


Common challenges and solutions in PHP Huawei Cloud API interface docking practice
Common challenges and solutions in PHP Huawei Cloud API interface docking practice
With the rapid development of cloud computing, more and more enterprises have begun to migrate their business to the cloud, and Huawei Cloud, as a domestic cloud One of the leading companies in computing, the API interface docking service it provides is also used by many developers. However, in practice, we will encounter some common challenges, such as interface authentication, parameter passing, and error handling. This article will discuss these challenges and provide corresponding solutions to help developers better connect PHP Huawei Cloud API interfaces.
1. Interface Authentication
Before using the Huawei Cloud API interface, you first need to authenticate to ensure the legitimacy of the request. Common authentication methods include Huawei Cloud AccessKey authentication and OAuth2.0 authentication. The PHP implementation of these two authentication methods is introduced below.
1.1 AccessKey Authentication
The AccessKey authentication method is the most basic method for connecting to the Huawei Cloud API interface. AccessKeyId and AccessKeySecret need to be passed to the API interface for verification. The following is a sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
|
1.2 OAuth2.0 authentication
OAuth2.0 authentication method is a token-based authentication method, which is more secure than the AccessKey authentication method. The following is a sample code that demonstrates how to use PHP to implement OAuth2.0 authentication:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
|
2. Passing of parameters
When connecting to API interfaces, it is very important to pass parameters correctly. Some API interfaces need to pass complex parameter structures, such as arrays or nested JSON objects. The following are some common parameter passing situations and their sample codes:
2.1 Passing array parameters
Some API interfaces need to pass array parameters, such as querying multiple resources. The following is a sample code that demonstrates how to pass array parameters:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
2.2 Passing nested JSON objects
Some API interfaces need to pass nested JSON object parameters, such as creating resources. The following is a sample code that demonstrates how to pass nested JSON object parameters:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
3. Error handling
When connecting to API interfaces, we must correctly handle possible errors. The Huawei Cloud API interface will return various error codes and error messages, and we need to handle them accordingly based on the error codes. The following is a sample code that demonstrates how to handle the error information returned by the Huawei Cloud API interface:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
|
Summary:
This article introduces common challenges and corresponding solutions in the practice of PHP Huawei Cloud API interface docking . By correctly performing authentication, parameter passing, and error handling, developers can better use Huawei Cloud's API interfaces to achieve more efficient and secure cloud services. I hope this article can provide some help to developers when connecting the PHP Huawei Cloud API interface.
The above is the detailed content of Common challenges and solutions in PHP Huawei Cloud API interface docking practice. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




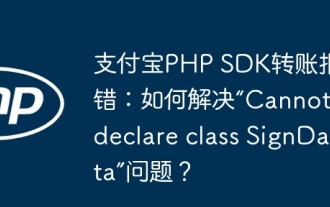
Alipay PHP...
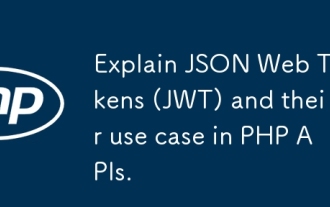
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
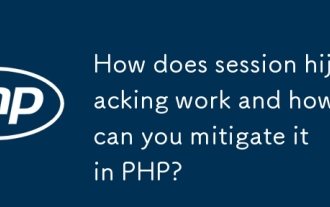
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
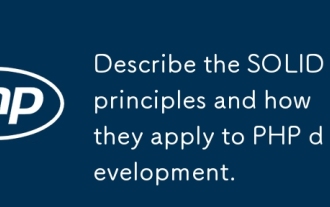
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
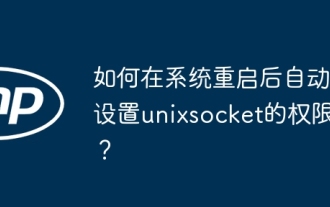
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
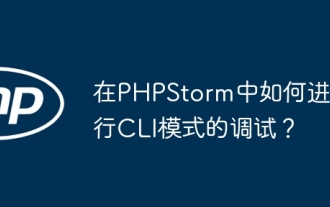
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
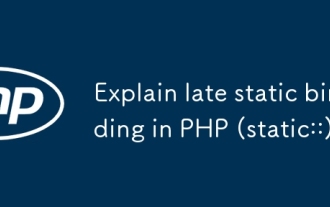
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
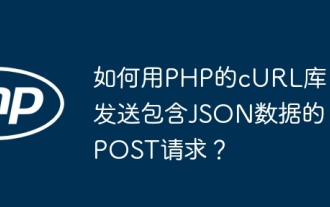
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
