


Implement automated testing framework using Python and WebDriver
Use Python and WebDriver to implement automated testing framework
With the rapid development of software development, automated testing has become an important means to ensure software quality and rapid delivery. Python, as a simple and easy-to-learn programming language, and WebDriver, as a powerful browser automation tool, can be combined well to achieve an efficient automated testing framework.
In this article, we will introduce how to use Python and WebDriver to build an automated testing framework, and provide code examples to help readers better understand.
Step 1: Install the necessary tools and libraries
First, we need to install Python and WebDriver. Python can be downloaded and installed from the official website (https://www.python.org), and WebDriver can find the corresponding driver from the official website of each browser or the open source community.
In addition, we need to install Python’s third-party library selenium. You can use the pip command to install it, as shown below:
pip install selenium
Step 2: Write test cases
Before building the automated testing framework, we need to write test cases first. A test case is a collection of test steps for a specific function or business logic, used to verify the correctness of the system.
The following is an example of a simple test case to verify the login function:
import unittest from selenium import webdriver class LoginTestCase(unittest.TestCase): def setUp(self): self.driver = webdriver.Chrome() self.driver.implicitly_wait(10) def test_login(self): # 打开登录页面 self.driver.get("http://www.example.com/login") # 输入用户名和密码 self.driver.find_element_by_id("username").send_keys("admin") self.driver.find_element_by_id("password").send_keys("123456") # 点击登录按钮 self.driver.find_element_by_id("login-button").click() # 验证登录成功后跳转到首页 self.assertEqual(self.driver.current_url, "http://www.example.com/home") def tearDown(self): self.driver.quit() if __name__ == "__main__": unittest.main()
Step 3: Build the test framework
Now that we have the test case, we can start Build an automated testing framework. The testing framework includes some common functions and classes to simplify and standardize the testing process.
The following is a simple test framework example, including a base class and a custom browser class:
class BaseTestCase(unittest.TestCase): def setUp(self): self.driver = None def tearDown(self): if self.driver: self.driver.quit() class Browser: def __init__(self, browser="chrome"): if browser == "chrome": self.driver = webdriver.Chrome() elif browser == "firefox": self.driver = webdriver.Firefox() else: raise ValueError("Unsupported browser: " + browser) def open(self, url): self.driver.get(url) def find_element(self, locator): return self.driver.find_element(*locator) def click(self, locator): self.find_element(locator).click() def input_text(self, locator, text): element = self.find_element(locator) element.clear() element.send_keys(text) def assert_url(self, expected_url): self.assertEqual(self.driver.current_url, expected_url) if __name__ == "__main__": unittest.main()
Step 4: Execute the test case
The last step is to execute the test case , and generate a test report. You can use the TestRunner provided by the unittest framework to run test cases and automatically generate test reports.
The following is a simple example of executing a test case:
import unittest from HTMLTestRunner import HTMLTestRunner if __name__ == "__main__": # 构建测试套件 suite = unittest.TestSuite() suite.addTest(LoginTestCase("test_login")) # 运行测试套件,并生成测试报告 with open("test_report.html", "wb") as f: runner = HTMLTestRunner(stream=f, title="Test Report", description="Test Results") runner.run(suite)
Summary
This article introduces the basic steps of how to use Python and WebDriver to implement an automated testing framework, and provides the corresponding code Example. The combination of Python and WebDriver provides a powerful and flexible tool for automated testing, allowing us to verify the correctness and stability of the software more efficiently.
The above is the detailed content of Implement automated testing framework using Python and WebDriver. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


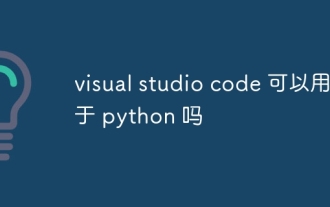
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
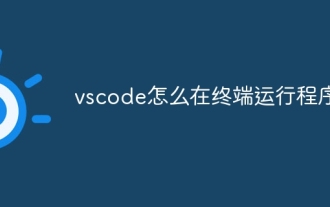
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
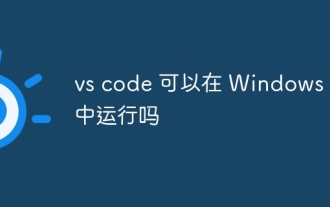
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
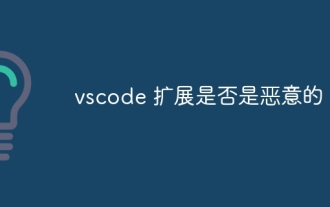
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
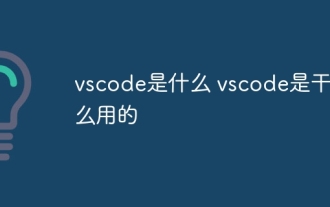
VS Code is the full name Visual Studio Code, which is a free and open source cross-platform code editor and development environment developed by Microsoft. It supports a wide range of programming languages and provides syntax highlighting, code automatic completion, code snippets and smart prompts to improve development efficiency. Through a rich extension ecosystem, users can add extensions to specific needs and languages, such as debuggers, code formatting tools, and Git integrations. VS Code also includes an intuitive debugger that helps quickly find and resolve bugs in your code.
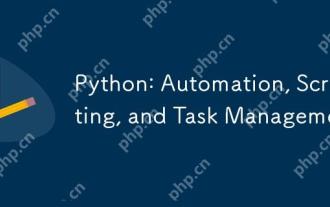
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
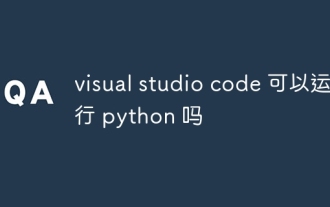
VS Code not only can run Python, but also provides powerful functions, including: automatically identifying Python files after installing Python extensions, providing functions such as code completion, syntax highlighting, and debugging. Relying on the installed Python environment, extensions act as bridge connection editing and Python environment. The debugging functions include setting breakpoints, step-by-step debugging, viewing variable values, and improving debugging efficiency. The integrated terminal supports running complex commands such as unit testing and package management. Supports extended configuration and enhances features such as code formatting, analysis and version control.
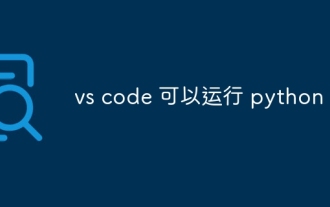
Yes, VS Code can run Python code. To run Python efficiently in VS Code, complete the following steps: Install the Python interpreter and configure environment variables. Install the Python extension in VS Code. Run Python code in VS Code's terminal via the command line. Use VS Code's debugging capabilities and code formatting to improve development efficiency. Adopt good programming habits and use performance analysis tools to optimize code performance.
