


Teach you how to use PHP and Vue.js to develop applications that prevent sensitive data tampering
Teach you how to use PHP and Vue.js to develop applications that protect against sensitive data tampering
In order to protect sensitive data from malicious tampering, it is very important to develop a powerful application. In this article, I will teach you how to use PHP and Vue.js to develop a defensive application that protects your data from the risk of tampering.
1. Background knowledge
Before we begin, let us first understand some basic concepts.
- PHP
PHP is an open source server-side scripting language that can be mixed with HTML to create dynamic web pages. We will use PHP to write server-side code to process and validate user-submitted data. - Vue.js
Vue.js is a popular JavaScript framework for building user interfaces. We will use Vue.js to build a responsive front-end application for rendering and displaying data. - Data Storage and Transfer
We will use MySQL as our database and PHP to interact with the database. In order to ensure the security of data transmission, we will use HTTPS to encrypt data transmission.
2. Create a database table
First, we need to create a database table to store our sensitive data. Create a database named "sensitive_data" and create a table named "users" in it to store users' sensitive data. The table structure is as follows:
CREATE TABLE `users` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(255) NOT NULL, `email` varchar(255) NOT NULL, `password` varchar(255) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
3. Configure PHP and Vue.js
- PHP configuration
In the PHP configuration, we will include the database connection information , and write some functions for processing and validating data. Create a file called "config.php" and paste the following code into it:
<?php // 数据库连接信息 define('DB_HOST', 'localhost'); define('DB_NAME', 'sensitive_data'); define('DB_USER', 'your_username'); define('DB_PASSWORD', 'your_password'); // 连接到数据库 $conn = mysqli_connect(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME); // 验证用户输入的函数 function validateInput($input) { // 执行你的验证逻辑,确保数据的完整性和正确性 } // 插入数据到数据库函数 function insertData($name, $email, $password) { global $conn; $stmt = $conn->prepare("INSERT INTO users (name, email, password) VALUES (?, ?, ?)"); $stmt->bind_param("sss", $name, $email, $password); if ($stmt->execute()) { return true; } else { return false; } } ?>
- Vue.js Configuration
In the Vue.js configuration, We will use the axios library to send requests to the server and Vue.js components to render and process the data. Create a file called "app.js" and paste the following code into it:
import Vue from 'vue'; import axios from 'axios'; // 执行一些全局配置,例如设置axios的默认baseURL等 axios.defaults.baseURL = 'http://localhost:8888'; Vue.prototype.$http = axios; new Vue({ el: '#app', data: { name: '', email: '', password: '' }, methods: { submitForm() { this.$http.post('/saveData.php', { name: this.name, email: this.email, password: this.password }) .then(response => { console.log(response); // 在这里可以处理服务器的响应,例如显示成功消息等 }) .catch(error => { console.log(error); }); } } });
4. Write PHP and Vue.js code
Now, we have configured PHP and Vue.js, we can start writing real code.
- PHP Code
Create a file called "saveData.php" and paste the following code into it:
<?php require_once 'config.php'; $data = $_POST; // 对用户输入进行验证 if (!validateInput($data['name']) || !validateInput($data['email']) || !validateInput($data['password'])) { $response = array( 'status' => 'error', 'message' => 'Invalid input data' ); } else { // 插入数据到数据库 $name = $data['name']; $email = $data['email']; $password = $data['password']; if (insertData($name, $email, $password)) { $response = array( 'status' => 'success', 'message' => 'Data saved successfully' ); } else { $response = array( 'status' => 'error', 'message' => 'Failed to save data' ); } } header('Content-Type: application/json'); echo json_encode($response); ?>
- Vue.js code
In the Vue.js code, we will use the form and input components to receive and process user input. Create a file called "index.html" and paste the following code into it:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>防御敏感数据篡改的应用程序</title> </head> <body> <div id="app"> <form @submit.prevent="submitForm"> <label for="name">Name</label> <input type="text" id="name" v-model="name"> <label for="email">Email</label> <input type="email" id="email" v-model="email"> <label for="password">Password</label> <input type="password" id="password" v-model="password"> <button type="submit">Save</button> </form> </div> <script src="https://cdn.jsdelivr.net/npm/vue"></script> <script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script> <script src="app.js"></script> </body> </html>
5. Run the application
Now that we have completed the development of the application, we The application can be launched by running the following command in the terminal:
php -S localhost:8888
Then, access the application by visiting "http://localhost:8888" in the browser.
6. Summary
It is not difficult to develop an application with the function of preventing sensitive data tampering using PHP and Vue.js. By using appropriate validation rules and ensuring secure transmission and storage of data, we can ensure our sensitive data is protected from the risk of tampering.
