


How to implement data push and message notification using PHP and UniApp
How PHP and UniApp implement data push and message notification
With the rapid development of the mobile Internet, message push and notification functions have attracted more and more attention from developers. In web development, PHP is a very commonly used server-side programming language, and UniApp is a cross-platform development framework based on Vue.js. This article will introduce how to use PHP and UniApp to implement data push and message notification.
1. Use PHP to push data
In PHP, you can use the CURL library to send HTTP requests to push data. The following is a sample code that uses PHP to send a POST request:
<?php $url = 'http://your_server_url/data_push.php'; $data = array( 'key1' => 'value1', 'key2' => 'value2' ); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_POST, 1); curl_setopt($curl, CURLOPT_POSTFIELDS, http_build_query($data)); curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); $response = curl_exec($curl); curl_close($curl); echo $response; ?>
In the above code, $url
is the target address of the data push, and $data
is the target address of the data push. The pushed data can be modified according to actual needs. Use the curl_setopt
function to set request options, where CURLOPT_URL
is used to set the target URL, CURLOPT_POST
indicates using POST request, CURLOPT_POSTFIELDS
is to send The data, CURLOPT_RETURNTRANSFER
means returning the response instead of outputting it directly to the browser. After sending the request, you can use curl_exec
to get the server's response.
2. Use UniApp to implement message notifications
UniApp is a development framework that can be developed quickly on multiple platforms and can support pushing messages to Android and iOS devices. The following is a sample code for using UniApp to implement message notification:
uni.request({ url: 'http://your_server_url/message_push.php', method: 'POST', data: { title: '消息标题', content: '消息内容' }, success: function(res) { console.log('消息推送成功'); }, fail: function(err) { console.log('消息推送失败:' + JSON.stringify(err)); } });
In the above code, url
is the target address of message push, and data
is the destination address to be pushed. The message content can be modified according to actual needs. Use uni.request
to send an HTTP request, where url
represents the target URL, method
represents the request method, data
is the data to be sent, success
is the callback function if the request is successful, fail
is the callback function if the request fails. After sending the request, you can handle it accordingly based on the returned results.
3. Integration of PHP and UniApp
In order to integrate data push and message notification, the above code can be merged into one file. The following is a sample code that integrates PHP and UniApp:
<?php $url = 'http://your_server_url/data_push.php'; $data = array( 'key1' => 'value1', 'key2' => 'value2' ); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_POST, 1); curl_setopt($curl, CURLOPT_POSTFIELDS, http_build_query($data)); curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); $response = curl_exec($curl); curl_close($curl); echo $response; ?> <script> uni.request({ url: 'http://your_server_url/message_push.php', method: 'POST', data: { title: '消息标题', content: '消息内容' }, success: function(res) { console.log('消息推送成功'); }, fail: function(err) { console.log('消息推送失败:' + JSON.stringify(err)); } }); </script>
In the above code, PHP is first used to send a data push request, and then UniApp is used to send a message push request. By merging the two pieces of code into one file, the integration of data push and message notification can be achieved and completed in the same request.
Summary:
This article introduces how to use PHP and UniApp to implement data push and message notification. In PHP, you can use the CURL library to send HTTP requests to push data, and in UniApp, you can use the uni.request method to implement message notifications. By integrating PHP and UniApp codes, data push and message notification can be integrated. Developers can make appropriate modifications and extensions according to their own needs to achieve more functional push and notifications.
The above is the detailed content of How to implement data push and message notification using PHP and UniApp. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


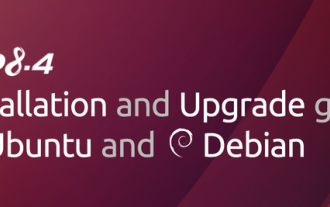
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
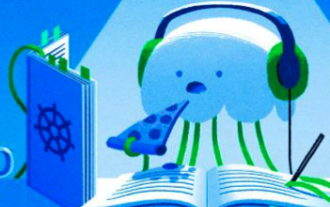
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
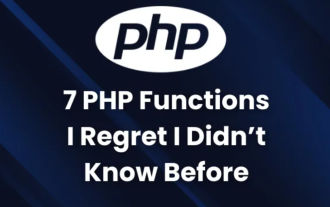
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
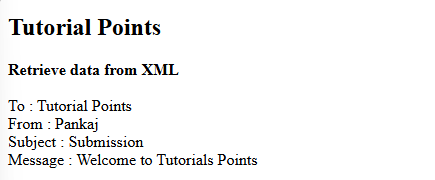
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
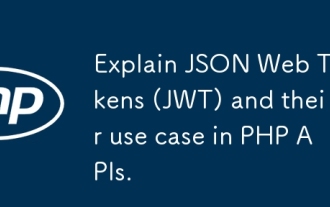
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
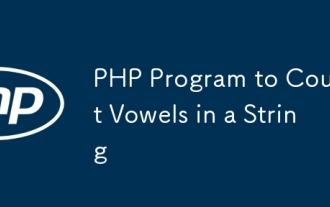
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
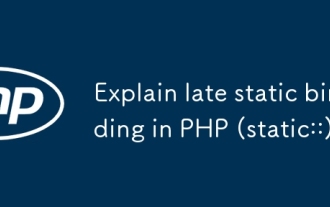
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
