


The second-hand recycling website uses the shopping cart real-time update function developed in PHP
The second-hand recycling website uses the real-time update function of the shopping cart developed by PHP
With the improvement of environmental protection awareness in modern society, more and more people have begun to pay attention to the recycling of second-hand items. In order to facilitate people to trade second-hand recycled items, many second-hand recycling websites have emerged.
On second-hand recycling websites, the shopping cart function is an indispensable and important component. It helps users conveniently manage and track their selected second-hand items, and updates the number of items in the shopping cart in real time. This article will introduce how to use PHP to develop a shopping cart with real-time update function.
First, we need to create a table in the database to store shopping cart information. In this table, we can store fields such as user ID, product ID, product name, product quantity, product unit price, etc. Here is an example of how the table might be structured:
CREATE TABLE shopping_cart ( id INT PRIMARY KEY AUTO_INCREMENT, user_id INT, product_id INT, product_name VARCHAR(255), quantity INT, price DECIMAL(10, 2) );
Next, we need to create a shopping cart page for users to select second-hand items. On this page we can list all recyclable items and provide an "Add to Cart" button for each item. When the user clicks the button, we can call a PHP script to add the selected item to the shopping cart.
Sample code:
<?php // 获取商品信息 $product_id = $_POST['product_id']; $product_name = $_POST['product_name']; $price = $_POST['price']; // 获取用户信息(这里假设用户已登录) $user_id = $_SESSION['user_id']; // 检查购物车中是否已经存在相同的商品 $query = "SELECT * FROM shopping_cart WHERE user_id = $user_id AND product_id = $product_id"; $result = mysqli_query($connection, $query); if (mysqli_num_rows($result) > 0) { // 如果已存在,则更新商品数量 $row = mysqli_fetch_assoc($result); $quantity = $row['quantity'] + 1; $update_query = "UPDATE shopping_cart SET quantity = $quantity WHERE user_id = $user_id AND product_id = $product_id"; mysqli_query($connection, $update_query); } else { // 如果不存在,则添加新的商品到购物车 $insert_query = "INSERT INTO shopping_cart (user_id, product_id, product_name, quantity, price) VALUES ($user_id, $product_id, '$product_name', 1, $price)"; mysqli_query($connection, $insert_query); } // 返回购物车页面 header('Location: shopping_cart.php'); ?>
In the shopping cart page, we can display the items in the shopping cart and provide the function of modifying the quantity. In order to achieve real-time update effect, we can use Ajax technology to achieve it.
Sample code:
// 监听数量输入框的变化 $('.quantity-input').change(function() { var product_id = $(this).data('product-id'); var new_quantity = $(this).val(); // 向服务器发送Ajax请求,更新购物车中商品的数量 $.ajax({ url: 'update_quantity.php', type: 'POST', data: { product_id: product_id, new_quantity: new_quantity }, success: function(response) { // 更新购物车显示 $('.product-quantity[data-product-id="' + product_id + '"]').text(new_quantity); calculateTotalPrice(); } }); }); // 计算总价 function calculateTotalPrice() { var total_price = 0; $('.product-row').each(function() { var price = $(this).find('.product-price').text(); var quantity = $(this).find('.product-quantity').text(); total_price += parseFloat(price) * parseInt(quantity); }); $('.total-price').text(total_price); }
On the server side, we need to write a PHP script that updates the number of items in the shopping cart.
Sample code:
<?php // 获取商品和新数量 $product_id = $_POST['product_id']; $new_quantity = $_POST['new_quantity']; // 获取用户ID(这里假设用户已登录) $user_id = $_SESSION['user_id']; // 更新购物车中商品的数量 $update_query = "UPDATE shopping_cart SET quantity = $new_quantity WHERE user_id = $user_id AND product_id = $product_id"; mysqli_query($connection, $update_query); ?>
Through the above sample code, we can implement a shopping cart with real-time update function. Users can easily add, delete and update items in the shopping cart, making it easier to trade second-hand items.
To sum up, the second-hand recycling website uses the shopping cart real-time update function developed in PHP to provide users with an efficient and convenient experience. The shopping cart function allows users to easily manage and track selected second-hand items, and update the item quantity in real time. Through reasonable use of PHP and Ajax technology, we can easily achieve this function.
