


How to develop best practices for defending against malicious code insertion attacks using PHP and Vue.js
How to develop best practices for defending against malicious code insertion attacks using PHP and Vue.js
Malicious code insertion attacks are a common cybersecurity threat in which attackers insert malicious code into an application. Obtain a user's sensitive information or take control of a user's device. To protect user security and data integrity, developers need to adopt some best practices during application development to defend against malicious code insertion attacks. This article will introduce some best practices that need to be followed when developing applications using PHP and Vue.js, and give relevant code examples.
1. Use PHP to filter and verify user input
When developing applications, user input is the most vulnerable link, so user input needs to be filtered and verified. PHP provides many filtering and validation functions, and developers can choose the appropriate function to use based on business needs. The following are some common user input filtering and validation methods:
- Use the htmlspecialchars() function to HTML escape user input to prevent malicious code execution.
$input = $_POST['username']; $filteredInput = htmlspecialchars($input);
- Use the preg_match() function to perform regular expression matching on user input to limit the format and content of the input.
$input = $_POST['email']; $pattern = '/^w+([.-]?w+)*@w+([.-]?w+)*(.w{2,3})+$/'; if (preg_match($pattern, $input)) { // 邮箱格式正确 } else { // 邮箱格式错误 }
2. Use precompiled statements and parameterized queries to process database queries
Database queries are another vulnerable link. Developers can use precompiled statements and parameters. ized queries to prevent SQL injection attacks. The following is an example of using PDO precompiled statements and parameterized queries:
$pdo = new PDO("mysql:host=localhost;dbname=mydb", $username, $password); $stmt = $pdo->prepare("SELECT * FROM users WHERE username = :username AND password = :password"); $stmt->bindParam(':username', $username); $stmt->bindParam(':password', $password); $stmt->execute();
3. Use Vue.js’s template syntax to prevent XSS attacks
Vue.js is a popular JavaScript Framework that helps developers build interactive and scalable front-end applications. The template syntax of Vue.js can prevent XSS attacks. Developers only need to use double curly brackets "{{ }}" to output dynamic data, and Vue.js will automatically HTML escape the data. The following is an example using Vue.js template syntax:
<div> <p>{{ message }}</p> </div>
4. Use HTTPS and CSRF tokens to prevent authentication and session hijacking
In order to prevent malicious users from obtaining the user’s identity information and For session data, developers need to use HTTPS to protect the security of data transmission and use CSRF tokens to verify whether the requests sent by users are legitimate. Here is an example using a CSRF token:
<form action="/update" method="POST"> <input type="hidden" name="_token" value="{{ csrf_token() }}"> <!--其他表单字段--> <button type="submit">更新</button> </form>
// 后端验证CSRF令牌 if ($_POST['_token'] !== $_SESSION['csrf_token']) { // 无效的CSRF令牌 }
Summary
Malicious code insertion attacks pose a threat to both the security of the application and the confidentiality of user data. When developing applications using PHP and Vue.js, developers need to adopt some best practices to defend against malicious code insertion attacks. This article describes some common defenses and provides corresponding code examples. Developers can choose the appropriate defense method to protect the security of their applications based on the specific situation.
The above is the detailed content of How to develop best practices for defending against malicious code insertion attacks using PHP and Vue.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


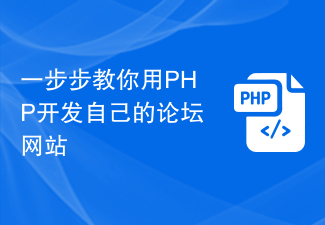
With the rapid development of the Internet and people's increasing demand for information exchange, forum websites have become a common online social platform. Developing a forum website of your own can not only meet your own personalized needs, but also provide a platform for communication and sharing, benefiting more people. This article will teach you step by step how to use PHP to develop your own forum website. I hope it will be helpful to beginners. First, we need to clarify some basic concepts and preparations. PHP (HypertextPreproces
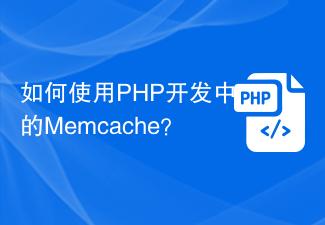
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
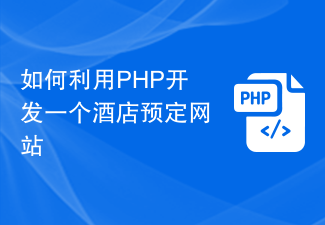
How to use PHP to develop a hotel booking website With the development of the Internet, more and more people are beginning to arrange their travels through online booking. As one of the common online booking services, hotel booking websites provide users with a convenient and fast way to book hotels. This article will introduce how to use PHP to develop a hotel reservation website, allowing you to quickly build and operate your own online hotel reservation platform. 1. System requirements analysis Before starting development, we need to conduct system requirements analysis first to clarify what the website we want to develop needs to have.
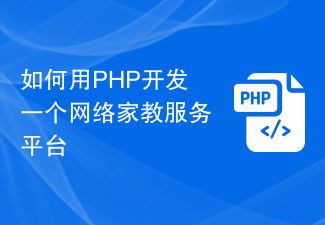
How to use PHP to develop an online tutoring service platform. With the rapid development of the Internet, online tutoring service platforms have attracted more and more people's attention and demand. Parents and students can easily find suitable tutors through such a platform, and tutors can also better demonstrate their teaching abilities and advantages. This article will introduce how to use PHP to develop an online tutoring service platform. First, we need to clarify the functional requirements of the platform. An online tutoring service platform needs to have the following basic functions: Registration and login system: users can
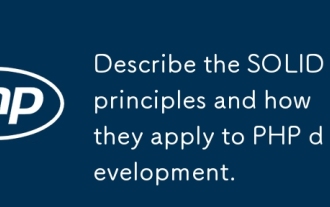
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
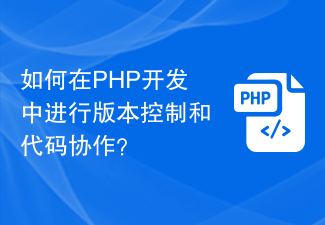
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
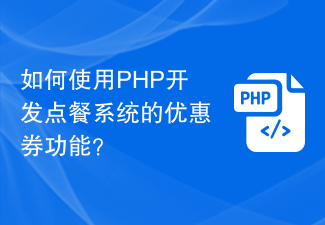
How to use PHP to develop the coupon function of the ordering system? With the rapid development of modern society, people's life pace is getting faster and faster, and more and more people choose to eat out. The emergence of the ordering system has greatly improved the efficiency and convenience of customers' ordering. As a marketing tool to attract customers, the coupon function is also widely used in various ordering systems. So how to use PHP to develop the coupon function of the ordering system? 1. Database design First, we need to design a database to store coupon-related data. It is recommended to create two tables: one
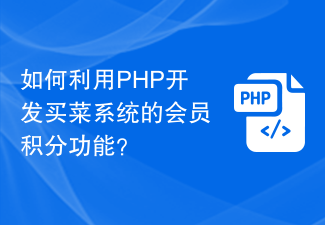
How to use PHP to develop the member points function of the grocery shopping system? With the rise of e-commerce, more and more people choose to purchase daily necessities online, including grocery shopping. The grocery shopping system has become the first choice for many people, and one of its important features is the membership points system. The membership points system can attract users and increase their loyalty, while also providing users with an additional shopping experience. In this article, we will discuss how to use PHP to develop the membership points function of the grocery shopping system. First, we need to create a membership table to store users
