Web Worker usage guide for HTML5_html5 tutorial tips
Web Workers is a javascript multi-threading solution provided by HTML5. We can hand over some computationally intensive code to web workers to run without freezing the user interface.
1: How to use Worker
The basic principle of Web Worker is to use the Worker class to load a javascript file in the current main thread of javascript to open a new thread, which has the effect of non-blocking execution and provides data between the main thread and the new thread. Exchanged interfaces: postMessage, onmessage.
So how to use it, let’s look at an example:
- //worker.js
- onmessage =function (evt){
- var d = evt.data;//Get the data sent through evt.data
- postMessage( d );//Send the obtained data to the main thread
- }
HTML page: test.html
- >
- <html>
- <<span style="width: auto; height: auto; float: none;" id="20_nwp"><a style="text-decoration: none;" mpid="20" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=head&k0=head&kdi0=0&luki=6&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="20_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">headspan>a>span>>
- <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
- <script type="text/<span style="width: auto; height: auto; float: none;" id="21_nwp"><a style="text-decoration: none;" mpid="21" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=javascript&k0=javascript&kdi0=0&luki=9&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="21_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">javascriptspan>a>span>">
- //WEB页主线程
- var worker =new Worker("worker.js"); //创建一个Worker对象并向它传递将在新线程中执行的脚本的URL
- worker.postMessage("hello world"); //向worker发送数据
- worker.onmessage =function(evt){ //接收worker传过来的数据<span style="width: auto; height: auto; float: none;" id="22_nwp"><a style="text-decoration: none;" mpid="22" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=����&k0=����&kdi0=0&luki=2&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="22_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">函数span>a>span>
- console.log(evt.<span style="width: auto; height: auto; float: none;" id="23_nwp"><a style="text-decoration: none;" mpid="23" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=data&k0=data&kdi0=0&luki=4&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="23_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">dataspan>a>span>); //输出worker发送来的数据
- }
- script>
- head>
- <body>body>
- html>
After opening test.html with Chrome browser, the console outputs "hello world", indicating that the program execution is successful.
Through this example we can see that using web workers is mainly divided into the following parts
WEB main thread:
1. Load a JS file through worker = new Worker( url ) to create a worker and return a worker instance.
2. Send data to the worker through the worker.postMessage(data) method.
3. Bind the worker.onmessage method to receive the data sent by the worker.
4. You can use worker.terminate() to terminate the execution of a worker.
Worker new thread:
1. Send data to the main thread through the postMessage(data) method.
2. Bind the onmessage method to receive the data sent by the main thread.
2: What can Worker do
Now that we know how to use web worker, what is its use and what problems can it help us solve. Let's look at an example of the fibonacci sequence.
Everyone knows that in mathematics, the fibonacci sequence is defined recursively: F0=0, F1=1, Fn=F(n-1) F(n-2) (n>=2, n∈N* ), and the common implementation of javascript is:
- var fibonacci =function(n) {
- return n <2? n : arguments.callee(n -1) arguments.callee(n -2);
- };
- //fibonacci(36)
Using this method in Chrome to execute the fibonacci sequence of 39 takes 19097 milliseconds, but when it comes to calculating 40, the browser directly prompts that the script is busy.
Since JavaScript is executed in a single thread, the browser cannot execute other JavaScript scripts during the process of calculating the sequence, and the UI rendering thread will also be suspended, causing the browser to enter a zombie state. Using a web worker to put the calculation process of the sequence into a new thread will avoid this situation. See the example specifically:
- //fibonacci.js
- varfibonacci =function(n) {
- return n <2? n : arguments.call(n -1) arguments.call(n -2);
- };
- onmessage =function(event) {
- var n = parseInt(event."width: auto; height: auto; float: none;" id="16_nwp">"text-decoration: none;" mpid="16" target="_blank" ref="http://cpro.baidu .com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=data&k0=data&kdi0=0&luki=4&n=10&p=baidu&q=0601 1078_cpr&rb=0&rs=1&seller_id=1&sid =45fdcf1cab219561&ssp2=1&time=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&url=0" id="16_nwl">"color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">data< /span, 10);
- postMessage(fibonacci(n));
- };
HTML Open:fibonacci.html
- >
- <html>
- <<span style="width: auto; height: auto; float: none;" id="11_nwp"><a style="text-decoration: none;" mpid="11" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=head&k0=head&kdi0=0&luki=6&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="11_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">headspan>a>span>>
- <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
- <title>web worker fibonaccititle>
- <script type="text/<span style="width: auto; height: auto; float: none;" id="12_nwp"><a style="text-decoration: none;" mpid="12" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=javascript&k0=javascript&kdi0=0&luki=9&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="12_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">javascriptspan>a>span>">
- onload =function(){
- var worker =new Worker('fibonacci.js');
- worker.addEventListener('message', function(event) {
