How to implement file upload function in uniapp
How to implement the file upload function in uniapp
With the development of mobile applications, the file upload function is becoming more and more common in many applications. uniapp is a cross-platform development framework based on Vue.js that can easily develop mobile applications. In uniapp, it is also very simple to implement the file upload function. This article will show you how to implement file upload functionality in uniapp.
- Create an upload component
First, we need to create a component for uploading files. Create a folder named Upload under the components folder and create an Upload.vue file in it. The code is as follows:
<template> <div> <input type="file" @change="handleFileChange" accept="image/*" /> <button @click="uploadFile">上传</button> </div> </template> <script> export default { data() { return { filePath: '' // 保存文件路径 } }, methods: { handleFileChange(e) { const file = e.target.files[0] // 获取文件路径 this.filePath = URL.createObjectURL(file) }, uploadFile() { // 在此处编写上传文件的代码 } } } </script> <style> // 样式可根据需求自行修改 </style>
- Implementing file upload
Next, we need to write the logic for file upload. In the uploadFile method, we can use the uni.request method to send file data to the server. The code is as follows:
<script> export default { data() { return { filePath: '' // 保存文件路径 } }, methods: { handleFileChange(e) { const file = e.target.files[0] // 获取文件路径 this.filePath = URL.createObjectURL(file) }, uploadFile() { const self = this uni.chooseImage({ count: 1, success: function(res) { const tempFilePaths = res.tempFilePaths uni.uploadFile({ url: 'http://your-upload-url', filePath: tempFilePaths[0], name: 'file', success: function(res) { const data = res.data // 处理上传成功后的逻辑 }, fail: function(res) { // 处理上传失败后的逻辑 } }) } }) } } } </script>
In the above example, we used the uni.chooseImage method to let the user select the file to be uploaded, and then used the uni.uploadFile method to upload the file to the server. After the upload is successful or failed, we can perform corresponding processing based on the returned results.
- Using the upload component in the page
Finally, we need to use the upload component in the page. In a page under the pages folder, introduce and use the Upload component. For example, in the index.vue file in the index folder under the pages folder, the code is as follows:
<template> <div> <upload></upload> </div> </template> <script> import Upload from '@/components/Upload/Upload' export default { components: { Upload } } </script>
In this way, we can see a file upload component on the page.
Summary
Through uniapp’s cross-platform development framework, we can easily implement the file upload function in mobile applications. This article shows you how to create a component for uploading files and write the logic for file uploads. I hope this article can help you quickly implement the file upload function.
The above is the detailed content of How to implement file upload function in uniapp. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


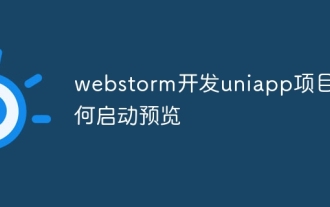
Steps to launch UniApp project preview in WebStorm: Install UniApp Development Tools plugin Connect to device settings WebSocket launch preview
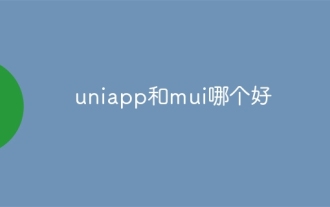
Generally speaking, uni-app is better when complex native functions are needed; MUI is better when simple or highly customized interfaces are needed. In addition, uni-app has: 1. Vue.js/JavaScript support; 2. Rich native components/API; 3. Good ecosystem. The disadvantages are: 1. Performance issues; 2. Difficulty in customizing the interface. MUI has: 1. Material Design support; 2. High flexibility; 3. Extensive component/theme library. The disadvantages are: 1. CSS dependency; 2. Does not provide native components; 3. Small ecosystem.
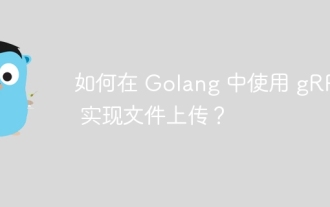
How to implement file upload using gRPC? Create supporting service definitions, including request and response messages. On the client, the file to be uploaded is opened and split into chunks, then streamed to the server via a gRPC stream. On the server side, file chunks are received and stored into a file. The server sends a response after the file upload is completed to indicate whether the upload was successful.
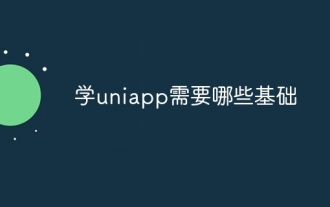
uniapp development requires the following foundations: front-end technology (HTML, CSS, JavaScript) mobile development knowledge (iOS and Android platforms) Node.js other foundations (version control tools, IDE, mobile development simulator or real machine debugging experience)
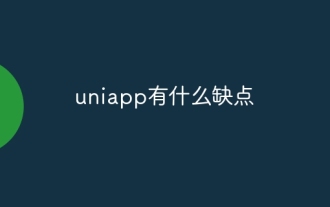
UniApp has many conveniences as a cross-platform development framework, but its shortcomings are also obvious: performance is limited by the hybrid development mode, resulting in poor opening speed, page rendering, and interactive response. The ecosystem is imperfect and there are few components and libraries in specific fields, which limits creativity and the realization of complex functions. Compatibility issues on different platforms are prone to style differences and inconsistent API support. The security mechanism of WebView is different from native applications, which may reduce application security. Application releases and updates that support multiple platforms at the same time require multiple compilations and packages, increasing development and maintenance costs.
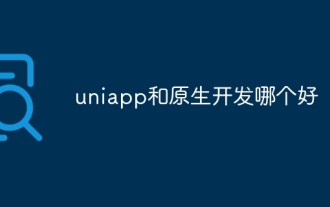
When choosing between UniApp and native development, you should consider development cost, performance, user experience, and flexibility. The advantages of UniApp are cross-platform development, rapid iteration, easy learning and built-in plug-ins, while native development is superior in performance, stability, native experience and scalability. Weigh the pros and cons based on specific project needs. UniApp is suitable for beginners, and native development is suitable for complex applications that pursue high performance and seamless experience.
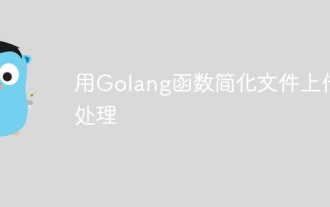
Answer: Yes, Golang provides functions that simplify file upload processing. Details: The MultipartFile type provides access to file metadata and content. The FormFile function gets a specific file from the form request. The ParseForm and ParseMultipartForm functions are used to parse form data and multipart form data. Using these functions simplifies the file processing process and allows developers to focus on business logic.
