


Use Python and WebDriver extensions to automatically process verification codes on web pages
Use Python and WebDriver extensions to automatically process verification codes on web pages
When we automate web page processing, verification codes are often a very thorny problem. Traditional verification code processing methods include manual input or the use of third-party verification code recognition services, but these methods are inconvenient. In this article, we will use Python and WebDriver technology to automatically process verification codes on web pages.
First, we need to install Python and WebDriver. Python is a popular scripting language with powerful text processing and networking capabilities. WebDriver is a tool for automated testing that controls browser behavior.
Next, we will use the selenium library in Python to operate WebDriver. First, we need to import the selenium library:
from selenium import webdriver
Then, we can choose our favorite browser to instantiate WebDriver. Here we take the Chrome browser as an example:
driver = webdriver.Chrome()
Next, we need to access a web page that requires a verification code and find the element of the verification code. We can use the element positioning method provided by WebDriver to find the verification code element.
captcha_element = driver.find_element_by_id("captcha")
Then, we can save the image of the verification code locally through the screenshot function. WebDriver provides a save_screenshot()
method to implement this function.
driver.save_screenshot("screenshot.png")
Next, we can use the third-party library PIL to process the image. We can open the screenshot image through PIL's Image module and crop it using the element coordinates of the verification code.
from PIL import Image screenshot = Image.open("screenshot.png") captcha_image = screenshot.crop((x, y, width + x, height + y))
Then, we can use PIL's image processing functions, such as converting the image to grayscale.
captcha_image = captcha_image.convert('L')
Now, we can use the third-party library tesseract to identify the verification code. tesseract is an open source OCR engine that can be used for image text recognition.
First, we need to install tesseract and configure it into environment variables. Then, in Python code, we can use the pytesseract library to call tesseract.
import pytesseract text = pytesseract.image_to_string(captcha_image)
Finally, we can fill in the recognized verification code into the corresponding input box on the web page. We can continue to use the element positioning method provided by WebDriver to find the input box, and use its send_keys()
method to fill in the verification code.
input_element = driver.find_element_by_id("captcha-input") input_element.send_keys(text)
So far, we have implemented the function of automatically processing verification codes on web pages using Python and WebDriver extensions. The complete code example is as follows:
from selenium import webdriver from PIL import Image import pytesseract # 实例化WebDriver driver = webdriver.Chrome() # 访问网页并找到验证码元素 captcha_element = driver.find_element_by_id("captcha") # 截屏保存验证码图像 driver.save_screenshot("screenshot.png") # 打开截屏的图像,并裁剪出验证码图像 screenshot = Image.open("screenshot.png") captcha_image = screenshot.crop((x, y, width + x, height + y)) # 图像处理,转换为灰度图 captcha_image = captcha_image.convert('L') # 使用tesseract识别验证码 text = pytesseract.image_to_string(captcha_image) # 填写验证码 input_element = driver.find_element_by_id("captcha-input") input_element.send_keys(text)
It should be noted that image recognition and verification code element positioning require certain debugging and testing. If the difficulty of the CAPTCHA is high, consider other approaches, such as using machine learning or deep learning models to identify the CAPTCHA.
To sum up, using Python and WebDriver extensions to automatically process verification codes on web pages is a very challenging task. However, through reasonable selection of methods and tools, we can effectively automate the processing of verification codes on web pages and improve the efficiency and accuracy of automated processing. I hope the content of this article will be helpful to everyone.
The above is the detailed content of Use Python and WebDriver extensions to automatically process verification codes on web pages. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


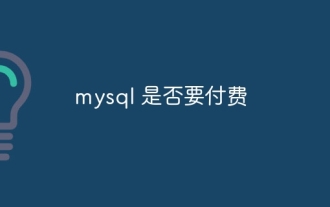
MySQL has a free community version and a paid enterprise version. The community version can be used and modified for free, but the support is limited and is suitable for applications with low stability requirements and strong technical capabilities. The Enterprise Edition provides comprehensive commercial support for applications that require a stable, reliable, high-performance database and willing to pay for support. Factors considered when choosing a version include application criticality, budgeting, and technical skills. There is no perfect option, only the most suitable option, and you need to choose carefully according to the specific situation.
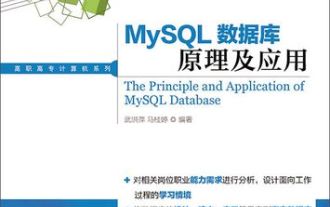
The article introduces the operation of MySQL database. First, you need to install a MySQL client, such as MySQLWorkbench or command line client. 1. Use the mysql-uroot-p command to connect to the server and log in with the root account password; 2. Use CREATEDATABASE to create a database, and USE select a database; 3. Use CREATETABLE to create a table, define fields and data types; 4. Use INSERTINTO to insert data, query data, update data by UPDATE, and delete data by DELETE. Only by mastering these steps, learning to deal with common problems and optimizing database performance can you use MySQL efficiently.
