How to write a greedy algorithm using PHP
How to use PHP to write a greedy algorithm
The greedy algorithm (Greedy algorithm) is a simple and effective algorithm used to solve a type of optimization problem. Its basic idea is to make the choice at each step that seems best at the moment, without regard to future consequences. This article will introduce how to write a greedy algorithm using PHP and provide relevant code examples.
1. Problem description
Before explaining the greedy algorithm, let’s first define a specific problem for a better understanding. Suppose there is a set of tasks, each task has a start time and end time. The goal is to select as many tasks as possible and make them not conflict with each other, i.e. their time periods do not overlap. The time of the task can be represented by an array, each element contains the start time and end time. We want to find the maximum number of tasks.
2. Algorithm idea
The greedy algorithm usually consists of three steps: selection phase, verification phase and update phase.
Selection phase: Select a task with the earliest end time from all tasks.
Verification phase: Remove the selected task from the task list and add it to the results list.
Update phase: Remove other tasks that conflict with the selected task.
Repeat the above steps until the task list is empty.
3. Code implementation
The following is a sample code for writing a greedy algorithm using PHP:
function greedyAlgorithm($tasks) { // 按结束时间对任务进行排序 usort($tasks, function($a, $b) { return $a['end'] - $b['end']; }); $result = []; // 结果列表 while (!empty($tasks)) { $task = array_shift($tasks); // 选择具有最早结束时间的任务 $result[] = $task; // 将任务添加到结果列表中 // 移除与所选任务冲突的其他任务 $tasks = array_filter($tasks, function($item) use ($task) { return $item['start'] >= $task['end']; }); } return $result; } // 测试 $tasks = [ ['start' => 1, 'end' => 3], ['start' => 2, 'end' => 4], ['start' => 3, 'end' => 6], ['start' => 5, 'end' => 7], ['start' => 6, 'end' => 8], ['start' => 8, 'end' => 10] ]; $result = greedyAlgorithm($tasks); print_r($result);
4. Algorithm analysis
The time complexity of the greedy algorithm Usually O(nlogn), where n is the number of tasks. Since the task list needs to be sorted, the time complexity of sorting is O(nlogn). Then, the task list is traversed, and the remaining tasks need to be filtered each time. The time complexity of filtering is O(n). Therefore, the time complexity of the entire algorithm is O(nlogn n), that is, O(nlogn).
5. Summary
The greedy algorithm is widely used in some optimization problems. Its simplicity and efficiency make it a commonly used algorithm. This article explains how to write a greedy algorithm using PHP and gives an example of a specific problem. I hope this article is helpful in understanding and using greedy algorithms.
The above is the detailed content of How to write a greedy algorithm using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


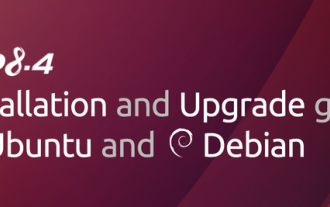
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
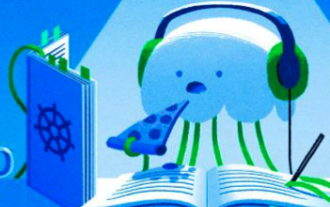
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
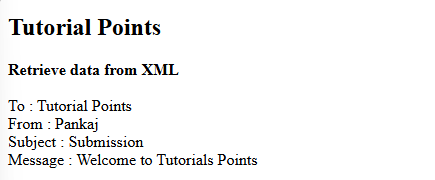
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
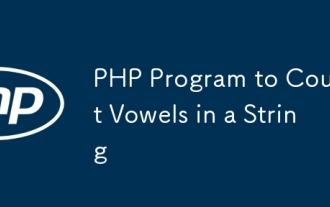
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
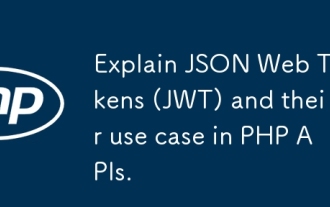
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
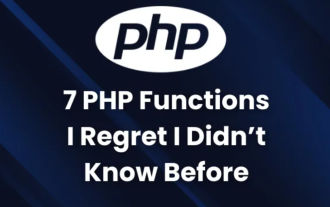
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
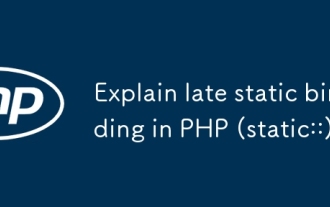
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
