How PHP uses MongoDB to implement data preprocessing
How PHP uses MongoDB to implement data preprocessing
Introduction
In PHP development, MongoDB is a very popular NoSQL database. It has high scalability, flexible data model and powerful query performance. When using MongoDB to store and query data, we usually need to perform some preprocessing on the data to ensure data integrity and consistency. This article will introduce how to use PHP and MongoDB for data preprocessing and provide some sample code.
Install MongoDB extension
Before using MongoDB, we need to install the MongoDB extension first. You can install the MongoDB extension in PHP by following the steps below:
- Download MongoDB extension
Visit the https://pecl.php.net/package/mongodb website to download the latest version of the MongoDB extension. - Unzip the extension and enter the directory
Unzip the downloaded expansion package and enter the unzipped directory. -
Compile and install the extension
Run the following command in the terminal to compile and install the extension:phpize ./configure make sudo make install
Copy after login Configure the PHP.ini file
Open php.ini file and add the following lines:extension=mongodb.so
Copy after login- Restart the web server
Restart the web server for the changes to take effect.
Connecting to MongoDB database
Before data preprocessing, we first need to connect to the MongoDB database. Use the following code example to connect to a local MongoDB database:
<?php $mongoClient = new MongoDBClient("mongodb://localhost:27017"); $database = $mongoClient->selectDatabase('mydb'); echo "Successfully connected to MongoDB"; ?>
Data Preprocessing
Once connected to the MongoDB database, we can perform various data preprocessing tasks. Below are some common data preprocessing operations and their sample code.
Insert data
Use theinsertOne()
orinsertMany()
method to insert data into the MongoDB database. The following is a sample code to insert a single piece of data:<?php $collection = $database->selectCollection('mycollection'); $result = $collection->insertOne([ 'name' => 'John', 'age' => 25, 'email' => 'john@example.com' ]); echo "Successfully inserted document with _id: " . $result->getInsertedId(); ?>
Copy after loginUpdate data
Use theupdateOne()
orupdateMany()
method to Update data in MongoDB. The following is a sample code to update data:<?php $collection = $database->selectCollection('mycollection'); $result = $collection->updateOne( ['name' => 'John'], ['$set' => ['age' => 26]] ); echo "Successfully updated " . $result->getModifiedCount() . " document(s)"; ?>
Copy after loginDelete data
Use thedeleteOne()
ordeleteMany()
method from Delete data in MongoDB. The following is a sample code to delete data:<?php $collection = $database->selectCollection('mycollection'); $result = $collection->deleteOne(['name' => 'John']); echo "Successfully deleted " . $result->getDeletedCount() . " document(s)"; ?>
Copy after loginQuery data
Use thefind()
method to perform query operations in MongoDB. The following is a sample code for querying data:<?php $collection = $database->selectCollection('mycollection'); $cursor = $collection->find(['age' => ['$gt' => 21]]); foreach ($cursor as $document) { echo "Name: " . $document['name'] . ", Age: " . $document['age']; } ?>
Copy after login
Conclusion
Through this article, we learned how to use PHP and MongoDB for data preprocessing. We learned how to install the MongoDB extension, connect to the MongoDB database, and perform common data preprocessing operations. I hope these sample codes can help you better understand and apply MongoDB's preprocessing functions.
The above is the detailed content of How PHP uses MongoDB to implement data preprocessing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
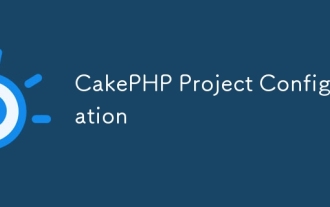
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
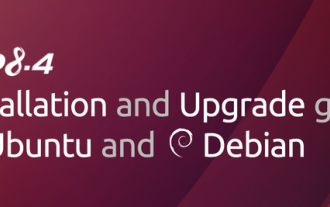
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
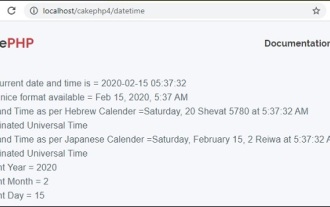
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
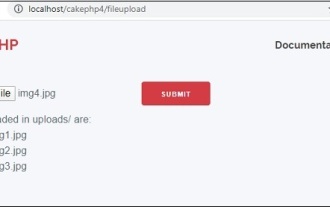
To work on file upload we are going to use the form helper. Here, is an example for file upload.
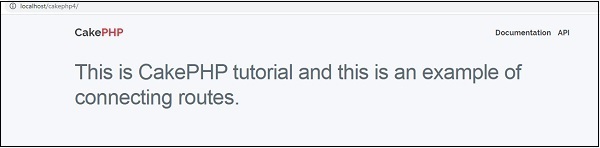
In this chapter, we are going to learn the following topics related to routing ?
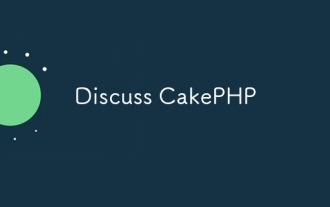
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
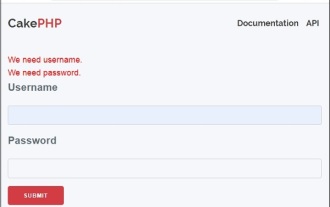
Validator can be created by adding the following two lines in the controller.
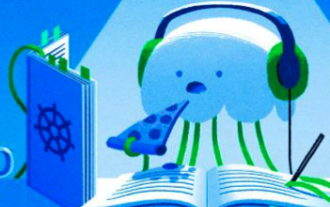
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
