


Implement highly maintainable cross-platform applications using Go language
Use Go language to implement highly maintainable cross-platform applications
Overview:
In the field of software development, achieving highly maintainable cross-platform applications is an important goal. Go language has become the first choice of many developers due to its simplicity, high performance and rich standard library. This article will introduce how to use Go language to implement a highly maintainable cross-platform application and provide relevant code examples.
1. Use the features of Go language
- Static type system: The static type system of Go language can catch some errors during compilation to ensure the robustness of the code.
- Garbage collection: The Go language's own garbage collection mechanism can reduce developers' burden on memory management and improve the maintainability of the code.
- Concurrency support: Go language natively supports concurrent programming. Through the combination of goroutine and channel, concurrent operations can be easily achieved and the performance and maintainability of the code can be improved.
- Richness of the standard library: The standard library of the Go language provides a wealth of functions, such as file system operations, network programming, string processing, etc., which can help developers quickly implement various functions.
2. Design considerations for cross-platform applications
When implementing cross-platform applications, the following aspects of design and implementation need to be considered:
- Operation System differences: There are differences in system calls, path separators, etc. of different operating systems, and adaptations need to be made for different operating systems.
- GUI interface: Different operating systems have different GUI frameworks, which require the use of cross-platform GUI libraries for development, such as fyne, qt, etc.
- Data storage: Cross-platform applications need to consider persistent storage of data. You can use the database driver provided by the Go language, such as gorm, sqlx, etc.
3. Sample code
The following is a sample code for a simple cross-platform calculator application implemented in Go language:
package main import ( "fmt" "os" "runtime" ) func main() { if runtime.GOOS == "windows" { fmt.Println("This is a calculator application for Windows.") } else if runtime.GOOS == "darwin" { fmt.Println("This is a calculator application for macOS.") } else if runtime.GOOS == "linux" { fmt.Println("This is a calculator application for Linux.") } else { fmt.Println("This is a calculator application for an unknown operating system.") } fmt.Println("Please enter two numbers:") var num1, num2 float64 fmt.Scanln(&num1, &num2) fmt.Println("Operation options:") fmt.Println("1. Add") fmt.Println("2. Subtract") fmt.Println("3. Multiply") fmt.Println("4. Divide") var option int fmt.Scanln(&option) switch option { case 1: add(num1, num2) case 2: subtract(num1, num2) case 3: multiply(num1, num2) case 4: divide(num1, num2) default: fmt.Println("Invalid option.") } } func add(num1, num2 float64) { fmt.Printf("Result: %.2f ", num1+num2) } func subtract(num1, num2 float64) { fmt.Printf("Result: %.2f ", num1-num2) } func multiply(num1, num2 float64) { fmt.Printf("Result: %.2f ", num1*num2) } func divide(num1, num2 float64) { if num2 == 0 { fmt.Println("Cannot divide by zero.") } else { fmt.Printf("Result: %.2f ", num1/num2) } }
4. Summary
Passed Using the features of the Go language and following the design considerations of cross-platform applications, we can implement a highly maintainable cross-platform application. This article provides a simple sample code that developers can extend and optimize according to their own needs. I hope this article can be helpful to implement cross-platform applications in Go language.
Reference materials:
- Go language official website: https://golang.org/
- fyne official website: https://fyne.io/
- Qt official website: https://www.qt.io/
- gorm official website: https://gorm.io/
- sqlx official website: https:/ /github.com/jmoiron/sqlx
The above is the detailed content of Implement highly maintainable cross-platform applications using Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


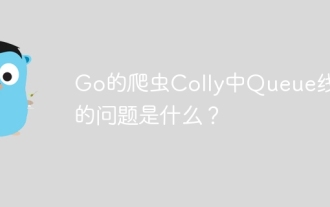
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
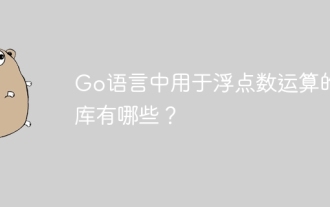
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
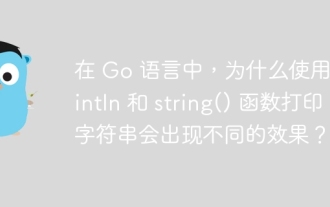
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
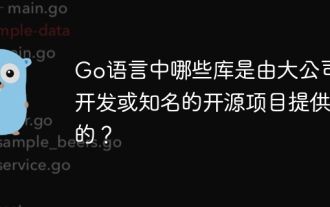
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
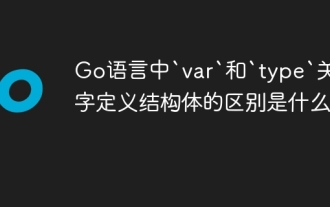
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
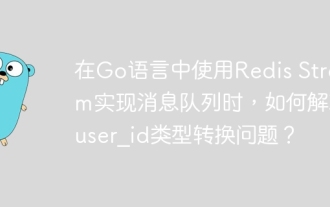
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
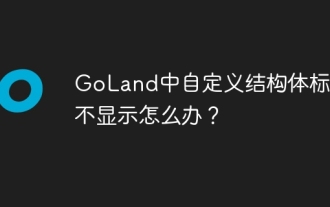
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
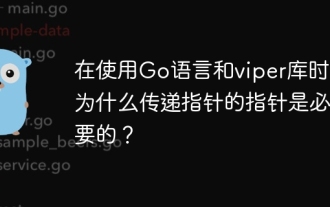
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
