


Use PHP and WebDriver extensions to truncate and intercept web content
Use PHP and WebDriver extensions to truncate and intercept web page content
In modern web development, we often encounter the need to truncate and intercept web page content. This article will introduce how to use PHP and WebDriver extensions to achieve this functionality.
First, we need to install and configure the PHP and WebDriver extensions. WebDriver is a tool for automated testing that can simulate user operations in the browser. In this article, we will use WebDriver to load web pages and obtain web content.
The process of installing and configuring PHP and WebDriver extensions is beyond the scope of this article. Readers can find relevant documents by themselves.
Next, we need to write PHP code to truncate and intercept web page content. The following is a sample code:
<?php use FacebookWebDriverRemoteDesiredCapabilities; use FacebookWebDriverRemoteRemoteWebDriver; use FacebookWebDriverWebDriverBy; // 设置WebDriver服务器的URL和浏览器类型 $driver = RemoteWebDriver::create('http://localhost:4444/wd/hub', DesiredCapabilities::firefox()); // 加载网页 $driver->get('http://example.com'); // 获取网页内容 $content = $driver->getPageSource(); // 截断网页内容 $max_length = 100; if (strlen($content) > $max_length) { $content = substr($content, 0, $max_length) . '...'; } // 输出截断后的网页内容 echo $content; // 关闭WebDriver $driver->quit(); ?>
The above code first creates a WebDriver instance, then uses the get()
method to load a web page, and uses the getPageSource()
The method obtains the content of the web page. Next, use the substr()
function to truncate the web page content. The truncated length is specified by the $max_length
variable. Finally, the truncated web page content is output to the browser, and the WebDriver is closed using the quit()
method.
Through the above code, we can easily realize the truncation and interception of web page content. This is very useful in some scenarios where you need to display a summary of the web page or limit the content length. Readers can modify and extend the above code according to their own needs.
It should be noted that the above example code uses the Firefox browser as the running environment of WebDriver. If you need to use other browsers, you can set the corresponding browser type in DesiredCapabilities
.
In addition to truncating web page content, we can also use the WebDriver extension to intercept specific parts of the web page. For example, we can use the findElement()
method and XPath or CSS selector to locate an element in the web page and then obtain its content. The following is a sample code:
<?php // ... // 定位到网页中的标题元素并获取其内容 $title_element = $driver->findElement(WebDriverBy::xpath("//h1")); $title = $title_element->getText(); // 输出标题内容 echo $title; // ... ?>
The above code uses the XPath selector to locate the h1 tag in the web page, and uses the getText()
method to obtain its content. Output the obtained title content to the browser.
In summary, using PHP and WebDriver extensions can easily achieve truncation and interception of web page content. By loading the web page, obtaining the web page content and processing it using string functions, we can flexibly operate on the web page content. I hope the content of this article will be helpful to readers.
The above is the detailed content of Use PHP and WebDriver extensions to truncate and intercept web content. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


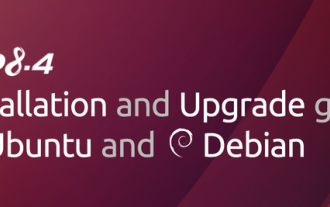
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
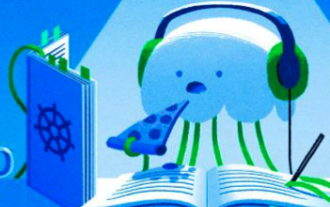
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
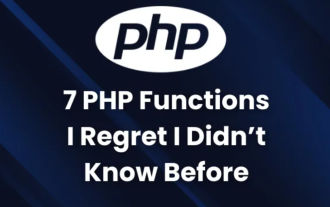
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
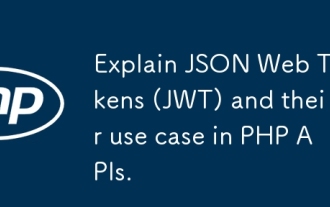
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
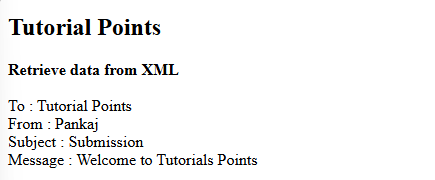
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
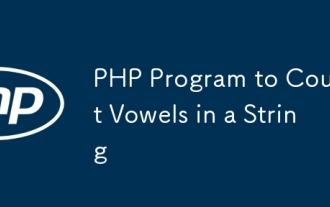
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
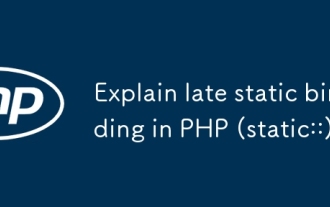
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