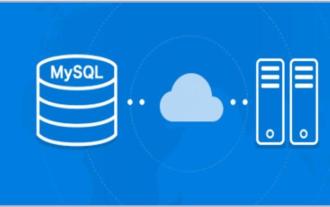
The main reasons for MySQL installation failure are: 1. Permission issues, you need to run as an administrator or use the sudo command; 2. Dependencies are missing, and you need to install relevant development packages; 3. Port conflicts, you need to close the program that occupies port 3306 or modify the configuration file; 4. The installation package is corrupt, you need to download and verify the integrity; 5. The environment variable is incorrectly configured, and the environment variables must be correctly configured according to the operating system. Solve these problems and carefully check each step to successfully install MySQL.
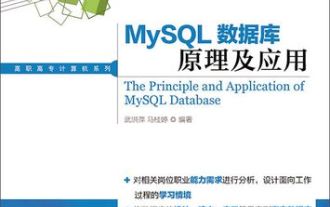
MySQL download file is corrupt, what should I do? Alas, if you download MySQL, you can encounter file corruption. It’s really not easy these days! This article will talk about how to solve this problem so that everyone can avoid detours. After reading it, you can not only repair the damaged MySQL installation package, but also have a deeper understanding of the download and installation process to avoid getting stuck in the future. Let’s first talk about why downloading files is damaged. There are many reasons for this. Network problems are the culprit. Interruption in the download process and instability in the network may lead to file corruption. There is also the problem with the download source itself. The server file itself is broken, and of course it is also broken when you download it. In addition, excessive "passionate" scanning of some antivirus software may also cause file corruption. Diagnostic problem: Determine if the file is really corrupt
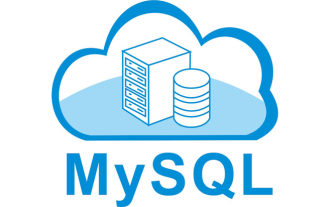
MySQL refused to start? Don’t panic, let’s check it out! Many friends found that the service could not be started after installing MySQL, and they were so anxious! Don’t worry, this article will take you to deal with it calmly and find out the mastermind behind it! After reading it, you can not only solve this problem, but also improve your understanding of MySQL services and your ideas for troubleshooting problems, and become a more powerful database administrator! The MySQL service failed to start, and there are many reasons, ranging from simple configuration errors to complex system problems. Let’s start with the most common aspects. Basic knowledge: A brief description of the service startup process MySQL service startup. Simply put, the operating system loads MySQL-related files and then starts the MySQL daemon. This involves configuration
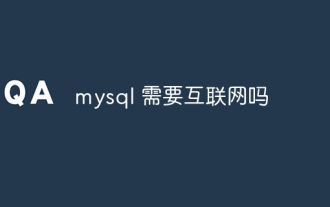
MySQL can run without network connections for basic data storage and management. However, network connection is required for interaction with other systems, remote access, or using advanced features such as replication and clustering. Additionally, security measures (such as firewalls), performance optimization (choose the right network connection), and data backup are critical to connecting to the Internet.
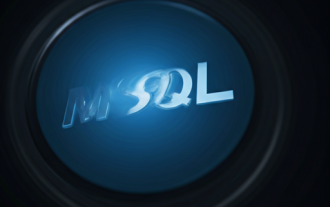
MySQL database performance optimization guide In resource-intensive applications, MySQL database plays a crucial role and is responsible for managing massive transactions. However, as the scale of application expands, database performance bottlenecks often become a constraint. This article will explore a series of effective MySQL performance optimization strategies to ensure that your application remains efficient and responsive under high loads. We will combine actual cases to explain in-depth key technologies such as indexing, query optimization, database design and caching. 1. Database architecture design and optimized database architecture is the cornerstone of MySQL performance optimization. Here are some core principles: Selecting the right data type and selecting the smallest data type that meets the needs can not only save storage space, but also improve data processing speed.
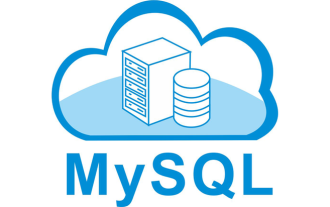
MySQL performance optimization needs to start from three aspects: installation configuration, indexing and query optimization, monitoring and tuning. 1. After installation, you need to adjust the my.cnf file according to the server configuration, such as the innodb_buffer_pool_size parameter, and close query_cache_size; 2. Create a suitable index to avoid excessive indexes, and optimize query statements, such as using the EXPLAIN command to analyze the execution plan; 3. Use MySQL's own monitoring tool (SHOWPROCESSLIST, SHOWSTATUS) to monitor the database health, and regularly back up and organize the database. Only by continuously optimizing these steps can the performance of MySQL database be improved.