- var timer2 = (new Date()).valueOf();
- console.log( '结果:' event.<span style="width: auto; height: auto; float: none;" id="13_nwp"><a style="text-decoration: none;" mpid="13" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=data&k0=data&kdi0=0&luki=4&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="13_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">dataspan>a>span>, '时间:' timer2, '用时:' ( timer2 - timer ) );
- }, false);
- var timer = (new Date()).valueOf();
- console.log('开始计算:40','时间:' timer );
- setTimeout(function(){
- console.log('定时器<span style="width: auto; height: auto; float: none;" id="14_nwp"><a style="text-decoration: none;" mpid="14" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=����&k0=����&kdi0=0&luki=2&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="14_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">函数span>a>span>在计算数列时执行了', '时间:' (new Date()).valueOf() );
- },1000);
- worker.postMessage(40);
- console.log('我在计算数列的时候执行了', '时间:' (new Date()).valueOf() );
- }
- script>
- <span style="width: auto; height: auto; float: none;" id="15_nwp"><a style="text-decoration: none;" mpid="15" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=head&k0=head&kdi0=0&luki=6&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="15_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">headspan>a>span>>
- <body>
- body>
- html>
在Chrome中打开fibonacci.html,控制台得到如下输出:
开始计算:40 时间:1316508212705
我在计算数列的时候执行了 时间:1316508212734
定时器
- <span style="width: auto; height: auto; float: none;" id="9_nwp"><a style="text-decoration: none;" mpid="9" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=����&k0=����&kdi0=0&luki=2&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="9_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">函数span>a>span>
在计算数列时执行了 时间:1316508213735
结果:102334155 时间:1316508262820 用时:50115
这个例子说明在worker中执行的fibonacci数列的计算并不会影响到主线程的代码执行,完全在自己独立的线程中计算,只是在计算完成之后将结果发回主线程。
利用web worker我们可以在前端执行一些复杂的大量运算而不会影响页面的展示,并且不会弹出恶心的脚本正忙提示。
下面这个例子使用了web worker来计算场景中的像素,场景打开时是一片一片进行绘制的,一个worker只计算一块像素值。
http://nerget.com/rayjs-mt/rayjs.html
三:Worker的其他尝试
我们已经知道Worker通过接收一个URL来创建一个worker,那么我们是否可以利用web worker来做一些类似jsonp的请求呢,大家知道jsonp是通过插入script标签来加载json数据的,而script元素在加载和执行过程中都是阻塞式的,如果能利用web worker实现异步加载将会非常不错。
下面这个例子将通过 web worker、jsonp、ajax三种不同的方式来加载一个169.42KB大小的JSON数据
- // /aj/webWorker/core.js
- function $E(id) {
- return document.getElementById(id);
- }
- onload =function() {
- //通过web worker加载
- $E('workerLoad').onclick =function() {
- var url ='http://js.wcdn.cn/aj/mblog/face2';
- var d = (new Date()).valueOf();
- var worker =new Worker(url);
- worker.onmessage =function(obj) {
- console.log('web worker: ' ((new Date()).valueOf() - d));
- };
- };
- //通过jsonp加载
- $E('jsonpLoad').onclick =function() {
- var url ='http://js.wcdn.cn/aj/mblog/face1';
- var d = (new Date()).valueOf();
- STK.core.io.scriptLoader({
- method:'post',
- url : url,
- onComplete : function() {
- console.log('jsonp: ' ((new Date()).valueOf() - d));
- }
- });
- };
- //通过ajax加载
- $E('ajaxLoad').onclick =function() {
- var url ='http://js.wcdn.cn/aj/mblog/face';
- var d = (new Date()).valueOf();
- STK.core.io.ajax({
- url : url,
- onComplete : function(json) {
- console.log('ajax: ' ((new Date()).valueOf() - d));
- }
- });
- };
- };
HTML页面:/aj/webWorker/worker.html
- >
- <html>
- <<span style="width: auto; height: auto; float: none;" id="4_nwp"><a style="text-decoration: none;" mpid="4" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=head&k0=head&kdi0=0&luki=6&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="4_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">headspan>a>span>>
- <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
- <title>Worker example: load <span style="width: auto; height: auto; float: none;" id="5_nwp"><a style="text-decoration: none;" mpid="5" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=data&k0=data&kdi0=0&luki=4&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="5_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">dataspan>a>span>title>
- <script src="http://js.t.sinajs.cn/STK/js/gaea.1.14.js" type="text/<span style="width: auto; height: auto; float: none;" id="6_nwp"><a style="text-decoration: none;" mpid="6" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=javascript&k0=javascript&kdi0=0&luki=9&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="6_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">javascriptspan>a>span>">script>
- <script type="text/javascript" src="http://js.wcdn.cn/aj/webWorker/core.js">script>
- head>
- <body>
- <input type="button" id="workerLoad" value="web worker加载">input>
- <input type="button" id="jsonpLoad" value="jsonp加载">input>
- <input type="button" id="<span style="width: auto; height: auto; float: none;" id="7_nwp"><a style="text-decoration: none;" mpid="7" target="_blank" href="http://cpro.baidu.com/cpro/ui/uijs.php?adclass=0&app_id=0&c=news&cf=1001&ch=0&di=128&fv=0&is_app=0&jk=619521ab1ccffd45&k=ajax&k0=ajax&kdi0=0&luki=8&n=10&p=baidu&q=06011078_cpr&rb=0&rs=1&seller_id=1&sid=45fdcf1cab219561&ssp2=1&stid=0&t=tpclicked3_hc&tu=u1922429&u=http://www.admin10000.com/document/1183.html&urlid=0" id="7_nwl"><span style="color:#0000ff;font-size:14px;width:auto;height:auto;float:none;">ajaxspan>a>span>Load" value="ajax加载">input>
- body>
- html>
设置HOST
通过 http://js.wcdn.cn/aj/webWorker/worker.html 访问页面然后分别通过三种方式加载数据,得到控制台输出:
jsonp: 25
ajax: 38
After trying several times, I found that the time to load data through jsonp and ajax is not much different, and the loading time of web worker has always been at a high level, so using web worker to load data is still relatively slow, even with large amounts of data. There is no advantage. It may be that it takes time for Worker to initialize new threads. There is no advantage other than being non-blocking during loading.
