


PHP and WebDriver Extensions: How to implement detection and assertion of page elements
PHP and WebDriver extensions: How to implement detection and assertion of page elements
Introduction: With the increase of Web applications, automated testing has become more and more important. During testing, we need to verify that elements on the page exist and that their state is as expected. This article will introduce how to use PHP and WebDriver extensions to implement detection and assertion of page elements.
1. Preparation
Before starting, we need to ensure that the following environment has been configured:
- Install PHP and Composer
-
Install WebDriver Extension
Execute the following command in the terminal to install the WebDriver extension:$ composer require facebook/webdriver
Copy after login Run the WebDriver server
Execute the following command in the terminal to start the WebDriver server:$ java -jar selenium-server-standalone-x.xx.x.jar
Copy after loginAmong them,
x.xx.x
is the version number of the WebDriver server.
2. Detection of page elements
Initializing WebDriver
First, we need to import the Webdriver class and initialize the WebDriver instance. Add the following code to your test file:<?php require_once('vendor/autoload.php'); use FacebookWebDriverRemoteDesiredCapabilities; use FacebookWebDriverRemoteRemoteWebDriver; use FacebookWebDriverWebDriverBy; $host = 'http://localhost:4444/wd/hub'; // WebDriver服务器地址 $capabilities = DesiredCapabilities::chrome(); // 选择浏览器 $driver = RemoteWebDriver::create($host, $capabilities);
Copy after loginOpen URL
Use the$driver->get()
method to open the URL to be tested. For example:$driver->get('https://www.example.com');
Copy after loginDetect whether an element exists
Use the$driver->findElement()
method to detect whether an element exists based on its selector. If the element exists, this method will return aWebDriverElement
object, otherwise it will throw aNoSuchElementException
exception. The following example detects whether an element with the idelementId
exists on the page:try { $element = $driver->findElement(WebDriverBy::id('elementId')); echo "元素存在"; } catch (Exception $e) { echo "元素不存在"; }
Copy after loginAssert the text content of the element
Use$webElement-> The getText()
method gets the text content of the element. For example, we can assert whether the text content of an element is "Hello, World!":$webElement = $driver->findElement(WebDriverBy::id('elementId')); $text = $webElement->getText(); assert($text == 'Hello, World!', '元素文本内容不符合预期');
Copy after loginAsserting the element's attribute value
Use$webElement->getAttribute( )
method to get the attribute value of the element. For example, we can assert whether thehref
attribute value of a link is "https://www.example.com":$webElement = $driver->findElement(WebDriverBy::tagName('a')); $href = $webElement->getAttribute('href'); assert($href == 'https://www.example.com', '链接的href属性值不符合预期');
Copy after login
3. Summary
By using PHP and WebDriver extensions, we can easily implement detection and assertion of page elements. This is a very important step in automated testing as it helps us verify that the elements on the page are present and that they behave as expected.
The above is the entire content of this article. I hope this article can help you improve the efficiency of automated testing. If you have any questions please feel free to leave a message. thanks for reading!
The above is the detailed content of PHP and WebDriver Extensions: How to implement detection and assertion of page elements. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


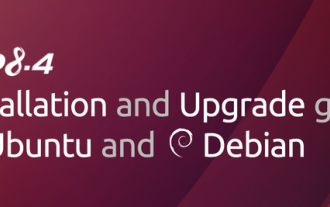
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
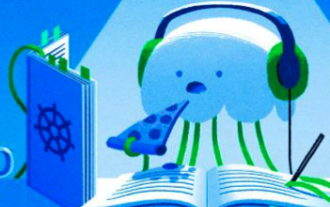
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
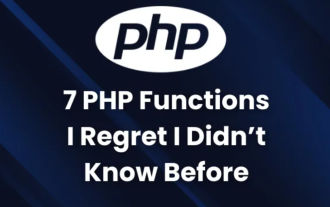
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
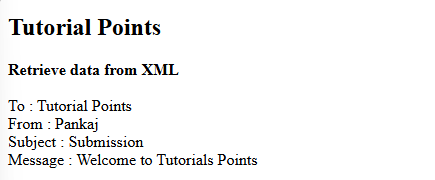
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
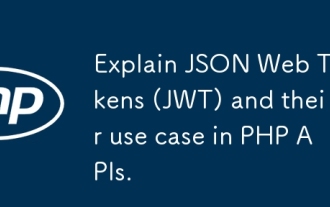
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
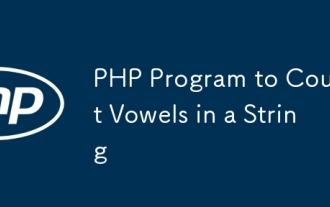
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
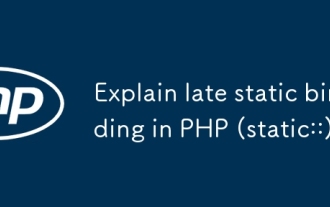
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
