


How to implement instant search and auto-completion functions through the Webman framework?
How to implement instant search and auto-completion functions through the Webman framework?
With the rapid development of the Internet, our requirements for the user experience of web pages are getting higher and higher. One of the important requirements is instant search and auto-complete functions. When the user enters keywords in the input box, the page can quickly provide relevant search results based on the keywords or automatically prompt the user for possible inputs. In this article, we will introduce how to use the Webman framework to achieve these two functions.
First of all, we need to introduce the Webman framework into the project. This can be achieved by adding the following dependencies in the project's pom.xml file:
<dependency> <groupId>com.github.yuedeng</groupId> <artifactId>webman-spring-boot-starter</artifactId> <version>0.5.2</version> </dependency>
Next, we need to configure some parameters of the Webman framework in the Spring Boot configuration file. You can add the following configuration in the application.properties file:
# 配置Webman框架的数据源 webman.datasource.driver-class-name=com.mysql.cj.jdbc.Driver webman.datasource.url=jdbc:mysql://localhost:3306/database_name?useUnicode=true&characterEncoding=utf-8&serverTimezone=Asia/Shanghai webman.datasource.username=root webman.datasource.password=root # 配置Webman框架的Redis缓存 webman.cache.type=redis webman.cache.redis.host=localhost webman.cache.redis.port=6379 webman.cache.redis.password= webman.cache.redis.database=0
In the above configuration, we need to configure the database and Redis cache used by the Webman framework. The database is used to store search result data, and Redis is used to store cached data for the auto-complete function.
Next, we need to create a search service class to handle the logic of user input and search results. You can create a class named SearchService and add the following code to the class:
@Service public class SearchService { @Autowired private WebmanTemplate webmanTemplate; public List<String> search(String keyword) { SearchQuery query = new SearchQuery("your_database_table_name"); query.addFilter("content", Operator.LIKE, keyword); query.setLimit(10); SearchResponse response = webmanTemplate.search(query); List<String> results = new ArrayList<>(); for (SearchHit hit : response.getHits()) { results.add(hit.getSource().get("content").toString()); } return results; } public List<String> autoComplete(String keyword) { AutoCompleteQuery query = new AutoCompleteQuery("your_redis_key_prefix", keyword); query.setLimit(10); AutoCompleteResponse response = webmanTemplate.autoComplete(query); List<String> results = new ArrayList<>(); for (AutoCompleteHit hit : response.getHits()) { results.add(hit.getValue()); } return results; } }
In the above code, we have injected a WebmanTemplate instance, which is the core of interacting with data sources and caches provided by the Webman framework kind. In the search method, we use SearchQuery to construct a search query, then use webmanTemplate to perform the query operation, and convert the search results into a List for return. In the autoComplete method, we use AutoCompleteQuery to build an auto-complete query, and then also use webmanTemplate to perform the query operation, and convert the results of the auto-prompt into a List for return.
Finally, we need to handle the user's request in the controller. You can create a controller class named SearchController and add the following code to the class:
@RestController public class SearchController { @Autowired private SearchService searchService; @GetMapping("/search") public List<String> search(@RequestParam("keyword") String keyword) { return searchService.search(keyword); } @GetMapping("/autocomplete") public List<String> autoComplete(@RequestParam("keyword") String keyword) { return searchService.autoComplete(keyword); } }
In the above code, we injected the SearchService instance and defined two interfaces for processing search requests. and autocomplete requests. By passing the keyword parameter in the request, the controller will call the corresponding SearchService method and return the search results or automatically prompted results.
So far, we have completed all the steps to use the Webman framework to implement instant search and auto-complete functions. Next, we can launch the application and test our functionality by accessing the following URL:
- Search interface: http://localhost:8080/search?keyword=Keyword
- Autocomplete interface: http://localhost:8080/autocomplete?keyword=Keyword
In the test, we can see that according to the entered keywords, the page will quickly display the corresponding search results or automatically prompted results.
Through the introduction of this article, we have learned how to use the Webman framework to implement instant search and automatic completion functions. Through the application of these functions, we can improve the user experience of web pages and allow users to find the information they need more easily. At the same time, this is also an application example of the Webman framework, I hope it will be helpful to readers.
The above is the detailed content of How to implement instant search and auto-completion functions through the Webman framework?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


