How to implement asynchronous event dispatch in PHP
How to implement asynchronous event dispatch in PHP
Event-driven is a commonly used programming model that can achieve asynchronous processing and better system responsiveness. In PHP, we can use asynchronous event dispatch to handle various events, such as network requests, scheduled tasks, etc. This article will introduce how to use PHP to implement asynchronous event dispatch, with code examples.
- Install dependency packages
First, we need to install some dependency packages to support asynchronous event dispatch. The more commonly used ones are ReactPHP and Swoole. This article uses ReactPHP as an example.
Use the Composer command line tool and execute the following command to install ReactPHP and its related dependency packages:
composer require react/event-loop composer require react/http-client
- Create an event loop
In PHP, We need to use an event loop to implement asynchronous event dispatch. The event loop continuously monitors the occurrence of events and calls the corresponding callback function for processing.
require 'vendor/autoload.php'; $loop = ReactEventLoopFactory::create();
- Register event listener
Next, we need to register the event listener. Event listeners are responsible for listening to specific events and defining corresponding callback functions.
$eventEmitter = new EvenementEventEmitter(); $eventEmitter->on('event1', function () { // 处理event1事件的回调函数 }); $eventEmitter->on('event2', function () { // 处理event2事件的回调函数 });
- Dispatching events
Events can now be dispatched. Use an event dispatcher object to dispatch events. The event dispatcher will look for registered event listeners and call the corresponding callback functions.
$eventEmitter->emit('event1'); $eventEmitter->emit('event2');
- Run the event loop
Finally, we need to run the event loop so that it starts listening for events.
$loop->run();
Complete example:
require 'vendor/autoload.php'; $loop = ReactEventLoopFactory::create(); $eventEmitter = new EvenementEventEmitter(); $eventEmitter->on('event1', function () { echo "处理event1事件 "; }); $eventEmitter->on('event2', function () { echo "处理event2事件 "; }); $eventEmitter->emit('event1'); $eventEmitter->emit('event2'); $loop->run();
The above are the basic steps for using ReactPHP to implement PHP asynchronous event dispatch. By registering event listeners and dispatching events, we can process various tasks asynchronously and improve the system's responsiveness.
Summary:
Asynchronous event dispatch is an efficient programming model that can be easily implemented in PHP using toolkits such as ReactPHP. By splitting tasks into multiple events and using the event loop mechanism for asynchronous processing, the system's concurrent processing capabilities can be improved. I hope this article can help readers better understand and apply asynchronous event dispatching in PHP.
The above is the detailed content of How to implement asynchronous event dispatch in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


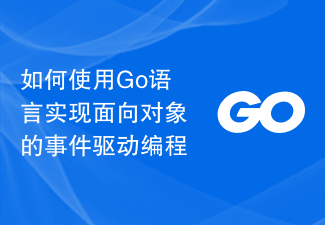
How to use Go language to implement object-oriented event-driven programming Introduction: The object-oriented programming paradigm is widely used in software development, and event-driven programming is a common programming model that realizes the program flow through the triggering and processing of events. control. This article will introduce how to implement object-oriented event-driven programming using Go language and provide code examples. 1. The concept of event-driven programming Event-driven programming is a programming model based on events and messages, which transfers the flow control of the program to the triggering and processing of events. in event driven
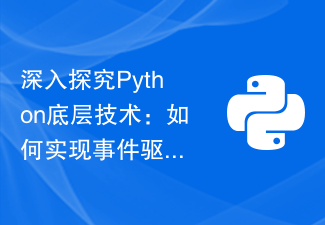
Python is a high-level programming language that is widely used to develop various applications. In the Python programming language, event-driven programming is considered a very efficient programming method. It is a technique for writing event handlers in which program code is executed in the order in which events occur. Principles of Event-Driven Programming Event-driven programming is an application design technique based on event triggers. Event triggers are handled by the event monitoring system. When an event trigger is fired, the event monitoring system calls the application's event handler.