The above is the detailed content of The second-hand recycling website uses the shopping cart real-time update function developed in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
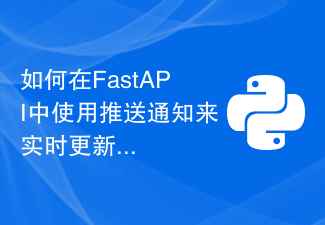
How to use push notifications in FastAPI to update data in real time Introduction: With the continuous development of the Internet, real-time data updates are becoming more and more important. For example, in application scenarios such as real-time trading, real-time monitoring, and real-time gaming, we need to update data in a timely manner to provide the most accurate information and the best user experience. FastAPI is a modern Python-based web framework that provides a simple and efficient way to build high-performance web applications. This article will introduce how to use FastAPI to implement
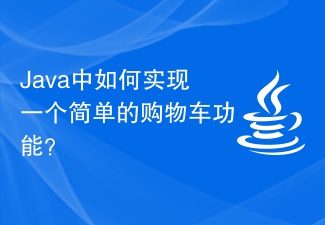
How to implement a simple shopping cart function in Java? The shopping cart is an important feature of an online store, which allows users to add items they want to purchase to the shopping cart and manage the items. In Java, we can implement a simple shopping cart function by using object-oriented approach. First, we need to define a product category. This class contains attributes such as product name, price, and quantity, as well as corresponding Getter and Setter methods. For example: publicclassProduct
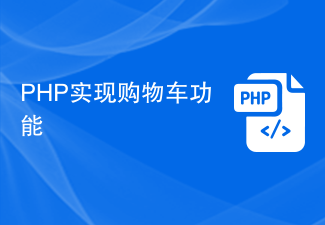
In our daily lives, online shopping has become a very common way of consumption, and the shopping cart function is also one of the important components of online shopping. So, this article will introduce how to use PHP language to implement shopping cart related functions. 1. Technical background The shopping cart is a common function on online shopping websites. When users browse some products on a website, they can add those items to a virtual shopping cart for easy selection and management during the subsequent checkout process. A shopping cart usually includes the following basic functions: Add items:

PHP mall development skills: Design shopping cart and order synchronization functions In a mall website, shopping cart and orders are indispensable functions. The shopping cart is used for users to purchase products and save them to a temporary shopping cart, while the order is a record generated after the user confirms the purchase of the product. In order to improve user experience and reduce errors, it is very important to design a shopping cart and order synchronization function. 1. The Concept of Shopping Cart and Order A shopping cart is usually a temporary container used to store items purchased by users. Users can add products to the shopping cart for easy browsing and management.
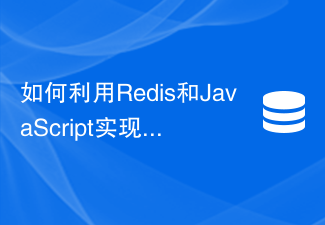
How to use Redis and JavaScript to implement the shopping cart function. The shopping cart is one of the very common functions in e-commerce websites. It allows users to add items of interest to the shopping cart, making it convenient for users to view and manage purchased items at any time. In this article, we will introduce how to implement the shopping cart function using Redis and JavaScript, and provide specific code examples. 1. Preparation Before starting, we need to ensure that Redis has been installed and configured, which can be done through the official website [https:/
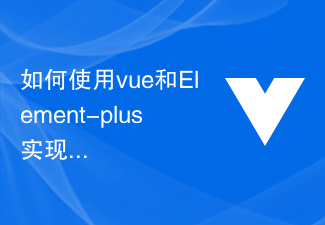
How to use Vue and ElementPlus to achieve real-time updates and real-time display Introduction: Vue is a front-end framework that provides real-time response and data binding features, allowing us to quickly build interactive user interfaces. ElementPlus is a component library based on Vue3, which provides a rich set of UI components to allow developers to build applications more conveniently. In many scenarios, we need to implement real-time update and real-time display functions, such as chat applications, real-time data
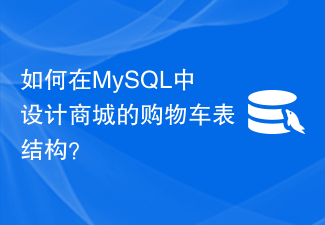
How to design the shopping cart table structure of the mall in MySQL? With the rapid development of e-commerce, shopping carts have become an important part of online malls. The shopping cart is used to save the products purchased by users and related information, providing users with a convenient and fast shopping experience. Designing a reasonable shopping cart table structure in MySQL can help developers store and manage shopping cart data effectively. This article will introduce how to design the shopping cart table structure of the mall in MySQL and provide some specific code examples. First, the shopping cart table should contain
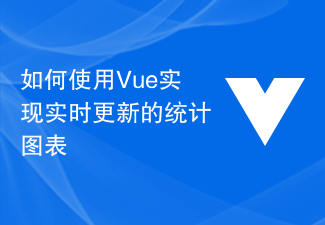
How to use Vue to implement real-time updated statistical charts Introduction: With the rapid development of the Internet and the explosive growth of data, data visualization has become an increasingly important way to convey information and analyze data. In front-end development, the Vue framework, as a popular JavaScript framework, can help us build interactive data visualization charts more efficiently. This article will introduce how to use Vue to implement a real-time updated statistical chart, obtain data in real time and update the chart through WebSocket, and provide relevant information at the same time.
