How to get product ratings and reviews using PHP Amazon API
How to use PHP Amazon API to obtain product ratings and comments
Amazon is one of the largest e-commerce platforms in the world. In order to help developers obtain evaluation and review data of Amazon products, Amazon provides a set of API interfaces . This article will introduce how to use PHP language to call Amazon API to obtain product ratings and reviews, and provide corresponding code examples.
- Apply for an Amazon developer account and API key
First, we need to register a developer account on the Amazon developer website and apply for an API key. After registration is completed and the API key is obtained, we can start using the Amazon API.
- Install Amazon AWS SDK for PHP
Amazon provides an official PHP SDK, which encapsulates various methods and functions for calling Amazon API for our convenience. We can install it through Composer or manually download and install it.
The following are the steps to install through Composer:
First, open the command line interface, switch to the project root directory, and execute the following command to install Composer:
curl -sS https://getcomposer.org/installer | php
Then, in Create a file named composer.json
in the project root directory and write the following content:
{ "require": { "aws/aws-sdk-php": "^3.0" } }
After saving the file, execute the following command to install the SDK:
php composer.phar install
Install After completion, the SDK will be downloaded to the vendor
directory.
- Calling Amazon API to get product ratings and reviews
It is very simple to call Amazon API using Amazon AWS SDK for PHP. Here is a sample code to get product ratings and reviews:
<?php require 'vendor/autoload.php'; use AwsCredentialsCredentials; use AwsSignatureSignatureV4; use AwsSdk; $accessKeyId = 'YOUR_ACCESS_KEY_ID'; $secretAccessKey = 'YOUR_SECRET_ACCESS_KEY'; $region = 'us-west-2'; // 根据实际情况选择区域 $credentials = new Credentials($accessKeyId, $secretAccessKey); $config = [ 'region' => $region, 'version' => 'latest', 'credentials' => $credentials, ]; $sdk = new Sdk($config); $service = $sdk->createAwsService([ 'service' => 'execute-api', ]); $request = $service->createRequest([ 'httpMethod' => 'GET', 'url' => 'https://api.amazon.com/your/api/endpoint', // 替换成实际的API地址 'headers' => [ 'Host' => 'api.amazon.com', ], 'endpoint' => 'https://api.amazon.com', ]); $signer = new SignatureV4('execute-api', $region); $request = $signer->signRequest($request, $credentials); $response = $service->executeRequest($request); $data = $response->toArray(); // 处理返回的数据 // ... ?>
Please replace YOUR_ACCESS_KEY_ID
and YOUR_SECRET_ACCESS_KEY
in the code with the actual API key.
https://api.amazon.com/your/api/endpoint
in the code indicates the specific address for calling the Amazon API, which should be modified according to actual needs.
- Processing the returned data
The data returned by the Amazon API is a JSON object containing product evaluation and review information. We can use PHP related functions to parse and process it. . According to actual needs, you can use the json_decode
function to convert JSON data into a PHP array, and then process and display it as required.
Summary
This article introduces how to use PHP language to call Amazon API to obtain product ratings and reviews. By applying for an Amazon developer account and API key, installing the Amazon AWS SDK for PHP, and then using the methods provided by the SDK to call the Amazon API, we can easily obtain product evaluation and review data. I hope this article will help you understand the use of Amazon API.
The above is the detailed content of How to get product ratings and reviews using PHP Amazon API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


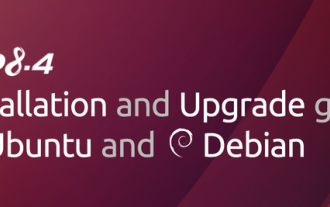
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
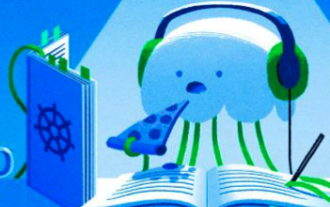
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
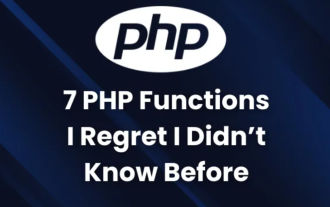
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
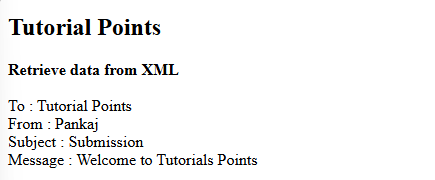
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
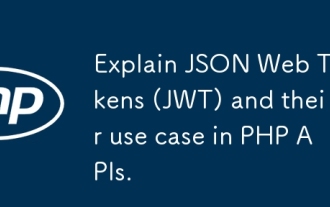
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
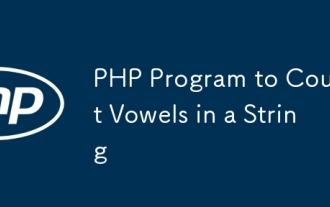
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
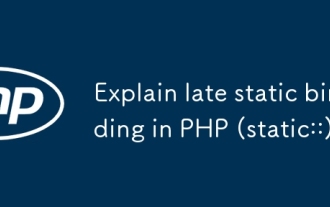
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