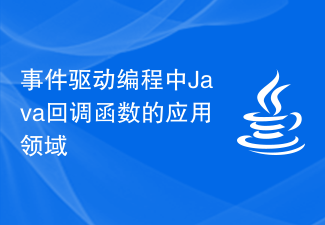
Application of Java callback function in event-driven programming Introduction to callback function A callback function is a function that is called after an event or operation occurs. It is commonly used in event-driven programming, where the program blocks while waiting for an event to occur. When the event occurs, the callback function is called and the program can continue execution. In Java, callback functions can be implemented through interfaces or anonymous inner classes. An interface is a mechanism for defining function signatures that allows one class to implement another class's
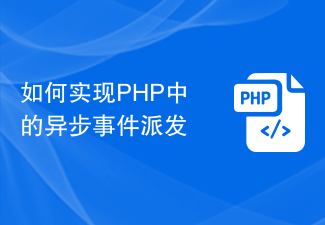
How to implement asynchronous event dispatching in PHP Event-driven is a commonly used programming model that can achieve asynchronous processing and better system responsiveness. In PHP, we can use asynchronous event dispatch to handle various events, such as network requests, scheduled tasks, etc. This article will introduce how to use PHP to implement asynchronous event dispatch, with code examples. Install dependency packages First, we need to install some dependency packages to support asynchronous event dispatch. The more commonly used ones are ReactPHP and Swoole. This article takes ReactPHP as an example
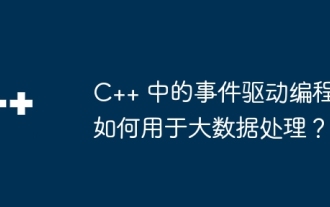
In C++, event-driven programming (EDP) is crucial for big data processing by waiting for events to trigger in the event loop to respond to events without affecting system performance. The C++Boost library provides rich event-driven programming functions, such as Boost.Asio and Boost.Thread, which can be used to handle network connections, file I/O and thread management. For example, EDP can be used to listen to the data stream of a Kafka topic and trigger events when data is received, thereby enabling efficient big data ingestion and processing.
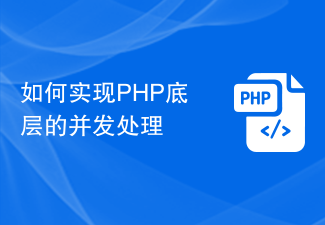
How to implement concurrency processing at the bottom of PHP requires specific code examples. In the process of web development, it is often necessary to handle a large number of concurrent requests. If concurrent processing is not used, problems such as long response times and excessive server pressure will occur. PHP is a language for web development. Its built-in multi-threading support is relatively weak, but it can achieve underlying concurrency processing through other methods. 1. Principle introduction In PHP, each request will be processed by a new process or thread opened by the web server. In order to improve concurrency capabilities, at the bottom
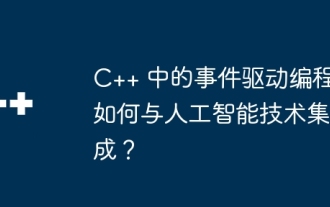
Event-driven programming (EDP) is integrated with artificial intelligence (AI) technology to create responsive AI systems. In the EDP framework, AI models can be registered as event handlers. After an event is triggered, the AI model will perform inference and use event data for classification. The steps are as follows: 1. Create an EDP application with an event loop and callback function. 2. Train the AI image classification model. 3. Instantiate the AI model in the application and register the callback function that is called when the image is available for classification. 4. Wait for image from user or external source in main loop. 5. When an image is received, the event is triggered and the AI model callback function is called for classification. 6. Display the classification results or store them for further processing.
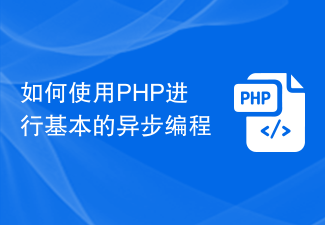
With the continuous development of Internet technology, asynchronous programming has become a basic feature in modern programming language design. Asynchronous programming relies on an event-driven model, allowing the program to handle multiple tasks at the same time, thereby improving the system's response speed and fault tolerance. In PHP programming, there are many ways to perform asynchronous programming, such as using multi-threading, coroutines, event-driven and other technologies. This article will focus on event-driven asynchronous programming in PHP and provide some usage examples and recommendations for open source tools. 1. Event-driven model in PHP PHP operation
