not be able to send messages to the user, view the user's circle of friends, or make calls with the user. 3. Blocking does not mean deleting the other party from the user's WeChat contact list. 4. If the user deletes the other party from the user's WeChat contact list after blocking them, there is no way to recover after deletion. 5. If the user wants to add the other party as a friend again, the other party needs to agree and add the user again.
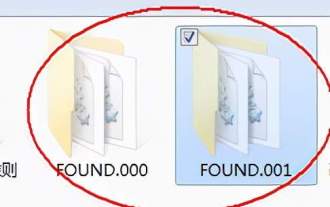
In the process of daily use of the computer, you may receive an error message that the found.000 file is lost and damaged. What folder is this found.000? Can it be deleted if it is no longer useful? Since so many people do not know this file, let me tell you about the found.000 folder in detail~ 1. What is the found.000 folder? When the computer is partially or completely lost due to illegal shutdown, , you can find the special folder named "found.000" and the files with the ".chk" extension contained inside it in the specified directory located in the system partition. This "fo
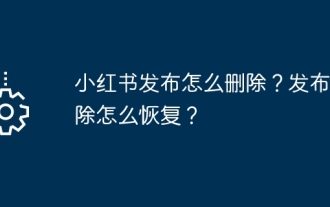
As a popular social e-commerce platform, Xiaohongshu has attracted a large number of users to share their daily life and shopping experiences. Sometimes we may inadvertently publish some inappropriate content, which needs to be deleted in time to better maintain our personal image or comply with platform regulations. 1. How to delete Xiaohongshu releases? 1. Log in to your Xiaohongshu account and enter your personal homepage. 2. At the bottom of the personal homepage, find the "My Creations" option and click to enter. 3. On the "My Creations" page, you can see all published content, including notes, videos, etc. 4. Find the content that needs to be deleted and click the "..." button on the right. 5. In the pop-up menu, select the "Delete" option. 6. After confirming the deletion, the content will disappear from your personal homepage and public page.
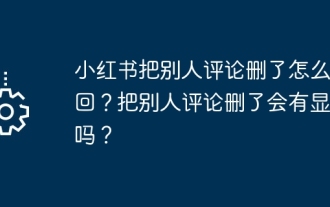
Xiaohongshu is a popular social e-commerce platform, and interactive comments between users are an indispensable method of communication on the platform. Occasionally, we may find that our comments have been deleted by others, which can be confusing. 1. How can I retrieve someone else’s deleted comments on Xiaohongshu? When you find that your comments have been deleted, you can first try to directly search for relevant posts or products on the platform to see if you can still find the comment. If the comment is still displayed after being deleted, it may have been deleted by the original post owner. At this time, you can try to contact the original post owner to ask the reason for deleting the comment and request to restore the comment. If a comment has been completely deleted and cannot be found on the original post, the chances of it being reinstated on the platform are relatively slim. You can try other ways
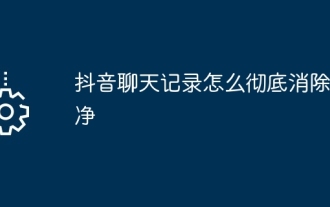
1. Open the Douyin app, click [Message] at the bottom of the interface, and click the chat conversation entry that needs to be deleted. 2. Long press any chat record, click [Multiple Select], and check the chat records you want to delete. 3. Click the [Delete] button in the lower right corner and select [Confirm deletion] in the pop-up window to permanently delete these records.
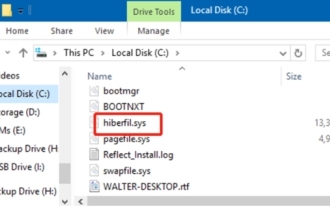
Recently, many netizens have asked the editor, what is the file hiberfil.sys? Can hiberfil.sys take up a lot of C drive space and be deleted? The editor can tell you that the hiberfil.sys file can be deleted. Let’s take a look at the details below. hiberfil.sys is a hidden file in the Windows system and also a system hibernation file. It is usually stored in the root directory of the C drive, and its size is equivalent to the size of the system's installed memory. This file is used when the computer is hibernated and contains the memory data of the current system so that it can be quickly restored to the previous state during recovery. Since its size is equal to the memory capacity, it may take up a larger amount of hard drive space. hiber