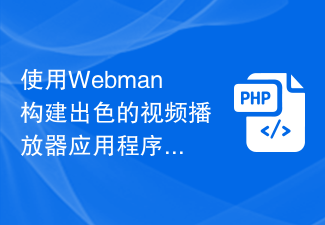
Build an excellent video player application using Webman With the rapid development of the Internet and mobile devices, video playback has become an increasingly important part of people's daily lives. Building a powerful, stable and efficient video player application is the pursuit of many developers. This article will introduce how to use Webman to build an excellent video player application, and attach corresponding code examples to help readers get started quickly. Webman is a lightweight web based on JavaScript and HTML5 technology
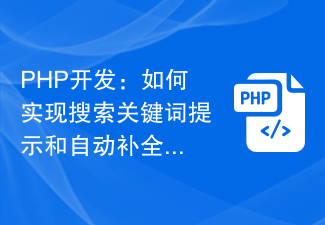
PHP development: Implementing search keyword prompts and automatic completion functions In today's Internet era, search engines have become one of the important channels for people to obtain information. In website development, the importance of search function is self-evident. In order to improve user experience and search results, implementing search keyword prompts and automatic completion functions is a very valuable and necessary development task. This article will introduce how to implement search keyword prompts and automatic completion functions in PHP development, and provide specific code examples. Search keyword prompts refer to the
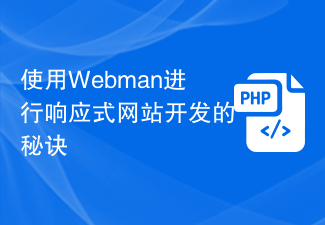
Tips for Responsive Website Development with Webman In today’s digital age, people are increasingly relying on mobile devices to access the Internet. In order to provide a better user experience and adapt to different screen sizes, responsive website development has become an important trend. As a powerful framework, Webman provides us with many tools and technologies to realize the development of responsive websites. In this article, we will share some tips for using Webman for responsive website development, including how to set up media queries,
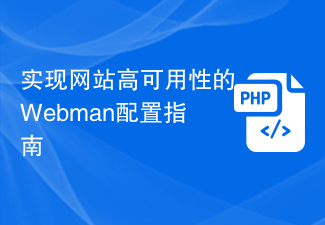
Introduction to Webman Configuration Guide for Implementing High Availability of Websites: In today's digital era, websites have become one of the important business channels for enterprises. In order to ensure the business continuity and user experience of enterprises and ensure that the website is always available, high availability has become a core requirement. Webman is a powerful web server management tool that provides a series of configuration options and functions that can help us achieve a high-availability website architecture. This article will introduce some Webman configuration guides and code examples to help you achieve the high performance of your website.
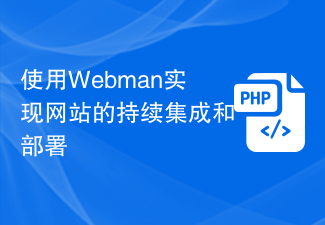
Using Webman to achieve continuous integration and deployment of websites With the rapid development of the Internet, the work of website development and maintenance has become more and more complex. In order to improve development efficiency and ensure website quality, continuous integration and deployment have become an important choice. In this article, I will introduce how to use the Webman tool to implement continuous integration and deployment of the website, and attach some code examples. 1. What is Webman? Webman is a Java-based open source continuous integration and deployment tool that provides

Optimize the maintainability and scalability of the website through Webman Introduction: In today's digital age, the website, as an important way of information dissemination and communication, has become an indispensable part of enterprises, organizations and individuals. With the continuous development of Internet technology, in order to cope with increasingly complex needs and changing market environments, we need to optimize the website and improve its maintainability and scalability. This article will introduce how to optimize the maintainability and scalability of the website through the Webman tool, and attach code examples. 1. What is
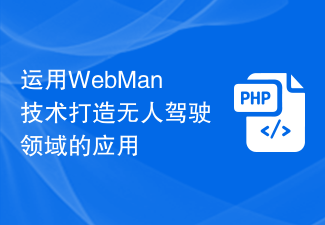
Using WebMan technology to create applications in the field of driverless driving With the continuous advancement of technology and the rapid development of artificial intelligence, driverless vehicles have gradually become a hot topic in the automotive industry. WebMan is a technology used to develop Web applications. It can be applied in the field of driverless driving to realize functions such as vehicle remote control, data monitoring, and vehicle information management. This article will introduce how to use WebMan technology to build applications in the field of autonomous driving, and illustrate its implementation process through code examples. 1. Environment preparation before using W

Webman: The best choice for building a modern corporate website. With the rapid development of the Internet and companies' emphasis on online image, modern corporate websites have become an important channel for companies to carry out brand promotion, product introduction and communication. However, building a powerful and easy-to-maintain corporate website is not an easy task. Before finding the best choice, we first need to clarify the needs and goals of the corporate website. Corporate websites usually need to have the following elements: Page design: attractive design style, clear navigation and layout, adaptable design
