


PHP and WebDriver extension: How to handle reset and submit of web forms
PHP and WebDriver extension: How to handle the reset and submission of web forms
Introduction:
In web development, handling the reset and submission of web forms is a very important task. This article will introduce how to use PHP and WebDriver extensions to implement the form reset and submit functions required in automated testing. Sample code will help you better understand the implementation process.
1. Install and configure the WebDriver extension
First, you need to install and configure the PHP WebDriver extension. This can be downloaded and installed from the PHP official website. Once the installation is complete, you need to enable the extension in the php.ini file and restart your web server.
2. Form reset
In the web page, the form reset button is used to reset all input fields of the form to their initial state. To simulate this, we can use WebDriver's sendKeys() function to send an empty string to each field in the form.
Sample code:
// 创建WebDriver实例 $webdriver = new WebDriver(); // 打开要测试的网页 $webdriver->get('http://example.com/form'); // 获取表单 $form = $webdriver->findElement(WebDriverBy::tagName('form')); // 重置表单 $form->sendKeys(Keys::CONTROL, 'a'); // 选择表单中的所有字段 $form->sendKeys(Keys::NULL, Keys::DELETE); // 删除所有字段的值 // 提交表单 $form->submit();
3. Form submission
In a web page, the form submit button is used to send the data entered by the user in the form to the server for processing. To simulate this operation, we can use WebDriver's submit() function to submit the form.
Sample code:
// 创建WebDriver实例 $webdriver = new WebDriver(); // 打开要测试的网页 $webdriver->get('http://example.com/form'); // 获取表单 $form = $webdriver->findElement(WebDriverBy::tagName('form')); // 填写表单字段 $nameInput = $form->findElement(WebDriverBy::name('name')); $nameInput->sendKeys('John Doe'); $emailInput = $form->findElement(WebDriverBy::name('email')); $emailInput->sendKeys('johndoe@example.com'); // 提交表单 $form->submit();
Conclusion:
By using the PHP WebDriver extension, we can easily handle the reset and submission of web forms. Through the sample code, we can see how to use the sendKeys() function to reset the values of the form fields and the submit() function to submit the form. These techniques are very useful for automated testing and can greatly simplify the testing process.
In actual projects, you can customize and optimize these sample codes according to specific needs and page structure. I hope this article can help you better understand and apply the reset and submit functions of web forms in the PHP WebDriver extension.
The above is the detailed content of PHP and WebDriver extension: How to handle reset and submit of web forms. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


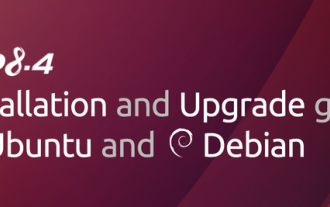
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
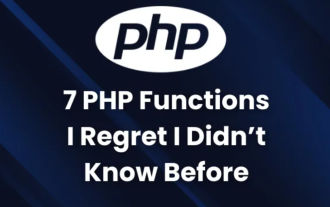
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
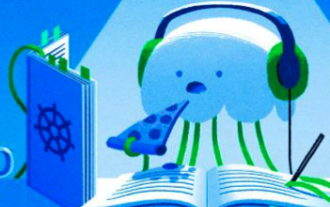
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
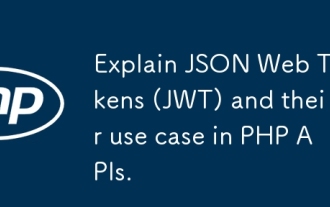
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
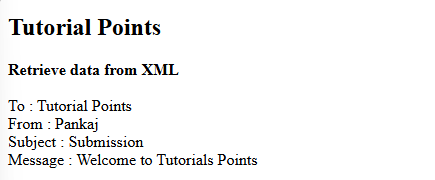
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
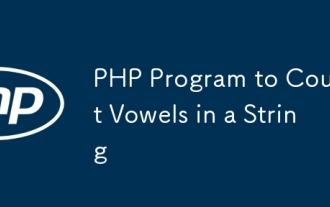
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
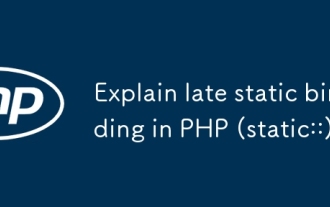
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
