New GRPC extension introduced in PHP8.1
New GRPC extension introduced in PHP8.1
PHP is a widely used server-side scripting language that can dynamically generate web page content by interacting with the web server. In order to better meet the needs of modern service architecture, PHP8.1 version introduces a new GRPC extension, which further improves the performance and scalability of PHP. This article will introduce the basic concepts of GRPC extension and give some code examples to help readers better understand and apply this new feature.
1. Introduction to GRPC
GRPC is a high-performance, general-purpose open source RPC (remote procedure call) framework. RPC is a computer communication protocol that allows a program to directly call functions or methods on another computer without knowing the underlying network details. GRPC is based on Google's open source HTTP/2 protocol implementation and uses a binary transmission protocol with the following characteristics:
- Efficient serialization and deserialization: GRPC uses Protocol Buffers as the default serialization mechanism. More efficient than text formats such as JSON and XML.
- Reliable streaming: GRPC supports bidirectional streaming, enabling multiple independent requests and responses to be made simultaneously on a single network connection.
- Multi-language support: GRPC supports multiple programming languages, including C, Java, Go, Python, etc.
- Automation tools: GRPC provides a wealth of code generation tools that can generate client-side and server-side code for different programming languages.
2. Install the GRPC extension
Before starting to use GRPC, we first need to install the GRPC extension. In PHP8.1, support for the GRPC extension is provided by default. We can install it through the following steps:
- Install the PHP8.1 version
-
Install the GRPC extension: use PECL The command to install the GRPC extension module is as follows:
1
pecl install grpc
Copy after login Enable the GRPC extension in the php.ini file: Add the following configuration to the php.ini file:
1
extension=grpc
Copy after login
3. Use the GRPC extension
After the installation is completed, we can use the GRPC extension to make remote procedure calls. Below we give a simple example to illustrate how to use the GRPC extension.
Define the interface
First, we need to define an interface file (.proto file) to describe the data structure and methods of the interface. For example, we define a Greeter interface, including a SayHello method:1
2
3
4
5
6
7
8
9
10
11
12
13
syntax =
"proto3"
;
service Greeter {
rpc SayHello (HelloRequest) returns (HelloResponse) {}
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
Copy after loginGenerate code
Next, we use the code generation tool provided by GRPC to generate the corresponding code based on the .proto file Client and server side code. First, we need to install the Protocol Buffers tool, and then execute the following command to generate code:1
protoc --php_out=./out --grpc_out=./out --plugin=protoc-gen-grpc=/usr/local/bin/grpc_php_plugin greeter.proto
Copy after loginAfter executing the above command, an out directory will be generated containing the generated PHP code file.
Implementing the server side
Next, we can implement the server side code. In the code, we need to implement the methods defined by the interface. For example:1
2
3
4
5
6
7
8
9
class
GreeterImpl
extends
GreeterGreeterService
{
public
function
SayHello(GreeterHelloRequest
$request
): GreeterHelloResponse
{
$response
=
new
GreeterHelloResponse();
$response
->setMessage(
"Hello, "
.
$request
->getName());
return
$response
;
}
}
Copy after loginStart the server
We can start a GRPC server through the following code and listen to the specified port:1
2
3
4
5
6
7
8
9
10
11
12
13
$server
=
new
SwooleServer(
'0.0.0.0'
, 50051, SWOOLE_PROCESS, SWOOLE_SOCK_TCP | SWOOLE_SSL);
$server
->set([
'ssl_cert_file'
=>
'server.crt'
,
'ssl_key_file'
=>
'server.key'
,
]);
$server
->on(
"start"
,
function
(SwooleServer
$server
) {
echo
"GRPC server is started at 0.0.0.0:50051
";
});
$server
->on(
"receive"
,
function
(SwooleServer
$server
,
$fd
,
$fromId
,
$data
) {
$server
->send(
$fd
,
$data
);
});
$server
->start();
Copy after loginClient Call
Finally, we can write a client code to call methods on the remote server over the network. For example:1
2
3
4
5
$client
=
new
GreeterGreeterClient(
'127.0.0.1:50051'
);
$request
=
new
GreeterHelloRequest();
$request
->setName(
"PHP"
);
$response
=
$client
->SayHello(
$request
);
echo
$response
->getMessage();
Copy after login
Through the above code example, we can learn how to use the GRPC extension to make remote procedure calls in PHP8.1. GRPC extensions provide a more efficient and reliable remote communication method, helping to build modern distributed systems. In actual projects, we can flexibly use GRPC extensions to build a cross-language microservice architecture based on business needs.
Summary
GRPC extension is an important feature introduced in PHP8.1 version, which enables PHP to have more efficient and scalable RPC capabilities. Through code examples, we have understood the basic concepts and usage of GRPC extensions. We hope that readers can further learn and apply this new feature and improve their PHP development capabilities.
The above is the detailed content of New GRPC extension introduced in PHP8.1. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




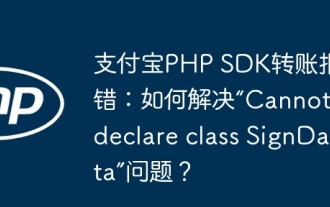
Alipay PHP...
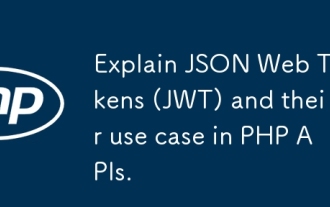
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
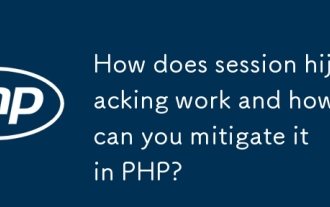
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
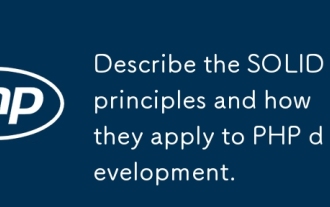
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
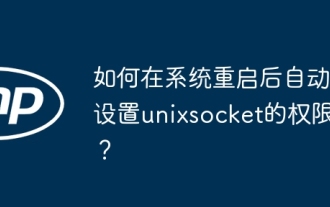
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
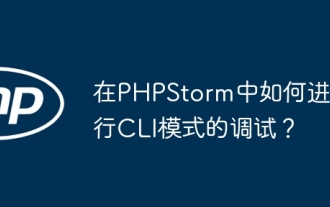
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
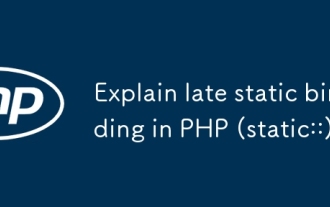
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
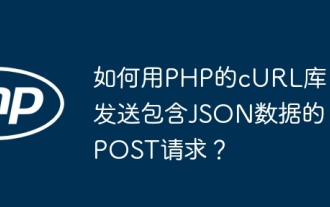
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