So can web worker support cross-domain js loading? This time we access the page through http://127.0.0.1/aj/webWorker/worker.html. When clicking the "web worker loading" loading button, Chrome will download There is no response, and an error is prompted under FF6. From this we can know that web worker does not support cross-domain loading of JS, which is bad news for websites that deploy static files to a separate static server.
So web worker can only be used to load json data in the same domain, but ajax can already do this, and it is more efficient and versatile. Let the Worker do what it is good at.
Four: Summary
Web workers look nice, but they are full of devils.
What we can do:
1. You can load a JS to perform a large number of complex calculations without hanging the main process, and communicate through postMessage, onmessage
2. You can load additional script files in the worker through importScripts(url)
3. You can use setTimeout(), clearTimeout(), setInterval(), and clearInterval()
4. You can use XMLHttpRequest to send requests
5. Can access some properties of navigator
What are the limitations:
1. Cannot load JS across domains
2. The code within the worker cannot access the DOM
3. The implementation of Worker in various browsers is not consistent. For example, FF allows the creation of new workers in workers, but Chrome does not allow it.
4. Not every browser supports this new feature

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
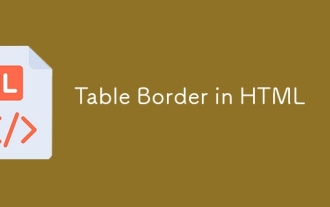
Guide to Table Border in HTML. Here we discuss multiple ways for defining table-border with examples of the Table Border in HTML.
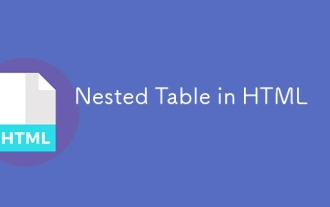
This is a guide to Nested Table in HTML. Here we discuss how to create a table within the table along with the respective examples.
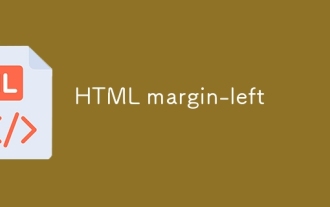
Guide to HTML margin-left. Here we discuss a brief overview on HTML margin-left and its Examples along with its Code Implementation.
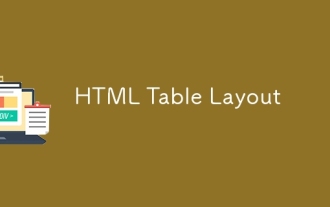
Guide to HTML Table Layout. Here we discuss the Values of HTML Table Layout along with the examples and outputs n detail.
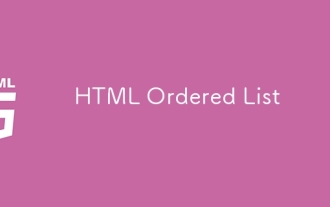
Guide to the HTML Ordered List. Here we also discuss introduction of HTML Ordered list and types along with their example respectively
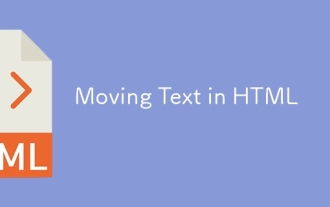
Guide to Moving Text in HTML. Here we discuss an introduction, how marquee tag work with syntax and examples to implement.
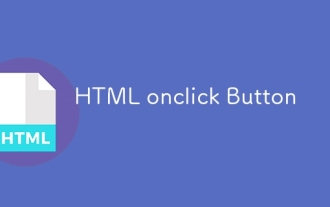
Guide to HTML onclick Button. Here we discuss their introduction, working, examples and onclick Event in various events respectively.
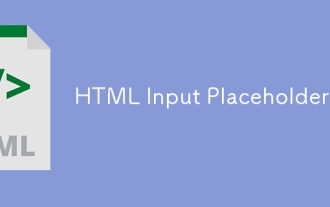
Guide to HTML Input Placeholder. Here we discuss the Examples of HTML Input Placeholder along with the codes and outputs.
