How to implement data paging function through PHP and UniApp
How to implement data paging function through PHP and UniApp
Introduction:
When developing mobile applications based on UniApp, it is often necessary to obtain a large amount of data from the server and display it in the App. In order to improve user experience and application performance, we often need to display data in pages. This article will introduce how to use PHP and UniApp to implement data paging function, with code examples.
1. PHP part:
- Create database table and data
First we need to create a table in the database to store the data to be displayed. For example, we created a table named "user", which contains fields id, username, and age.
CREATE TABLE user
(
id
int(11) NOT NULL AUTO_INCREMENT,
username
varchar(255 ) NOT NULL,
age
int(11) NOT NULL,
PRIMARY KEY (id
)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
Then insert some test data:
INSERT INTO user
(id
, username
, age
) VALUES
(1, 'Zhang San', 18),
(2, 'Li Si', 20),
(3, 'Wang Wu', 25),
(4, 'Zhao Six', 30),
(5, 'Qianqi', 35),
(6, 'Sunba', 40),
(7, 'Zhoujiu', 45),
(8, 'Wu Shi', 50);
- Write PHP interface
Next we need to write a PHP interface to obtain data of a specific page number from the database.
header('Access-Control-Allow-Origin: *');
header('Content-Type: application/json');
// Connect to the database
$mysqli = new mysqli("localhost", "username", "password", "database");
// Check whether the connection is successful
if ( $mysqli->connect_errno) {
echo "Failed to connect to MySQL: " . $mysqli->connect_error; exit();
}
// Get the current page number
$page = isset($_GET['page']) ? $_GET['page' ] : 1;
//The number of data items displayed per page
$perPage = 3;
//Calculate the starting offset
$offset = ($page - 1) * $perPage;
//Query data
$result = $mysqli->query("SELECT * FROM user
LIMIT $offset, $perPage");
// Return data to the front end in JSON format
$data = array();
while ($row = $result->fetch_assoc()) {
$data[] = $row;
}
echo json_encode($data);
//Close the database connection
$mysqli->close();
?>
2. UniApp Part:
- Writing Vue components
In UniApp, we need to write a Vue component to initiate HTTP requests and display the obtained data.
<view v-for="user in userList" :key="user.id"> <text>{{ user.username }}</text> <text>{{ user.age }}</text> </view> <button @click="loadMore">加载更多</button>
<script> <br>export default {<br> data() {</p><div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>return { userList: [], // 存储用户列表数据 page: 1 // 当前页码 };</pre><div class="contentsignin">Copy after login</div></div><p>},<br> mounted() {</p><div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>this.loadData();</pre><div class="contentsignin">Copy after login</div></div><p>},<br> methods: {</p><div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>loadData() { uni.request({ url: 'http://your-php-server/api/getData.php', data: { page: this.page }, success: (res) => { if (res.statusCode === 200) { this.userList = this.userList.concat(res.data); } } }); }, loadMore() { this.page++; this.loadData(); }</pre><div class="contentsignin">Copy after login</div></div><p>}<br>};<br></script>
- Configure the request domain name
In the UniApp project, you need to configure a legal request domain name. Add the following code to the manifest.json file and replace it with your own PHP server address.
"networkTimeout": {
"request": 60000,
"downloadFile": 60000,
"uploadFile": 60000,
"websocket": 60000
},
"uni.request": {
"protocol": "https",
"domain": "your-php-server"
}
Above These are the steps and code examples for using PHP and UniApp to implement data paging function. Obtain the data of the specified page number from the database through the PHP interface and display the data in UniApp. When the user clicks the "Load More" button, the corresponding page number is sent and the data for the next page is loaded. In this way, the data paging function is realized, which improves user experience and application performance.
The above is the detailed content of How to implement data paging function through PHP and UniApp. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


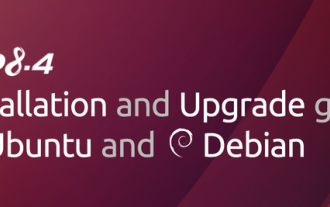
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
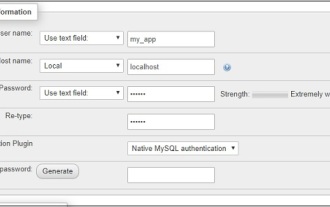
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
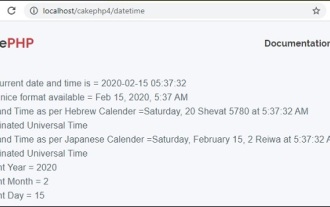
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
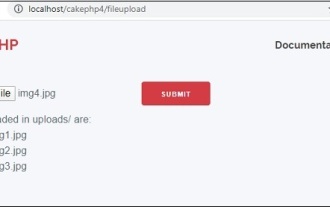
To work on file upload we are going to use the form helper. Here, is an example for file upload.
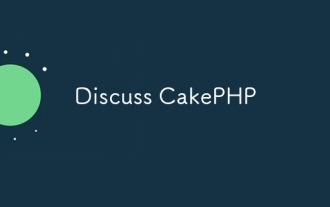
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
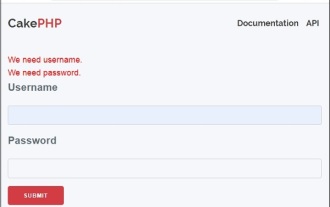
Validator can be created by adding the following two lines in the controller.
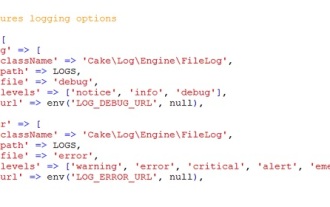
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
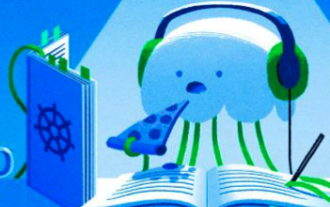
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
