


Learn Python to implement Qiniu Cloud interface docking and realize image color adjustment function
Learn Python to implement Qiniu Cloud interface docking and realize image color adjustment function
Abstract:
With the rapid development of the Internet, the demand for image processing and storage is also increasing. The emergence of cloud storage services provides a convenient and efficient solution for image storage, and Qiniu Cloud is one of the most popular cloud storage services. This article will introduce how to use Python to implement Qiniu Cloud interface docking, and use Qiniu Cloud's image processing function to complete image color adjustment.
Article text:
1. Preparation:
First, we need to create a storage space (Bucket) on Qiniu Cloud and obtain the corresponding Access Key and Secret Key. These two keys will be used to connect to Qiniu Cloud interface. In addition, we also need to install the Python requests library.
2. Connect to Qiniu Cloud interface:
We can use Python's requests library to make HTTP requests, and send requests to Qiniu Cloud's API by constructing the corresponding request URL and request parameters.
import requests import hashlib import hmac import base64 access_key = "your_access_key" secret_key = "your_secret_key" bucket_name = "your_bucket_name" # 构建URL url = "http://rs.qiniu.com/stat/" + bucket_name # 查询存储空间信息的API接口 # 构建请求参数 params = {} params['bucket'] = bucket_name # 生成AccessToken sign = hmac.new(secret_key.encode('utf-8'), url.encode('utf-8'), hashlib.sha1).digest() token = access_key + ':' + base64.urlsafe_b64encode(sign).decode('utf-8') # 发送GET请求 response = requests.get(url, params=params, headers={'Authorization': 'Qiniu ' + token}) # 处理返回结果 if response.status_code == 200: result = response.json() # 将返回结果转为JSON格式 print(result) else: print("Request Failed:", response.status_code)
Through the above code, we can obtain the basic information of the storage space.
3. Image color adjustment:
Qiniu Cloud provides rich image processing functions. We can achieve color adjustment effects by adjusting the image processing parameters.
def image_color_adjust(image_url, bucket_name): access_key = "your_access_key" secret_key = "your_secret_key" # 构建URL url = "http://<domain>/style/<style>/<source>" # 构建请求参数 params = {} params['bucket'] = bucket_name params['source'] = base64.urlsafe_b64encode(image_url.encode('utf-8')).decode('utf-8') params['style'] = "your_style" # 调整图片色彩的样式 # 生成AccessToken sign = hmac.new(secret_key.encode('utf-8'), url.encode('utf-8'), hashlib.sha1).digest() token = access_key + ':' + base64.urlsafe_b64encode(sign).decode('utf-8') # 发送GET请求 response = requests.get(url, params=params, headers={'Authorization': 'Qiniu ' + token}) # 处理返回结果 if response.status_code == 200: result = response.json() # 将返回结果转为JSON格式 print(result) else: print("Request Failed:", response.status_code) # 调用函数 image_url = "http://example.com/path/to/image.jpg" # 替换为需要调整色彩的图片URL bucket_name = "your_bucket_name" image_color_adjust(image_url, bucket_name)
<domain>
, <style>
and <source>
in the above code need to be replaced with the real domain name, Style and image URL. Through the above code, we can achieve the effect of color adjustment on the specified picture.
Summary:
This article uses Python as a tool to teach you how to implement Qiniu Cloud interface docking and use Qiniu Cloud's image processing function to complete picture color adjustment. By studying this article, you can further understand and apply the functions of Qiniu Cloud to achieve richer image processing effects. Hope this article is helpful to you!
The above is the detailed content of Learn Python to implement Qiniu Cloud interface docking and realize image color adjustment function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
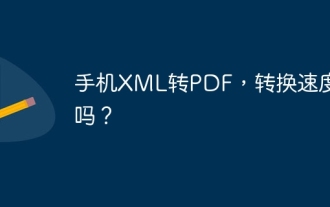
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
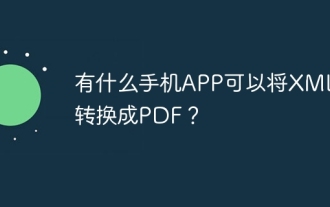
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
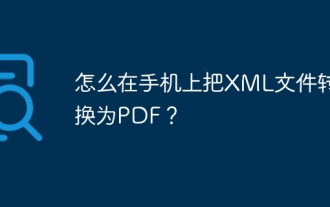
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
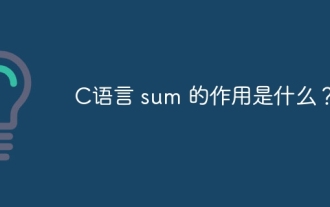
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
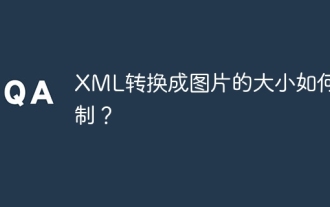
To generate images through XML, you need to use graph libraries (such as Pillow and JFreeChart) as bridges to generate images based on metadata (size, color) in XML. The key to controlling the size of the image is to adjust the values of the <width> and <height> tags in XML. However, in practical applications, the complexity of XML structure, the fineness of graph drawing, the speed of image generation and memory consumption, and the selection of image formats all have an impact on the generated image size. Therefore, it is necessary to have a deep understanding of XML structure, proficient in the graphics library, and consider factors such as optimization algorithms and image format selection.
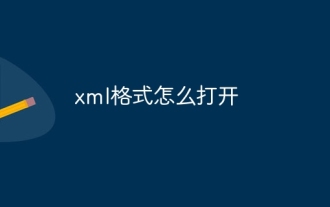
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
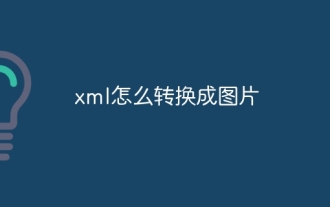
XML can be converted to images by using an XSLT converter or image library. XSLT Converter: Use an XSLT processor and stylesheet to convert XML to images. Image Library: Use libraries such as PIL or ImageMagick to create images from XML data, such as drawing shapes and text.
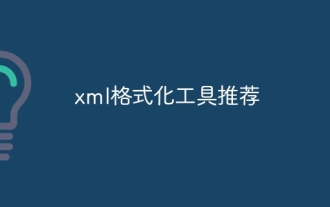
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
