How to implement payment processing using PHP Amazon API
How to use PHP Amazon API to implement payment processing
Amazon is a world-renowned e-commerce platform with a huge inventory of goods and millions of users. To facilitate developers to integrate Amazon's payment processing capabilities on their websites, Amazon provides a set of simple yet powerful APIs.
This article will introduce how to use PHP Amazon API to implement payment processing. First, we need to create an Amazon developer account and obtain the corresponding API key.
Step 1: Install and configure AWS PHP SDK
First, we need to install the AWS PHP SDK and introduce the library files provided by the SDK into the code. You can install the SDK through Composer, or download the compressed package from the official website and extract it into the project.
After the installation is complete, we need to introduce the SDK's automatic loading file into the code in order to use the functions provided by the SDK.
require 'aws/aws-autoloader.php';
Step 2: Initialize AWS SDK
Before starting to use the API, we need to initialize the AWS SDK and set related configuration options.
use AwsCommonAws; // 创建一个新的AWS实例 $aws = Aws::factory(array( 'key' => 'YOUR_AWS_ACCESS_KEY', 'secret' => 'YOUR_AWS_SECRET_ACCESS_KEY', 'region' => 'us-west-2' // 根据自己的需求设置区域 ));
Step 3: Create an Amazon Payment Object
Next, we need to create an Amazon Payment object in order to use the payment processing functionality provided by Amazon.
// 创建Pay对象 $payClient = $aws->get('Pay');
Step 4: Create an order
Before doing any payment operation, we need to create an order first.
// 创建一个新订单 $orderRequest = array( 'amount' => '100.00', // 订单总金额 'currencyCode' => 'USD', // 货币代码 'merchantOrderId' => '123456', // 商户订单号 // 其他订单相关信息,如收货地址,商品信息等 ); // 发送创建订单请求 $response = $payClient->createOrder($orderRequest);
The return value of the create order request contains the order number generated by Amazon, which we can save in the database for subsequent use.
Step 5: Process Payment
Once the order is successfully created, we can use the payment functionality provided by Amazon to process our payment.
// 创建支付请求 $paymentRequest = array( 'orderId' => 'AMAZON_ORDER_ID', // 亚马逊生成的订单号 'amount' => '100.00', // 支付金额 'currencyCode' => 'USD', // 货币代码 // 其他支付相关信息,如卡号,过期日期等 ); // 发送支付请求 $response = $payClient->authorize($paymentRequest);
After sending a payment request through the authorize method, Amazon will verify the payment information and try to deduct the corresponding amount from the buyer's payment account.
Step 6: Process the payment result
After the payment request is sent successfully, we can obtain the payment result by checking $response['status']. If the value of $status is "Pending", it means the payment is being processed; if the value is "Open", it means the payment was successful; if the value is "Closed", it means the payment was cancelled.
Based on the payment results, we can perform corresponding processing operations, such as updating order status, sending confirmation emails, etc.
Summary
This article explains how to implement payment processing using the PHP Amazon API. By installing and configuring AWS PHP SDK, initializing AWS SDK, creating Amazon payment objects, creating orders, sending payment requests, processing payment results and other steps, we can easily implement Amazon's payment processing function in our website. Of course, in practical applications, more security and exception handling mechanisms need to be considered to ensure the stability and security of the payment process.
The above is the detailed content of How to implement payment processing using PHP Amazon API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
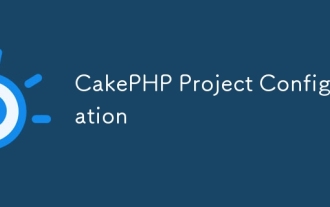
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
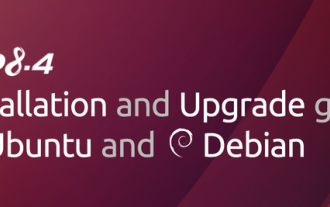
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
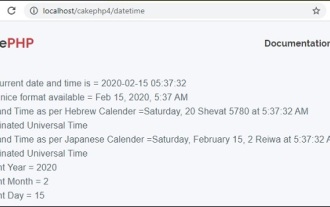
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
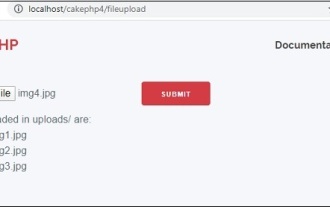
To work on file upload we are going to use the form helper. Here, is an example for file upload.
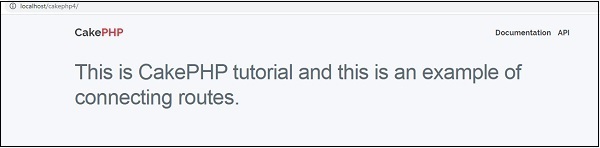
In this chapter, we are going to learn the following topics related to routing ?
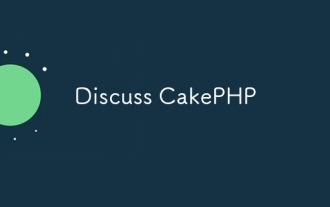
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
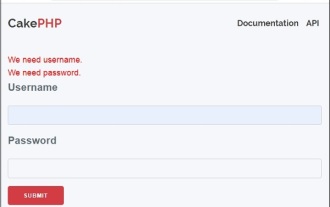
Validator can be created by adding the following two lines in the controller.
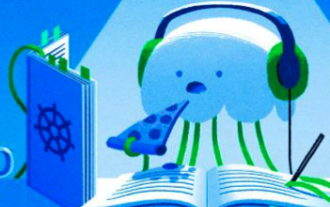
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
