


How to use PHP and Youpai Cloud API to implement live video streaming
How to use PHP and Youpai Cloud API to implement live video function
Nowadays, with the continuous development and popularization of network technology, live video has become one of the important ways for people to obtain information and entertainment. . As a scripting language widely used in web development, PHP, combined with Youpaiyun API, can help us easily implement the function of live video streaming.
Youpaiyun is a well-known cloud storage and content distribution network (CDN) service provider that provides related functions and APIs for live video broadcasts. Its services are stable and reliable and its technical support is complete, making it very suitable for building videos. Live streaming platform.
This article will introduce how to use PHP and Youpai Cloud API to implement video live broadcast functions, including creating channels, generating push address, pushing and playing videos, etc. The following are specific steps and code examples:
- Register Youpaiyun account and obtain API key
First, you need to register an account on Youpaiyun official website and obtain API key. The API key is an important credential for calling Youpai Cloud API, ensuring it is used correctly in the code. -
Create channel
On the live broadcast platform, we first need to create a channel to host the live video stream. Channel creation can be completed by calling Youpaiyun's API.<?php $apiUrl = 'https://api.upyun.com/'; $apiKey = 'YOUR_API_KEY'; $apiSecret = 'YOUR_API_SECRET'; // 请求参数 $params = [ 'service' => 'live', 'type' => 'push', 'app' => 'live', 'name' => 'channel_name' ]; // 生成签名 $sign = md5(json_encode($params) . $apiSecret); // 发送请求 $response = file_get_contents($apiUrl . 'create/channels?' . http_build_query($params) . '&sign=' . $sign); // 显示返回结果 echo $response; ?>
Copy after loginIn the above code, you need to replace
YOUR_API_KEY
andYOUR_API_SECRET
with your own API key. Then modifychannel_name
as needed to the name of the channel you want to create. Generate push address
After the channel is successfully created, we need to generate a push address to push the video stream to Youpaiyun's server. Similarly, we can achieve this by calling Youpaiyun's API.<?php $apiUrl = 'https://api.upyun.com/'; $apiKey = 'YOUR_API_KEY'; $apiSecret = 'YOUR_API_SECRET'; // 请求参数 $params = [ 'service' => 'live', 'type' => 'push', 'app' => 'live', 'name' => 'channel_name', 'expire' => time() + 3600, // 过期时间设定为1小时后 'nonce' => uniqid() // 随机字符串,用于防止重放攻击 ]; // 生成签名 $sign = md5(json_encode($params) . $apiSecret); // 发送请求 $response = file_get_contents($apiUrl . 'get/streamUrls?' . http_build_query($params) . '&sign=' . $sign); // 解析返回结果 $result = json_decode($response, true); // 显示推流地址 echo $result['data']['rtmp']; ?>
Copy after loginAlso need to replace
YOUR_API_KEY
andYOUR_API_SECRET
with your own API key. Modifychannel_name
to the channel name you created previously.Pushing and playing videos
After obtaining the push address, we can use any video push tool to push, such as OBS, etc. To play videos, you can use the HTML5<video>
tag.<?php $rtmpUrl = 'YOUR_RTMP_URL'; // 替换为你自己的推流地址 ?> <!-- 推流 --> <object width="640" height="480"> <param name="movie" value="player.swf"/> <param name="allowFullScreen" value="true"/> <param name="flashvars" value="rtmp_url=<?php echo $rtmpUrl; ?>"/> <object type="application/x-shockwave-flash" data="player.swf" width="640" height="480"> <param name="allowFullScreen" value="true"/> <param name="flashvars" value="rtmp_url=<?php echo $rtmpUrl; ?>"/> <div> <h1>视频播放需要启用Flash Player,请点击 <a href="http://www.adobe.com/go/getflashplayer">这里</a>下载最新版本。</h1> </div> </object> </object> <!-- 播放 --> <video width="640" height="480" controls> <source src="<?php echo $rtmpUrl; ?>" type="video/rtmp"> 您的浏览器不支持HTML5 video标签。 </video>
Copy after loginReplace
YOUR_RTMP_URL
with the push address you obtained before.
Through the above steps, we have successfully implemented the video live broadcast function using PHP and Youpai Cloud API. Of course, this is just a simple example and you can extend and improve the code according to your needs. I hope this article will be helpful to your development work!
The above is the detailed content of How to use PHP and Youpai Cloud API to implement live video streaming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
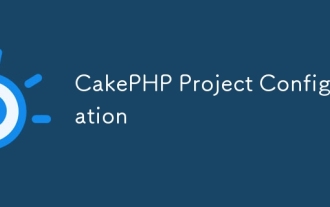
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
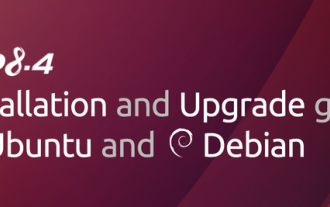
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
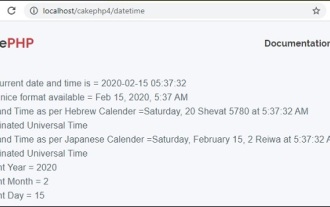
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
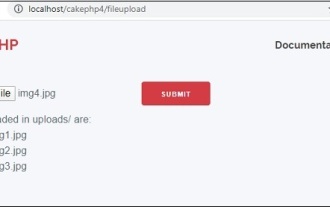
To work on file upload we are going to use the form helper. Here, is an example for file upload.
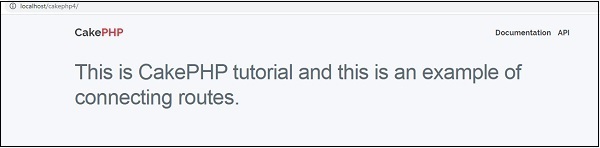
In this chapter, we are going to learn the following topics related to routing ?
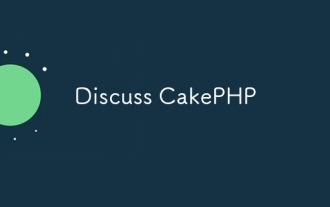
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
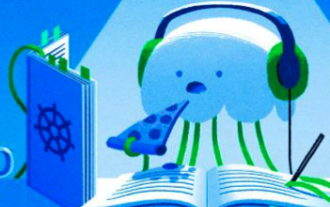
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
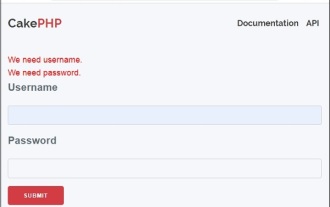
Validator can be created by adding the following two lines in the controller.
