


Method to realize real-time processing and quick preview of images using PHP and Qiniu cloud storage interface
Methods to use PHP and Qiniu Cloud Storage interface to achieve real-time processing and quick preview of images
Abstract: This article will introduce how to use PHP and Qiniu Cloud Storage interface to achieve real-time processing and quick preview of images. We explain specific steps through sample code to help readers understand how to process images, generate thumbnails, and provide quick preview functions.
Introduction
With the rapid development of the Internet, pictures, as one of the important elements in information transmission, are widely used in various fields. In order to improve the user experience, we usually need to process images, such as generating thumbnails, adding watermarks, etc. At the same time, in high-concurrency scenarios, in order to provide fast preview effects, we need to rely on cloud storage services to store and accelerate image access.
Qiniu Cloud Storage is one of the leading cloud storage platforms in China. It provides rich interfaces and powerful functions to facilitate developers to process and store images. Combined with PHP as a server-side language, we can easily achieve real-time processing and quick preview of images.
Environment preparation
Before starting the implementation, we need to complete the following preparations:
- Register a Qiniu cloud storage account and create a new storage space .
- Install PHP, it is recommended to use the latest version.
- Install composer to manage PHP dependency packages.
Install dependent libraries
Open a terminal in the project directory and execute the following command to install the required dependent libraries:
composer require qiniu/php-sdk composer require intervention/image
Sample code
The following sample code demonstrates how to use PHP and Qiniu cloud storage interface to achieve real-time processing and quick preview of images.
<?php require 'vendor/autoload.php'; use InterventionImageImageManagerStatic as Image; use QiniuAuth; use QiniuStorageUploadManager; // 七牛云账号信息 $accessKey = '<Your Access Key>'; $secretKey = '<Your Secret Key>'; $bucket = '<Your Bucket Name>'; // 构建Auth对象 $auth = new Auth($accessKey, $secretKey); // 在前端直传文件时,可以使用七牛云的回调机制获取到上传成功的文件信息 $callbackBody = '{"key": $(key), "hash": $(etag), "width": $(imageInfo.width), "height": $(imageInfo.height)}'; $callbackUrl = '<Your Callback URL>'; $callbackHost = '<Your Callback Host>'; $policy = array( 'callbackUrl' => $callbackUrl, 'callbackBody' => $callbackBody, 'callbackHost' => $callbackHost, 'mimeLimit' => 'image/*', // 限制文件类型为图片 ); // 生成上传Token $token = $auth->uploadToken($bucket, null, 3600, $policy); // 处理图片并上传到七牛云 if ($_SERVER['REQUEST_METHOD'] === 'POST' && isset($_FILES['image'])) { $file = $_FILES['image']; if ($file['error'] === 0) { // 使用Intervention/Image库处理图片 $image = Image::make($file['tmp_name']); // 生成缩略图(宽度为300px) $thumbnail = $image->resize(300, null, function ($constraint) { $constraint->aspectRatio(); $constraint->upsize(); }); // 保存缩略图 $thumbnailFile = tempnam(sys_get_temp_dir(), 'thumbnail_'); $thumbnail->save($thumbnailFile); // 上传图片到七牛云 $uploader = new UploadManager(); $uploadResult = $uploader->putFile($token, null, $thumbnailFile); // 输出结果(包含上传成功后的文件信息) echo json_encode($uploadResult, JSON_PRETTY_PRINT); exit; } } ?> <!DOCTYPE html> <html> <head> <title>图片上传示例</title> </head> <body> <form method="post" enctype="multipart/form-data"> <input type="file" name="image"> <button type="submit">上传</button> </form> </body> </html>
Summary
By using PHP and Qiniu cloud storage interface, we can easily achieve real-time processing and quick preview of images. This article introduces the implementation steps in detail through sample code, and provides an example of image uploading, which readers can extend and modify according to their own needs.
In practical applications, we can learn more about the use of advanced functions, such as adding watermarks, cropping pictures, etc. according to the interface document of Qiniu Cloud Storage. At the same time, we can also use the CDN acceleration service provided by Qiniu Cloud Storage to speed up image access and improve user experience. I hope this article will be helpful to developers in image processing and storage in actual projects.
The above is the detailed content of Method to realize real-time processing and quick preview of images using PHP and Qiniu cloud storage interface. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
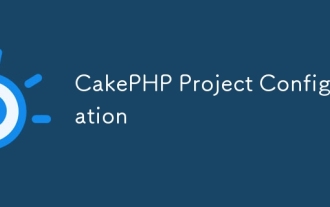
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
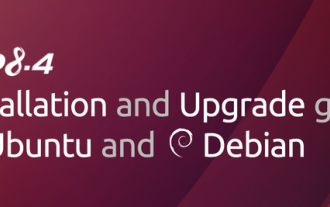
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
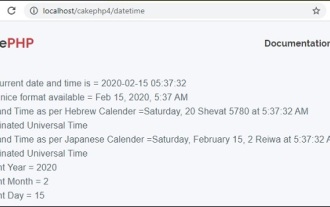
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
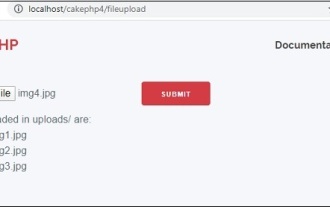
To work on file upload we are going to use the form helper. Here, is an example for file upload.
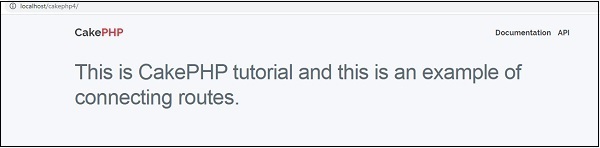
In this chapter, we are going to learn the following topics related to routing ?
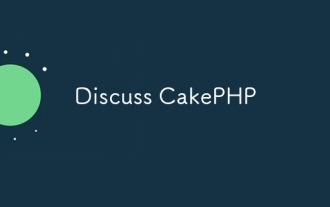
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
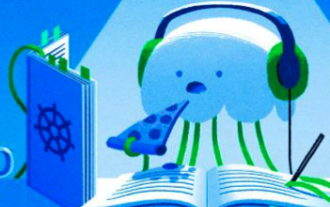
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
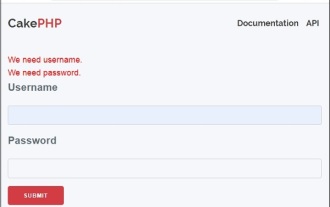
Validator can be created by adding the following two lines in the controller.
