


DingTalk Interface and PHP Customer Management Application Development Guide
Customer Management Application Development Guide for DingTalk Interface and PHP
In recent years, with the rapid development of the mobile Internet, enterprises’ demand for customer management has become more and more urgent. DingTalk is an enterprise-level communication and collaboration tool. Its rich API interface provides developers with the possibility of building enterprise-level applications. This article will introduce how to use the DingTalk interface and PHP to develop a simple customer management application, and provide code examples.
- Preparation
First, you need a DingTalk developer account. Register on the DingTalk developer platform and create an enterprise developer application, and obtain the App Key and App Secret of the application. This information will be used in subsequent code. - Create PHP project
Create a new PHP project locally, in which we will develop customer management applications. - Get DingTalk authorization code
In the customer management application, we need to obtain the DingTalk user's authorization code. After the user logs in on DingTalk and agrees to the authorization, we can obtain the authorization code to obtain the user information. The following is a sample code to obtain the DingTalk authorization code:
<?php $appkey = 'your_app_key'; $appsecret = 'your_app_secret'; $code = $_GET['code']; $requestData = [ 'method' => 'dingtalk.smartwork.bpms.processinstance.create', 'format' => 'json', 'access_token' => '', 'code' => $code ]; $authUrl = 'https://oapi.dingtalk.com/user/getuserinfo'; $authUrl .= '?corpid=' . $appkey; $authUrl .= '&corpsecret=' . $appsecret; $authUrl .= '&code=' . $code; $response = file_get_contents($authUrl); $userInfo = json_decode($response, true); if ($userInfo && $userInfo['errcode'] == 0) { $authCode = $userInfo['user_info']['auth_code']; // 将授权码存入数据库或其他合适的地方 } else { // 处理错误情况 }
- Get user information
Through the authorization code, we can obtain the user's detailed information through the DingTalk interface, including user ID, Name, mobile phone number, etc. The following is a sample code to obtain user information:
<?php $appkey = 'your_app_key'; $appsecret = 'your_app_secret'; $authCode = 'user_auth_code'; $requestData = [ 'method' => 'dingtalk.user.get', 'format' => 'json', 'access_token' => '', 'code' => $authCode ]; $userInfoUrl = 'https://oapi.dingtalk.com/user/getuserinfo'; $userInfoUrl .= '?corpid=' . $appkey; $userInfoUrl .= '&corpsecret=' . $appsecret; $userInfoUrl .= '&code=' . $authCode; $response = file_get_contents($userInfoUrl); $userInfo = json_decode($response, true); if ($userInfo && $userInfo['errcode'] == 0) { $userId = $userInfo['userid']; $name = $userInfo['name']; $mobile = $userInfo['mobile']; // 处理获取到的用户信息 } else { // 处理错误情况 }
- Create Customer
In the customer management application, we need to provide a function to create customers. The following is a sample code for creating a customer:
<?php $appkey = 'your_app_key'; $appsecret = 'your_app_secret'; // 获取access_token的代码省略 $requestData = [ 'method' => 'dingtalk.crm.customer.create', 'format' => 'json', 'access_token' => '', 'userid' => 'user_id', 'name' => 'customer_name', 'mobile' => 'customer_mobile', // 其他客户信息字段 ]; $createCustomerUrl = 'https://oapi.dingtalk.com/topapi/crm/{api_name}'; $response = file_get_contents($createCustomerUrl, false, $requestData); $customerInfo = json_decode($response, true); if ($customerInfo && $customerInfo['errcode'] == 0) { // 处理创建成功的情况 } else { // 处理错误情况 }
Through the above steps, we can use the DingTalk interface and PHP to develop a simple customer management application. Of course, this is just an example. In actual development, more functions and business logic need to be developed according to specific needs. I hope this article can help you understand the customer management application development of DingTalk interface and PHP!
The above is the detailed content of DingTalk Interface and PHP Customer Management Application Development Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


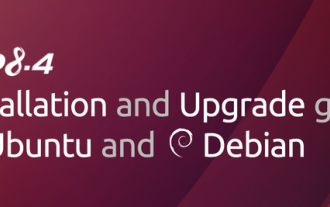
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
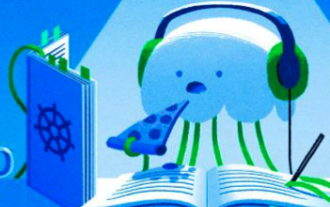
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
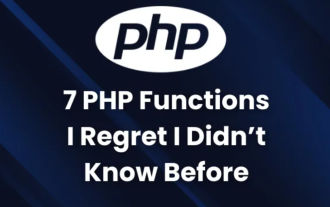
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
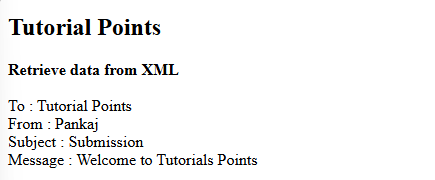
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
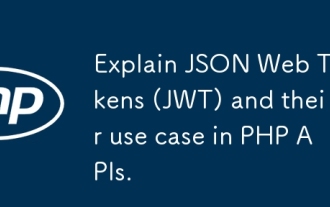
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
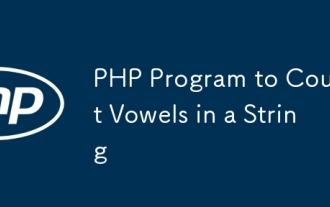
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
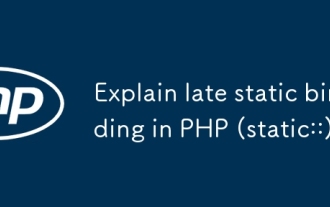
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