In this article, we learned how to develop a basic application using PHP and Vue.js, and provided PHP and Vue.js code examples for reference. I hope this tutorial is helpful to you, and I wish you successful development!
The above is the detailed content of Teach you how to use PHP and Vue.js to develop applications that prevent sensitive data tampering. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


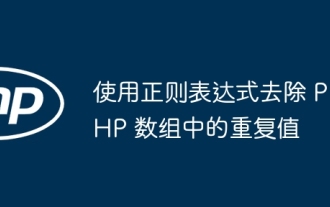
How to remove duplicate values from PHP array using regular expressions: Use regular expression /(.*)(.+)/i to match and replace duplicates. Iterate through the array elements and check for matches using preg_match. If it matches, skip the value; otherwise, add it to a new array with no duplicate values.
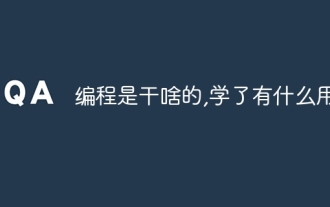
1. Programming can be used to develop various software and applications, including websites, mobile applications, games, and data analysis tools. Its application fields are very wide, covering almost all industries, including scientific research, health care, finance, education, entertainment, etc. 2. Learning programming can help us improve our problem-solving skills and logical thinking skills. During programming, we need to analyze and understand problems, find solutions, and translate them into code. This way of thinking can cultivate our analytical and abstract abilities and improve our ability to solve practical problems.
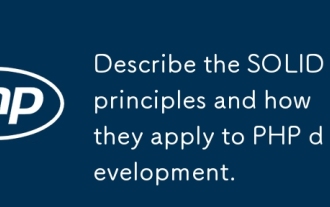
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
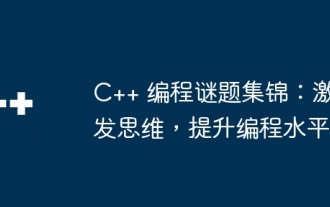
C++ programming puzzles cover algorithm and data structure concepts such as Fibonacci sequence, factorial, Hamming distance, maximum and minimum values of arrays, etc. By solving these puzzles, you can consolidate C++ knowledge and improve algorithm understanding and programming skills.
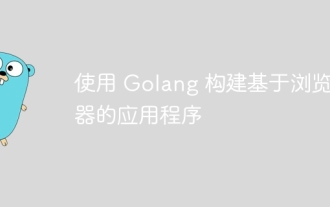
Build browser-based applications with Golang Golang combines with JavaScript to build dynamic front-end experiences. Install Golang: Visit https://golang.org/doc/install. Set up a Golang project: Create a file called main.go. Using GorillaWebToolkit: Add GorillaWebToolkit code to handle HTTP requests. Create HTML template: Create index.html in the templates subdirectory, which is the main template.
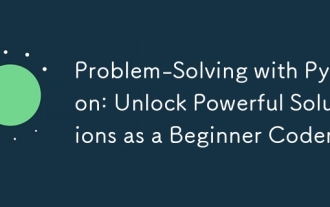
Pythonempowersbeginnersinproblem-solving.Itsuser-friendlysyntax,extensivelibrary,andfeaturessuchasvariables,conditionalstatements,andloopsenableefficientcodedevelopment.Frommanagingdatatocontrollingprogramflowandperformingrepetitivetasks,Pythonprovid
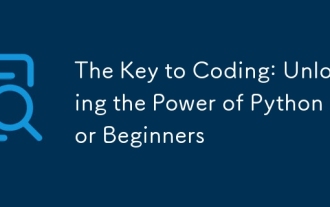
Python is an ideal programming introduction language for beginners through its ease of learning and powerful features. Its basics include: Variables: used to store data (numbers, strings, lists, etc.). Data type: Defines the type of data in the variable (integer, floating point, etc.). Operators: used for mathematical operations and comparisons. Control flow: Control the flow of code execution (conditional statements, loops).
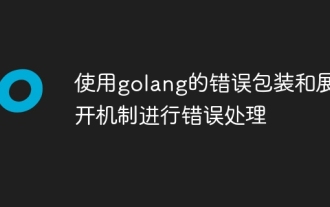
Error handling in Go includes wrapping errors and unwrapping errors. Wrapping errors allows one error type to be wrapped with another, providing a richer context for the error. Expand errors and traverse the nested error chain to find the lowest-level error for easy debugging. By combining these two technologies, error conditions can be effectively handled, providing richer error context and better debugging capabilities.
